springboot mabatis sql留言板
时间: 2023-09-20 10:06:18 浏览: 164
### 回答1:
好的,你想了解关于 Spring Boot 和 MyBatis 结合实现 SQL 留言板的内容是吗?下面是一个简单的示例:
首先,你需要在 pom.xml 文件中添加 Spring Boot 和 MyBatis 的依赖:
```xml
<dependencies>
<!-- Spring Boot -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- MyBatis -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
<!-- MySQL 驱动 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.26</version>
</dependency>
</dependencies>
```
接下来,你需要创建一个 MyBatis 的 Mapper 接口和对应的 XML 文件来实现 SQL 操作。下面是一个简单的示例:
```java
@Mapper
public interface MessageMapper {
@Select("SELECT * FROM messages")
List<Message> getAllMessages();
@Insert("INSERT INTO messages (name, content) VALUES (#{name}, #{content})")
void addMessage(Message message);
}
```
```xml
<mapper namespace="com.example.mapper.MessageMapper">
<resultMap id="BaseResultMap" type="com.example.entity.Message">
<id column="id" property="id" jdbcType="BIGINT"/>
<result column="name" property="name" jdbcType="VARCHAR"/>
<result column="content" property="content" jdbcType="VARCHAR"/>
<result column="created_at" property="createdAt" jdbcType="TIMESTAMP"/>
</resultMap>
<select id="getAllMessages" resultMap="BaseResultMap">
SELECT * FROM messages
</select>
<insert id="addMessage">
INSERT INTO messages (name, content) VALUES (#{name}, #{content})
</insert>
</mapper>
```
接着,你需要创建一个 Service 层来调用 Mapper 接口:
```java
@Service
public class MessageService {
@Autowired
private MessageMapper messageMapper;
public List<Message> getAllMessages() {
return messageMapper.getAllMessages();
}
public void addMessage(Message message) {
messageMapper.addMessage(message);
}
}
```
最后,你需要创建一个 Controller 层来处理 HTTP 请求:
```java
@RestController
public class MessageController {
@Autowired
private MessageService messageService;
@GetMapping("/messages")
public List<Message> getAllMessages() {
return messageService.getAllMessages();
}
@PostMapping("/messages")
public void addMessage(@RequestBody Message message) {
messageService.addMessage(message);
}
}
```
这样,你就可以通过访问 `/messages` 接口来获取留言列表,通过访问 `/messages` 接口并传入留言信息来添加新的留言了。当然,你需要先创建一个 Message 实体类来对应数据库中的 messages 表:
```java
public class Message {
private Long id;
private String name;
private String content;
private Date createdAt;
// getters and setters
}
```
注意,在 Spring Boot 中,你需要在启动类上添加注解 `@MapperScan("com.example.mapper")` 来扫描 Mapper 接口所在的包。另外,你需要在 application.properties 或 application.yml 文件中配置数据库连接信息。
### 回答2:
Spring Boot是一种用于构建独立的、可执行的Spring应用程序的框架。MyBatis是一种持久层框架,用于将Java对象与SQL语句进行映射。SQL留言板是一个使用Spring Boot和MyBatis实现的具有基本的增删改查功能的留言板应用程序。
在使用Spring Boot和MyBatis开发SQL留言板时,可以先创建一个消息实体类,用于表示留言的相关信息,例如留言内容和创建时间等。然后,创建一个Mapper接口,用于定义数据库操作的方法,例如新增留言、删除留言和查询留言等。
接下来,可以通过注解方式配置MyBatis的数据库连接和映射关系。在应用启动时,Spring Boot会自动加载MyBatis的配置,并创建对应的数据库连接和SessionFactory。
在控制器(Controller)中,可以调用Mapper中的方法来实现留言板的各种功能。例如,可以编写一个用于新增留言的控制器方法,接收用户提交的留言信息,然后调用Mapper中的新增留言方法将留言信息插入数据库中。
此外,在视图层(View)中,可以使用Thymeleaf等模板引擎来展示和渲染留言数据。可以设计一个留言列表页面,展示所有留言的内容,并提供留言删除和分页功能。
总而言之,使用Spring Boot和MyBatis来开发SQL留言板,可以快速进行开发和部署,同时通过MyBatis的映射功能,可以方便地进行数据库操作,简化开发流程。这种结合使用的方式,可以帮助开发者更轻松地实现一个功能完善的留言板应用程序。
### 回答3:
Spring Boot是一个开源的Java开发框架,它简化了Java应用的开发过程。MyBatis是一个持久层框架,提供了对数据库的访问和操作的支持。SQL留言板是一个基于Spring Boot和MyBatis的实现的留言板应用。
在这个应用中,我们可以使用Spring Boot创建一个简单的web应用程序。我们可以通过创建一个Controller类来处理用户的请求,并定义相应的路由和处理逻辑。对于留言板应用,我们可以创建一个留言板Controller类,用于处理用户发表留言、查看留言和删除留言等操作。
在MyBatis中,我们可以定义留言板的数据模型,并创建对应的Mapper接口和对应的SQL语句。通过MyBatis的配置,我们可以连接数据库,并执行SQL语句进行数据的存储和读取操作。对于留言板应用,我们可以创建一个Message类来表示留言的数据模型,并创建对应的Mapper接口和SQL语句。
通过Spring Boot和MyBatis的结合,我们可以实现留言板应用的完整功能。用户可以通过访问网页来发表留言,系统会将留言存储到数据库中。用户可以查看所有留言,并选择删除自己的留言。应用还可以提供一些其他功能,如分页显示留言、按时间排序等。
总之,使用Spring Boot和MyBatis可以轻松地实现一个简单的SQL留言板应用。通过Spring Boot的简化开发和MyBatis的数据库访问支持,开发者可以专注于业务逻辑的实现,而无需过多关注底层的技术细节。
阅读全文
相关推荐
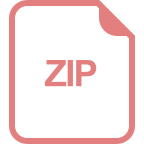
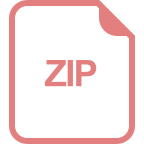
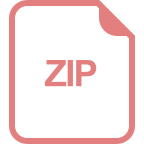
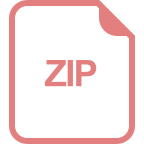
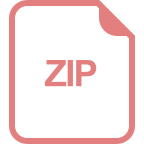
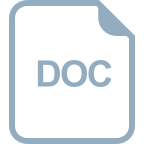
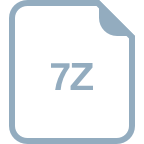
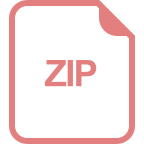
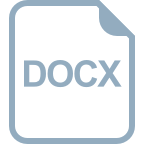
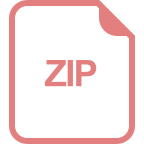
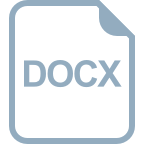
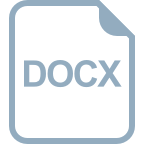
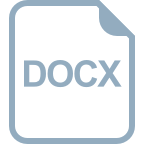
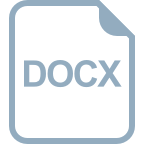
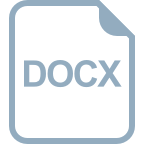
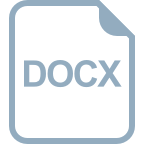
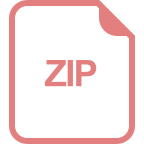
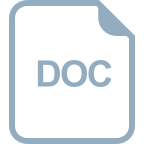
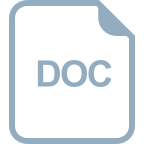