基于遗传算法的生产排程python代码
时间: 2023-08-26 14:05:26 浏览: 178
以下是一个基于遗传算法的生产排程的 Python 代码示例:
```python
import random
# 定义遗传算法的参数
POPULATION_SIZE = 10
MUTATION_RATE = 0.1
CROSSOVER_RATE = 0.9
NUM_GENERATIONS = 100
# 定义生产任务的参数
NUM_JOBS = 5
NUM_MACHINES = 3
JOB_TIMES = [[2, 1, 4], [3, 2, 1], [1, 3, 2], [4, 2, 3], [2, 1, 3]]
# 定义一个函数来计算每个个体的适应度值
def fitness(individual):
# 初始化每个机器的结束时间
machine_end_times = [0] * NUM_MACHINES
# 计算每个任务的完成时间
for job in individual:
for i in range(NUM_MACHINES):
start_time = max(machine_end_times[i], sum([JOB_TIMES[job][k] for k in range(i)]))
end_time = start_time + JOB_TIMES[job][i]
machine_end_times[i] = end_time
# 计算总的完成时间
total_time = max(machine_end_times)
# 适应度值为完成时间的倒数
return 1 / total_time
# 定义一个函数来生成初始种群
def generate_population():
population = []
for i in range(POPULATION_SIZE):
individual = list(range(NUM_JOBS))
random.shuffle(individual)
population.append(individual)
return population
# 定义一个函数来进行交叉操作
def crossover(parent1, parent2):
# 随机选择一个交叉点
crossover_point = random.randint(1, NUM_JOBS - 1)
# 生成两个子代
child1 = parent1[:crossover_point] + [job for job in parent2 if job not in parent1[:crossover_point]]
child2 = parent2[:crossover_point] + [job for job in parent1 if job not in parent2[:crossover_point]]
return child1, child2
# 定义一个函数来进行变异操作
def mutate(individual):
# 随机选择两个位置进行交换
pos1, pos2 = random.sample(range(NUM_JOBS), 2)
individual[pos1], individual[pos2] = individual[pos2], individual[pos1]
# 定义一个函数来进行选择操作
def select(population):
# 选择两个个体进行比较
parent1, parent2 = random.choices(population, weights=[fitness(individual) for individual in population], k=2)
# 如果第一个个体的适应度值大于第二个个体的适应度值,则选择第一个个体
if fitness(parent1) > fitness(parent2):
return parent1
else:
return parent2
# 生成初始种群
population = generate_population()
# 进行遗传算法的迭代
for generation in range(NUM_GENERATIONS):
# 生成下一代种群
next_population = []
while len(next_population) < POPULATION_SIZE:
# 进行选择操作
parent1 = select(population)
parent2 = select(population)
# 进行交叉操作
if random.random() < CROSSOVER_RATE:
child1, child2 = crossover(parent1, parent2)
else:
child1, child2 = parent1, parent2
# 进行变异操作
if random.random() < MUTATION_RATE:
mutate(child1)
if random.random() < MUTATION_RATE:
mutate(child2)
# 添加子代到下一代种群中
next_population.append(child1)
next_population.append(child2)
# 更新种群
population = next_population
# 打印最优解
best_individual = max(population, key=fitness)
print('最优解:', best_individual)
print('最优解的适应度值:', fitness(best_individual))
```
这个代码演示了如何使用遗传算法来解决生产排程问题。在这个问题中,我们需要将一组生产任务分配给多个机器,使得所有任务都能在最短的时间内完成。遗传算法通过不断进行选择、交叉和变异操作来搜索最优解。在这个例子中,我们使用了一个简单的适应度函数来评估每个个体的适应度值,即完成时间的倒数。最终,我们使用遗传算法找到了最优的任务分配方案。
阅读全文
相关推荐
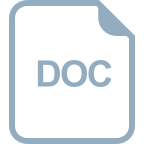
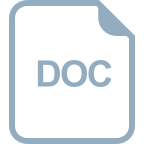
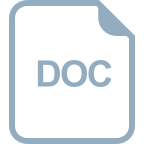
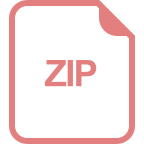
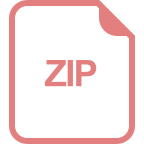
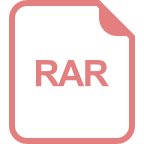
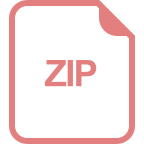
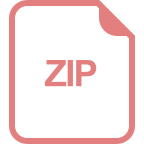
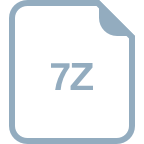
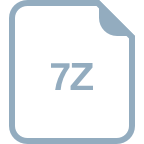
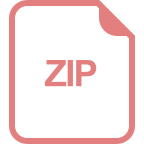
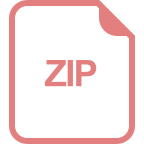
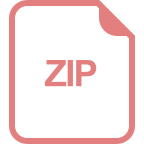




