python pandas merge
时间: 2023-04-24 12:05:09 浏览: 63
Python Pandas中的merge是一种数据合并操作,可以将两个或多个数据框按照指定的列进行合并,类似于SQL中的JOIN操作。通过merge操作,可以将不同数据源的数据整合在一起,方便进行数据分析和处理。
相关问题
python pandas merge合并excel
### 回答1:
可以使用python中的pandas库,通过读取excel文件,并使用merge函数合并。具体步骤为:
1. 使用pandas的read_excel函数读取需要合并的excel文件,并转化为dataframe类型。
2. 使用merge函数将需要合并的dataframe进行合并,根据合并所需的关键列,在on参数中指定。
3. 将合并后的结果保存为需要的格式,如excel文件。
例子:
```python
import pandas as pd
# 读取需要合并的excel文件
df1 = pd.read_excel('file1.xlsx')
df2 = pd.read_excel('file2.xlsx')
# 合并
merged_df = pd.merge(df1, df2, on='key_column')
# 保存为excel
merged_df.to_excel('merged_file.xlsx', index=False)
```
其中,key_column为需要合并的关键列。
### 回答2:
Pandas是一个扩展的Python库,它提供了许多功能来进行数据操作,其中包括对Excel数据的合并。Pandas的merge()函数提供了一种将多个Excel数据表合并为一个数据表的方法。下面是如何使用Pandas库进行Excel数据表的合并操作的步骤。
1.导入Pandas库:首先,必须导入Pandas和NumPy库。Pandas库将用于数据分析和处理,而NumPy库将用于数组操作。
import pandas as pd
import numpy as np
2.读入Excel文件:将要合并的所有Excel文件读入到Pandas DataFrame中。可以使用read_excel方法读入数据。将excel文件读入为数据框。
df1=pd.read_excel("文件路径1")
df2=pd.read_excel("文件路径2")
3.合并Excel文件:使用Pandas的merge()函数将两个DataFrame合并为一个。可以使用类似于SQL inner join,left join,right join和outer join的类型来进行合并。使用merge()函数完成合并。
merged_df=pd.merge(df1,df2,on="id",how="outer")
上述代码中,id是两个数据框共有的行,outer join表示合并两个数据框并保留所有行,以最长的数据框中的行为准。结果merged_df包含合并数据。
4.保存数据:最后,我们可以通过to_excel()函数将合并的数据保存为新Excel文件。
merged_df.to_excel("合并后的文件路径")
总之,使用Pandas的merge()函数可以非常方便地将多个Excel数据表合并为一个数据表,这将大大简化数据操作,提高数据分析的效率。
### 回答3:
Pandas是Python数据处理的一个强大工具,可用于读取、写入、合并和处理各种数据格式,包括Excel文件。在Pandas中,merge是一种合并数据的方法,可以在不同的DataFrame之间共享相同的列名,并将它们合并成一张表。
要在Pandas中合并Excel文件,需要首先加载Excel文件,使用Pandas库的read_excel函数可以读取Excel文件,它将Excel文件读取为Pandas DataFrame。
例如:
import pandas as pd
file1 = pd.read_excel('file1.xlsx')
file2 = pd.read_excel('file2.xlsx')
此时可以使用merge函数将两个DataFrame对象进行合并。需要注意的是,在进行merge操作之前需要确认两个DataFrame中需要合并的列名,这样才能确保merge操作的正确性。
例如,合并file1和file2的'Student ID'列:
merged_file = pd.merge(file1, file2, on='Student ID')
此时合并后的DataFrame将包含file1和file2中的所有行,并且只保留包含共同的'Student ID'的行。如果有名称不同的列需要合并,可以使用left_on和right_on。
例如:
merged_file = pd.merge(file1, file2, left_on='ID', right_on='Student ID')
在合并之后,可以使用to_excel方法将合并后的结果写入一个新的Excel文件中,代码如下:
merged_file.to_excel('merged_file.xlsx',index=False)
可以设置index参数将DataFrame中的索引排除在输出文件之外,这是为了减轻文件大小并避免任何可能的索引问题。
总的来说,Pandas库的merge方法使合并Excel文件变得非常简单和容易,在任何需要合并多个Excel文件或多个DataFrame的情况下,这些步骤都是非常实用和有用的。
pandas merge
Pandas merge is a function that allows you to combine two or more DataFrames based on a common column or index. It is similar to SQL join operations.
Here is an example of how to use the merge function in pandas:
```python
import pandas as pd
# Create two sample DataFrames
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': ['a', 'b', 'c']})
df2 = pd.DataFrame({'A': [3, 4, 5], 'C': ['x', 'y', 'z']})
# Merge the two DataFrames based on the 'A' column
merged_df = pd.merge(df1, df2, on='A')
print(merged_df)
```
This will result in the merged DataFrame with columns 'A', 'B', and 'C', where the rows with matching values in column 'A' are combined.
There are different types of merge operations, such as inner, outer, left, and right merge, which can be specified using the 'how' parameter in the merge function.
相关推荐
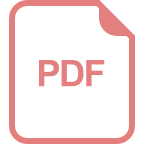
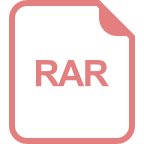
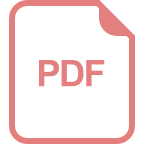
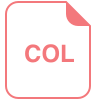
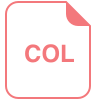
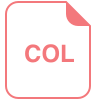
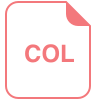
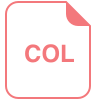







