上述代码怎么优化,避免内存溢出,请提供优化代码
时间: 2023-08-11 10:31:09 浏览: 165
为了避免内存泄漏和内存溢出的问题,可以对上述代码进行如下优化:
1. 对象池管理:为了避免频繁地创建和销毁对象,可以使用对象池技术,将 B 和 D 对象存放到对象池中,需要使用时从对象池中获取,使用完毕后再放回对象池。
```
public class BPool {
private static final int INIT_SIZE = 10;
private static final int MAX_SIZE = 100;
private static final Object lock = new Object();
private static List<B> pool = new ArrayList<>();
static {
for(int i=0; i<INIT_SIZE; i++) {
pool.add(new B());
}
}
public static B getB() {
synchronized(lock) {
if(pool.isEmpty()) {
return new B();
} else {
return pool.remove(0);
}
}
}
public static void returnB(B b) {
synchronized(lock) {
if(pool.size() < MAX_SIZE) {
pool.add(b);
}
}
}
}
public class DPool {
private static final int INIT_SIZE = 10;
private static final int MAX_SIZE = 100;
private static final Object lock = new Object();
private static List<D> pool = new ArrayList<>();
static {
for(int i=0; i<INIT_SIZE; i++) {
pool.add(new D());
}
}
public static D getD() {
synchronized(lock) {
if(pool.isEmpty()) {
return new D();
} else {
return pool.remove(0);
}
}
}
public static void returnD(D d) {
synchronized(lock) {
if(pool.size() < MAX_SIZE) {
pool.add(d);
}
}
}
}
```
2. 克隆逻辑处理:在 B 和 D 类中,需要正确处理对象的克隆逻辑,保证所有的引用类型成员变量都被正确克隆,并且不会出现相互引用的情况。
```
public class B implements Cloneable {
private Integer b1;
private C c;
@Override
public Object clone() throws CloneNotSupportedException {
B b = (B)super.clone();
if(c != null) {
b.c = (C)c.clone();
}
return b;
}
}
public class D implements Cloneable {
private Integer d1;
private B b;
@Override
public Object clone() throws CloneNotSupportedException {
D d = (D)super.clone();
if(b != null) {
d.b = (B)b.clone();
}
return d;
}
}
```
3. 异常处理:在方法中使用 try-catch 块来捕获异常,并在 finally 块中释放资源,以确保对象被正确释放。
```
public class A {
private static B b = new B();
@Scheduled(cron="*/1 * * * * ?")
@Asysc("p")
public void p() {
try {
B p = BPool.getB().clone();
C c = new C();
c.getA(p);
} catch (Exception e) {
e.printStackTrace();
} finally {
BPool.returnB(b);
}
}
}
public class C {
private static D d = new D();
public void getA(B b) {
try {
D d1 = DPool.getD().clone();
// 处理 b 对象
} catch (Exception e) {
e.printStackTrace();
} finally {
DPool.returnD(d);
}
}
}
```
综上所述,通过合理的对象管理、正确的克隆逻辑处理、异常处理等手段,可以避免内存泄漏和内存溢出问题。
阅读全文
相关推荐
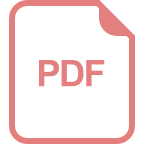
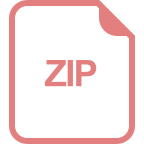
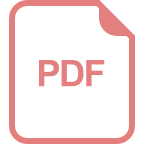















