python设计整数顺序表的基本运算程序
时间: 2023-04-24 16:05:00 浏览: 198
Python设计整数顺序表的基本运算程序包括以下几个方面:
1. 创建整数顺序表:可以通过列表或数组等数据结构来创建整数顺序表。
2. 插入元素:可以在顺序表的任意位置插入元素,需要考虑插入位置的合法性和顺序表的扩容问题。
3. 删除元素:可以删除顺序表中的任意元素,需要考虑删除位置的合法性和顺序表的缩容问题。
4. 查找元素:可以根据元素值或下标来查找顺序表中的元素,需要考虑查找的效率和准确性。
5. 修改元素:可以根据元素下标来修改顺序表中的元素值,需要考虑修改的合法性和顺序表的扩容问题。
6. 排序元素:可以对顺序表中的元素进行排序,需要考虑排序算法的选择和实现。
7. 合并顺序表:可以将两个顺序表合并成一个新的顺序表,需要考虑合并的效率和正确性。
以上是Python设计整数顺序表的基本运算程序的主要内容,具体实现可以根据实际需求和具体情况进行调整和优化。
相关问题
(Python)设计整数顺序表的基本运算程序,并用相关数据就行测试。
在Python中,设计一个整数顺序表(也称为线性表或数组),我们可以创建一个名为`IntegerList`的简单类,它包含基本的插入、删除和查找操作。这里是一个基础版本:
```python
class IntegerList:
def __init__(self):
self.items = []
# 插入元素
def insert(self, index, value):
if index < 0 or index > len(self.items):
raise IndexError("Index out of range")
self.items.insert(index, value)
# 删除元素
def remove(self, index):
if index < 0 or index >= len(self.items):
raise IndexError("Index out of range")
del self.items[index]
# 查找元素
def find(self, value):
return self.items.index(value) if value in self.items else -1
# 测试数据和示例操作
test_data = [5, 2, 8, 1, 9]
integer_list = IntegerList()
# 插入元素
integer_list.insert(1, 4)
print(f"Before insertion: {integer_list.items}")
integer_list.insert(0, 0)
print(f"After inserting at index 0: {integer_list.items}")
# 删除元素
integer_list.remove(2)
print(f"After removing element at index 2: {integer_list.items}")
# 查找元素
search_value = 8
result_index = integer_list.find(search_value)
if result_index != -1:
print(f"{search_value} found at index {result_index}")
else:
print(f"{search_value} not found")
设计整数顺序表的基本运算程序,并用相关数据进行测试
好的,以下是基本的设计整数序列表的算法,以及一些相关的数据测试:
假设有一个整数数组 nums,要求对该数组进行排序,可以使用冒泡排序、选择排序、插入排序、快速排序等算法,这里我们以冒泡排序为例进行说明。
冒泡排序的基本思想是通过相邻元素之间的比较和交换来实现排序,具体步骤如下:
1. 比较相邻的元素。如果第一个比第二个大,就交换它们两个。
2. 对每一对相邻的元素做同样的工作,从开始的第一对到结尾的最后一对。这一步完成后,最后的元素会是最大的数。
3. 针对所有的元素重复以上的步骤,除了最后一个。
4. 持续每次对越来越少的元素重复上述步骤,直到没有任何一对数字需要比较。
下面是冒泡排序的Python实现代码:
```python
def bubble_sort(nums):
n = len(nums)
for i in range(n):
for j in range(0, n-i-1):
if nums[j] > nums[j+1]:
nums[j], nums[j+1] = nums[j+1], nums[j]
return nums
```
下面是一些数据测试:
```python
print(bubble_sort([64, 34, 25, 12, 22, 11, 90])) # 输出 [11, 12, 22, 25, 34, 64, 90]
print(bubble_sort([10, 7, 8, 9, 1, 5])) # 输出 [1, 5, 7, 8, 9, 10]
```
希望这能够回答你的问题,如有需要请继续咨询。
阅读全文
相关推荐
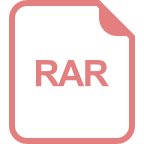


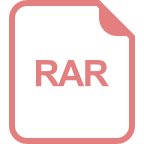











