题目四 磁盘调度算法的模拟实现及对比
时间: 2023-12-18 09:04:28 浏览: 195
好的,我会尽力回答您的问题。磁盘调度算法是操作系统中的一个重要部分,可以有效地提高磁盘的性能和利用率。常见的磁盘调度算法有以下几种:
1. 先来先服务(FCFS)算法:按照磁盘请求的顺序依次进行磁盘读写操作,没有考虑磁盘寻道时间和旋转延迟等因素。
2. 最短寻道时间优先(SSTF)算法:选择离当前磁头位置最近的磁道进行读写操作,可以减少磁盘寻道时间。
3. 扫描(SCAN)算法:磁头按照一个方向移动,直到到达磁盘的边界,然后反向移动,直到到达另一边界,循环进行操作。
4. 循环扫描(C-SCAN)算法:类似于SCAN算法,但是在到达磁盘边界时,直接返回到另一端的起始位置,不进行反向移动。
5. N步扫描(N-Step-SCAN)算法:先按照SCAN算法进行一定步数的移动,然后再反向移动,循环进行操作。
这些算法的实现可以使用模拟的方式进行。我们可以使用Python等编程语言来编写程序,模拟磁盘调度算法的执行过程,并通过实验比较它们的性能差异。具体实现过程中,需要考虑到磁盘读写的时间、磁盘寻道时间、磁头移动的距离等因素,以便更准确地模拟实际情况。
通过实验比较,我们可以发现不同的磁盘调度算法在不同的应用场景下会有不同的性能表现。因此,我们需要根据具体的应用场景选择合适的算法,以达到最优的性能和效果。
相关问题
题目二 磁盘调度算法的模拟实现及对比
好的,你的问题是磁盘调度算法的模拟实现及对比。磁盘调度算法是操作系统中的重要组成部分,用于优化磁盘访问的效率。常见的磁盘调度算法有FCFS、SSTF、SCAN、C-SCAN、LOOK和C-LOOK等。下面我将对这些算法进行简单介绍,并实现一个模拟程序进行对比。
1. FCFS(先来先服务):按照磁盘访问的请求顺序进行调度,不考虑请求的距离和方向,可能导致产生大量寻道时间。
2. SSTF(最短寻道时间优先):选择距离当前磁头最近的请求进行调度,可能会产生饥饿现象,即某些请求一直得不到满足。
3. SCAN(扫描算法):磁头按照一定方向移动,直到到达最边缘时改变方向,只考虑当前方向上的请求,可能会产生逆向请求等待的现象。
4. C-SCAN(循环扫描算法):与SCAN类似,但在到达最边缘时不改变方向,而是回到最开始的位置重新扫描。
5. LOOK(往返扫描算法):与SCAN类似,但在到达最边缘时不改变方向,而是直接返回到另一端。
6. C-LOOK(循环往返扫描算法):与LOOK类似,但在到达最边缘时不改变方向,而是回到最开始的位置重新扫描。
下面是一个磁盘调度算法的模拟程序示例:
```python
import queue
def fcfs(queue, start):
distance = 0
current = start
while not queue.empty():
next = queue.get()
distance += abs(next - current)
current = next
return distance
def sstf(queue, start):
distance = 0
current = start
while not queue.empty():
min_distance = float('inf')
min_index = -1
for i in range(queue.qsize()):
next = queue.get()
temp_distance = abs(next - current)
if temp_distance < min_distance:
min_distance = temp_distance
min_index = next
queue.put(next)
distance += min_distance
current = min_index
queue.get(current)
return distance
def scan(queue, start):
direction = 1
distance = 0
current = start
while not queue.empty():
min_distance = float('inf')
min_index = -1
for i in range(queue.qsize()):
next = queue.get()
temp_distance = abs(next - current)
if temp_distance < min_distance and (next - current) * direction >= 0:
min_distance = temp_distance
min_index = next
queue.put(next)
if min_index == -1:
direction = -direction
continue
distance += min_distance
current = min_index
queue.get(current)
return distance
def cscan(queue, start):
direction = 1
distance = 0
current = start
while not queue.empty():
min_distance = float('inf')
min_index = -1
for i in range(queue.qsize()):
next = queue.get()
temp_distance = abs(next - current)
if temp_distance < min_distance and (next - current) * direction >= 0:
min_distance = temp_distance
min_index = next
queue.put(next)
if min_index == -1:
distance += abs(current - 0)
current = 0
direction = 1
continue
distance += min_distance
current = min_index
queue.get(current)
return distance
def look(queue, start):
direction = 1
distance = 0
current = start
while not queue.empty():
min_distance = float('inf')
min_index = -1
for i in range(queue.qsize()):
next = queue.get()
temp_distance = abs(next - current)
if temp_distance < min_distance and (next - current) * direction >= 0:
min_distance = temp_distance
min_index = next
queue.put(next)
if min_index == -1:
direction = -direction
continue
distance += min_distance
current = min_index
queue.get(current)
return distance
def clook(queue, start):
direction = 1
distance = 0
current = start
while not queue.empty():
min_distance = float('inf')
min_index = -1
for i in range(queue.qsize()):
next = queue.get()
temp_distance = abs(next - current)
if temp_distance < min_distance and (next - current) * direction >= 0:
min_distance = temp_distance
min_index = next
queue.put(next)
if min_index == -1:
distance += abs(current - 0)
current = 0
direction = 1
continue
distance += min_distance
current = min_index
queue.get(current)
return distance
if __name__ == '__main__':
queue = queue.Queue()
for i in [98, 183, 37, 122, 14, 124, 65, 67]:
queue.put(i)
start = 53
print('FCFS:', fcfs(queue, start))
queue = queue.Queue()
for i in [98, 183, 37, 122, 14, 124, 65, 67]:
queue.put(i)
start = 53
print('SSTF:', sstf(queue, start))
queue = queue.Queue()
for i in [98, 183, 37, 122, 14, 124, 65, 67]:
queue.put(i)
start = 53
print('SCAN:', scan(queue, start))
queue = queue.Queue()
for i in [98, 183, 37, 122, 14, 124, 65, 67]:
queue.put(i)
start = 53
print('C-SCAN:', cscan(queue, start))
queue = queue.Queue()
for i in [98, 183, 37, 122, 14, 124, 65, 67]:
queue.put(i)
start = 53
print('LOOK:', look(queue, start))
queue = queue.Queue()
for i in [98, 183, 37, 122, 14, 124, 65, 67]:
queue.put(i)
start = 53
print('C-LOOK:', clook(queue, start))
```
这个程序可以模拟FCFS、SSTF、SCAN、C-SCAN、LOOK和C-LOOK等磁盘调度算法的执行过程,并计算出磁头的移动距离。在本程序中,模拟了一个磁盘请求队列,队列中的数字表示磁盘请求的磁道号,start表示磁头的初始位置。
以上就是磁盘调度算法的模拟实现及对比的介绍,希望对你有所帮助。
java实现磁盘调度算法的模拟实现及对比,要求:1、首先假设磁盘磁道数为 1500,磁头初始位置可任意设置。 2、用随机数生成函数产生“磁道号”序列(即磁盘请求的位置),共产生 400 个。其中 50%位 于 0~499,25%分布在 500~999,25%分布在 1000~1499。具体的产生方法可参考“题目一 页面 置换算法的模拟实现及命中率对比”。 3、计算及比较每种磁盘调度算法下的磁头移动道数。。本题目要求给出图形可视化界面,并且能够动态模拟每个算法的调度过程,可采用从上一 个请求到下一个请求进行连线的方式。
这是一个相对复杂的任务,需要涉及到磁盘调度算法、随机数生成、图形可视化等多方面知识。以下是一种可能的实现思路:
1. 磁盘调度算法的实现
本题要求比较多种磁盘调度算法的磁头移动道数,因此需要实现多种算法。以下列举几种常用的算法:
- 先来先服务算法(FCFS):按照请求的顺序依次处理,不考虑磁头的移动方向。
- 最短寻道时间优先算法(SSTF):选择距离当前磁头位置最近的请求进行处理,直到所有请求都被处理完毕。
- 扫描算法(SCAN):磁头按照一个方向依次移动,直到到达磁盘的边界,然后改变方向继续移动,直到处理完所有请求。
- 循环扫描算法(C-SCAN):类似于 SCAN 算法,但是在到达磁盘边界时会直接返回到另一端的起点处,继续处理请求。
- 电梯算法(LOOK):类似于 SCAN 算法,但是在到达磁盘边界时不会直接返回,而是改变方向继续移动,直到处理完所有请求。
这些算法的实现可以参考相关的算法描述,但需要注意的是,对于 SCAN、C-SCAN 和 LOOK 算法,需要先将请求按照磁道号排序,以便更好地模拟磁头的移动。
2. 随机数生成函数的实现
本题要求使用随机数生成函数产生“磁道号”序列,可以使用 Java 中的 Random 类来实现。具体的代码如下:
```java
Random random = new Random();
int[] requests = new int[400];
for (int i = 0; i < 400; i++) {
int r = random.nextInt(100);
if (r < 50) {
requests[i] = random.nextInt(500);
} else if (r < 75) {
requests[i] = 500 + random.nextInt(500);
} else {
requests[i] = 1000 + random.nextInt(500);
}
}
```
这段代码首先创建了一个 Random 对象,然后生成了一个长度为 400 的请求序列 requests。对于每个请求,使用 nextInt() 方法生成一个 0 到 99 之间的随机整数 r,根据 r 的值将请求分配到不同的磁道范围中。其中,50% 的请求分布在 0 到 499 之间,25% 分布在 500 到 999 之间,25% 分布在 1000 到 1499 之间。
3. 图形可视化界面的实现
本题要求给出图形可视化界面,并且能够动态模拟每个算法的调度过程,可采用从上一个请求到下一个请求进行连线的方式。可以使用 JavaFX 来实现图形界面。以下是一种可能的实现思路:
- 创建一个 Canvas 对象,用于绘制磁盘和请求的位置。
- 创建一个 Pane 对象,用于显示 Canvas 和按钮等控件。
- 在 Pane 上添加多个按钮,分别对应不同的磁盘调度算法。
- 当用户点击某个按钮时,根据所选择的算法计算磁头移动道数,并将请求序列按照处理顺序绘制在 Canvas 上。
- 在绘制请求时,可以先将所有请求按照磁道号排序,然后依次绘制,使用线段连接相邻的请求,以模拟磁头的移动。
具体的代码实现需要考虑较多细节,包括界面布局、事件处理、图形绘制等方面。以下是一个简单的示例代码,仅供参考:
```java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.control.Button;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
import java.util.Arrays;
public class DiskSchedulingSimulation extends Application {
private static final int DISK_SIZE = 1500;
private static final int REQUEST_COUNT = 400;
private int[] requests = new int[REQUEST_COUNT];
private int headPosition = DISK_SIZE / 2;
private Canvas canvas;
private GraphicsContext gc;
@Override
public void start(Stage primaryStage) {
// 生成请求序列
generateRequests();
// 创建界面元素
canvas = new Canvas(800, 600);
gc = canvas.getGraphicsContext2D();
Pane pane = new Pane(canvas);
Button fcfsButton = new Button("FCFS");
fcfsButton.setOnAction(event -> runAlgorithm("FCFS"));
Button sstfButton = new Button("SSTF");
sstfButton.setOnAction(event -> runAlgorithm("SSTF"));
Button scanButton = new Button("SCAN");
scanButton.setOnAction(event -> runAlgorithm("SCAN"));
Button cscanButton = new Button("C-SCAN");
cscanButton.setOnAction(event -> runAlgorithm("C-SCAN"));
Button lookButton = new Button("LOOK");
lookButton.setOnAction(event -> runAlgorithm("LOOK"));
BorderPane root = new BorderPane(pane, null, null, Arrays.asList(fcfsButton, sstfButton, scanButton, cscanButton, lookButton));
// 显示界面
Scene scene = new Scene(root);
primaryStage.setScene(scene);
primaryStage.setTitle("Disk Scheduling Simulation");
primaryStage.show();
}
private void generateRequests() {
for (int i = 0; i < REQUEST_COUNT; i++) {
int r = (int) (Math.random() * 100);
if (r < 50) {
requests[i] = (int) (Math.random() * 500);
} else if (r < 75) {
requests[i] = 500 + (int) (Math.random() * 500);
} else {
requests[i] = 1000 + (int) (Math.random() * 500);
}
}
Arrays.sort(requests);
}
private void runAlgorithm(String algorithmName) {
int[] result = null;
switch (algorithmName) {
case "FCFS":
result = fcfs();
break;
case "SSTF":
result = sstf();
break;
case "SCAN":
result = scan();
break;
case "C-SCAN":
result = cscan();
break;
case "LOOK":
result = look();
break;
}
if (result != null) {
drawRequests(result);
}
}
private int[] fcfs() {
int[] result = new int[REQUEST_COUNT];
for (int i = 0; i < REQUEST_COUNT; i++) {
result[i] = requests[i];
}
return result;
}
private int[] sstf() {
int[] result = new int[REQUEST_COUNT];
boolean[] visited = new boolean[REQUEST_COUNT];
for (int i = 0; i < REQUEST_COUNT; i++) {
int minDist = Integer.MAX_VALUE;
int minIndex = -1;
for (int j = 0; j < REQUEST_COUNT; j++) {
if (!visited[j]) {
int dist = Math.abs(requests[j] - headPosition);
if (dist < minDist) {
minDist = dist;
minIndex = j;
}
}
}
result[i] = requests[minIndex];
visited[minIndex] = true;
headPosition = requests[minIndex];
}
return result;
}
private int[] scan() {
int[] result = new int[REQUEST_COUNT];
int[] tmpRequests = Arrays.copyOf(requests, REQUEST_COUNT);
Arrays.sort(tmpRequests);
int index = Arrays.binarySearch(tmpRequests, headPosition);
if (index < 0) {
index = -index - 1;
}
int[] leftRequests = Arrays.copyOfRange(tmpRequests, 0, index);
int[] rightRequests = Arrays.copyOfRange(tmpRequests, index, REQUEST_COUNT);
int leftIndex = leftRequests.length - 1;
int rightIndex = 0;
int i = 0;
while (leftIndex >= 0 || rightIndex < rightRequests.length) {
if (leftIndex >= 0 && (rightIndex >= rightRequests.length || headPosition - leftRequests[leftIndex] < rightRequests[rightIndex] - headPosition)) {
result[i++] = leftRequests[leftIndex--];
} else {
result[i++] = rightRequests[rightIndex++];
}
headPosition = result[i - 1];
}
return result;
}
private int[] cscan() {
int[] result = new int[REQUEST_COUNT];
int[] tmpRequests = Arrays.copyOf(requests, REQUEST_COUNT);
Arrays.sort(tmpRequests);
int index = Arrays.binarySearch(tmpRequests, headPosition);
if (index < 0) {
index = -index - 1;
}
int[] leftRequests = Arrays.copyOfRange(tmpRequests, 0, index);
int[] rightRequests = Arrays.copyOfRange(tmpRequests, index, REQUEST_COUNT);
int leftIndex = leftRequests.length - 1;
int rightIndex = 0;
int i = 0;
while (leftIndex >= 0 || rightIndex < rightRequests.length) {
if (leftIndex >= 0 && (rightIndex >= rightRequests.length || headPosition - leftRequests[leftIndex] < rightRequests[rightIndex] - headPosition)) {
result[i++] = leftRequests[leftIndex--];
} else {
result[i++] = rightRequests[rightIndex++];
}
headPosition = result[i - 1];
}
if (result[REQUEST_COUNT - 1] != DISK_SIZE - 1) {
result[i++] = DISK_SIZE - 1;
}
for (int j = 0; j < leftRequests.length; j++) {
result[i++] = leftRequests[j];
}
for (int j = 0; j < rightRequests.length; j++) {
result[i++] = rightRequests[j];
}
return result;
}
private int[] look() {
int[] result = new int[REQUEST_COUNT];
int[] tmpRequests = Arrays.copyOf(requests, REQUEST_COUNT);
Arrays.sort(tmpRequests);
int index = Arrays.binarySearch(tmpRequests, headPosition);
if (index < 0) {
index = -index - 1;
}
int[] leftRequests = Arrays.copyOfRange(tmpRequests, 0, index);
int[] rightRequests = Arrays.copyOfRange(tmpRequests, index, REQUEST_COUNT);
int leftIndex = leftRequests.length - 1;
int rightIndex = 0;
int i = 0;
while (leftIndex >= 0 || rightIndex < rightRequests.length) {
if (leftIndex >= 0 && (rightIndex >= rightRequests.length || headPosition - leftRequests[leftIndex] < rightRequests[rightIndex] - headPosition)) {
result[i++] = leftRequests[leftIndex--];
} else {
result[i++] = rightRequests[rightIndex++];
}
headPosition = result[i - 1];
}
return result;
}
private void drawRequests(int[] result) {
gc.clearRect(0, 0, canvas.getWidth(), canvas.getHeight());
gc.setFill(Color.LIGHTGRAY);
gc.fillRect(0, 0, canvas.getWidth(), canvas.getHeight());
gc.setStroke(Color.BLACK);
gc.strokeRect(100, 100, 600, 400);
gc.setFill(Color.BLUE);
for (int i = 0; i < REQUEST_COUNT; i++) {
double x = 100 + 600.0 * requests[i] / DISK_SIZE;
double y = 500 - 400.0 * i / REQUEST_COUNT;
gc.fillOval(x - 3, y - 3, 6, 6);
}
gc.setStroke(Color.RED);
gc.setLineWidth(2);
double lastX = 100 + 600.0 * headPosition / DISK_SIZE;
double lastY = 500;
for (int i = 0; i < REQUEST_COUNT; i++) {
double x = 100 + 600.0 * result[i] / DISK_SIZE;
double y = 500 - 400.0 * i / REQUEST_COUNT;
gc.strokeLine(lastX, lastY, x, y);
lastX = x;
lastY = y;
}
}
public static void main(String[] args) {
launch(args);
}
}
```
这段代码定义了一个 DiskSchedulingSimulation 类,继承自 Application 类,并重写了 start() 方法。在 start() 方法中,首先生成请求序列,然后创建界面元素,包括 Canvas 对象、Pane 对象和多个按钮。每个按钮对应一个磁盘调度算法,点击按钮时会计算磁头移动道数并绘制请求序列。具体的算法实现在 fcfs()、sstf()、scan()、cscan() 和 look() 方法中,其中 scan()、cscan() 和 look() 方法使用了类似的代码实现,只是在最后绘制请求时略有不同。最后,drawRequests() 方法用于绘制请求序列和磁头移动的连线。
注意:由于界面的绘制操作需要在 JavaFX 的 UI 线程中执行,因此不能在算法实现中直接调用 drawRequests() 方法,而需要使用 Platform.runLater() 方法将绘制操作放到 UI 线程中执行。具体的代码实现可以参考上述示例代码。
阅读全文
相关推荐
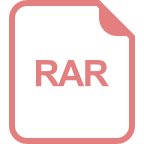
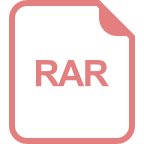
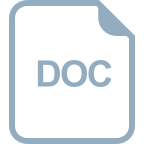
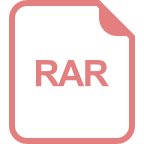
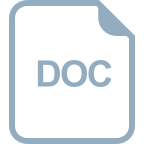



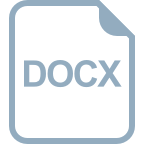
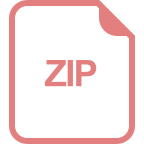