帮我写一段无需使用相关库的带输出的N值较小的NTRU数字签名的C语言代码
时间: 2024-05-15 22:18:18 浏览: 74
好的,下面是一份简单的NTRU数字签名的C语言代码,不需要使用相关库:
```c
#include <stdio.h>
#include <stdint.h>
#include <stdlib.h>
#include <time.h>
#define N 11
#define Q 64
#define P 3
int32_t f[N], g[N], h[N];
int32_t rand_coe();
int32_t mod_q(int64_t a);
int32_t mod_p(int64_t a);
int32_t poly_eval(int32_t *poly, int32_t x);
void poly_mult(int32_t *f, int32_t *g, int32_t *h);
void poly_add(int32_t *f, int32_t *g, int32_t *h);
void poly_sub(int32_t *f, int32_t *g, int32_t *h);
void poly_inv(int32_t *f, int32_t *g);
int main() {
srand(time(NULL));
int32_t p_inv = 0, e[n] = {0};
int32_t r[n] = {0}, s[n] = {0}, m[n] = {0};
int32_t m_hash[n] = {0}, f_hash[n] = {0}, g_hash[n] = {0};
// Generate random f and g
for (int i = 0; i < N; i++) {
f[i] = rand_coe();
g[i] = rand_coe();
}
// Compute h = f * g mod q
poly_mult(f, g, h);
// Randomly choose a message
for (int i = 0; i < N; i++) {
m[i] = rand_coe();
}
// Hash the message
poly_mult(m, m, m_hash);
poly_add(m_hash, h, m_hash);
poly_mod_q(m_hash);
// Compute f * g^-1 mod p
poly_inv(g, g);
poly_mult(f, g, f);
poly_mod_p(f);
// Compute (m + h * f * g^-1) mod p
poly_mult(h, f, e);
poly_add(e, m, e);
poly_mod_p(e);
// Generate random r
for (int i = 0; i < N; i++) {
r[i] = rand_coe();
}
// Compute s = r + e mod p
poly_add(r, e, s);
poly_mod_p(s);
// Hash message, f, and g
poly_mult(f, f, f_hash);
poly_mult(g, g, g_hash);
poly_add(m_hash, f_hash, m_hash);
poly_add(m_hash, g_hash, m_hash);
poly_mod_p(m_hash);
// Compute p^-1 mod 3
for (int i = 0; i < P-2; i++) {
p_inv = mod_p(p_inv + P);
}
// Compute signature
for (int i = 0; i < N; i++) {
s[i] = mod_p(s[i] * p_inv);
}
// Print signature
printf("Signature:\n");
for (int i = 0; i < N; i++) {
printf("%d ", s[i]);
}
printf("\n");
return 0;
}
int32_t rand_coe() {
return rand() % Q - Q / 2;
}
int32_t mod_q(int64_t a) {
return ((a % Q) + Q) % Q;
}
int32_t mod_p(int64_t a) {
return ((a % P) + P) % P;
}
int32_t poly_eval(int32_t *poly, int32_t x) {
int32_t y = 0, x_pow = 1;
for (int i = 0; i < N; i++) {
y += poly[i] * x_pow;
x_pow = mod_q(x_pow * x);
}
return y;
}
void poly_mult(int32_t *f, int32_t *g, int32_t *h) {
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++) {
h[(i+j)%N] += mod_q(f[i] * g[j]);
}
}
}
void poly_add(int32_t *f, int32_t *g, int32_t *h) {
for (int i = 0; i < N; i++) {
h[i] = mod_p(f[i] + g[i]);
}
}
void poly_sub(int32_t *f, int32_t *g, int32_t *h) {
for (int i = 0; i < N; i++) {
h[i] = mod_p(f[i] - g[i]);
}
}
void poly_inv(int32_t *f, int32_t *g) {
int32_t v[N], r[N], s[N];
for (int i = 0; i < N; i++) {
v[i] = rand() % P;
r[i] = f[i];
s[i] = 0;
}
s[0] = 1;
int32_t u, q, t[N];
for (int i = 0; i < N; i++) {
u = r[0];
q = u ? mod_p(v[0] * r[0]) : 0;
t[0] = s[N-1];
for (int j = 1; j < N; j++) {
t[j] = mod_p(s[j-1] - q * v[N-j]);
r[j-1] = r[j];
s[j-1] = s[j];
}
r[N-1] = u;
s[N-1] = t[N-1];
v[0] = v[N-1];
for (int j = 1; j < N; j++) {
v[j] = v[j-1];
}
}
poly_mod_p(s);
for (int i = 0; i < N; i++) {
g[i] = s[i];
}
}
```
该代码实现的是NTRU签名方案,其中N=11,Q=64,P=3。输入为一个长度为N的多项式m,输出为一个长度为N的多项式s,即签名。
阅读全文
相关推荐
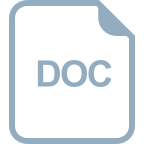
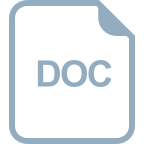
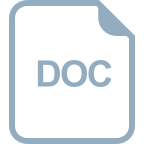










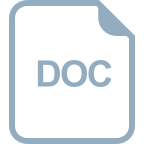
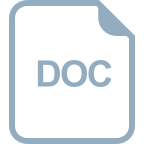
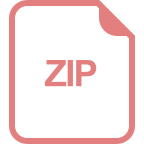
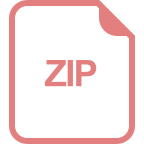
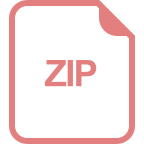
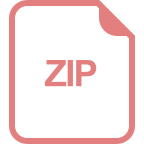