如何创建二维数组,并使用libtorch将其转化为tensor
时间: 2023-06-04 14:07:33 浏览: 585
您好,关于二维数组的创建,可以使用C++提供的标准库中的vector容器来实现,代码如下:
```c++
#include <vector>
using namespace std;
int main() {
// 创建一个二维数组
vector<vector<float>> arr(3, vector<float>(4, 0.0));
// 将二维数组转化为Tensor
Tensor tensor = torch::from_blob(arr.data(), {3, 4});
return 0;
}
```
以上代码使用了vector容器来实现二维数组的创建,同时使用了libtorch中的from_blob函数将二维数组转换为Tensor。需要注意的是,上面的代码假设创建的二维数组是float类型的,如果是其他类型的数组,需要将上述代码中的float替换为其它类型的名称。另外,在转化为Tensor时,需要提供数组的shape信息。
相关问题
将数组转化为tensor
在Python中,可以使用NumPy库将数组转换为Tensor。示例如下:
```python
import numpy as np
import torch
# 定义一个数组
arr = np.array([1, 2, 3, 4, 5])
# 将数组转换为Tensor
tensor = torch.from_numpy(arr)
print(tensor)
```
输出结果为:
```
tensor([1, 2, 3, 4, 5])
```
注意:在将NumPy数组转换为Tensor时,Tensor会共享数据存储空间,因此对Tensor的修改会反映在原始NumPy数组上。
数组转化为tensor
在PyTorch和TensorFlow中,可以通过torch.tensor()和tf.convert_to_tensor()方法将数组转换为Tensor对象。以下是一个示例:
在PyTorch中:
```python
import torch
import numpy as np
# 创建一个2x3的数组
arr = np.array([[1, 2, 3], [4, 5, 6]])
# 将数组转换为Tensor对象
t = torch.tensor(arr)
# 打印数组和Tensor对象
print("Array:", arr)
print("Tensor:", t)
```
输出:
```
Array: [[1 2 3]
[4 5 6]]
Tensor: tensor([[1, 2, 3],
[4, 5, 6]])
```
在TensorFlow中:
```python
import tensorflow as tf
import numpy as np
# 创建一个2x3的数组
arr = np.array([[1, 2, 3], [4, 5, 6]])
# 将数组转换为Tensor对象
t = tf.convert_to_tensor(arr)
# 打印数组和Tensor对象
print("Array:", arr)
print("Tensor:", t)
```
输出:
```
Array: [[1 2 3]
[4 5 6]]
Tensor: tf.Tensor(
[[1 2 3]
[4 5 6]], shape=(2, 3), dtype=int64)
```
可以看到,两种方法都可以将数组转换为Tensor对象。
阅读全文
相关推荐
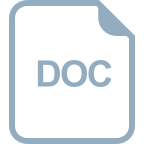
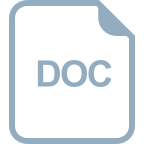
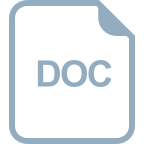
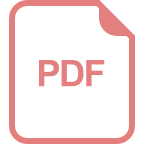












