void SaveInt(int *p,int size,int n,char filename[20]){ FILE *fp; fp=fopen(filename,"wb"); fwrite(p,size,n,fp); fclose(fp); }修改char指向常量
时间: 2024-02-22 12:59:28 浏览: 68
你可以在函数原型中将 `filename` 的类型修改为 `const char*`,表示指向常量的指针,这样就可以避免在函数中修改指针指向的内容。同时,你也需要修改函数中使用 `filename` 的地方,将其修改为只读操作。示例代码如下:
```
void SaveInt(int *p, int size, int n, const char* filename) {
FILE *fp;
int i;
if ((fp = fopen(filename, "wb")) == NULL) {
printf("Cannot open file.\n");
return;
}
for (i = 0; i < n; i++) {
fwrite(&p[i], size, 1, fp);
}
fclose(fp);
}
```
这样修改后,就可以避免在函数中修改 `filename` 指向的内容,从而增强程序的安全性和健壮性。
相关问题
void SaveInt(int *p,int size,int n,char filename[20]) 怎么修改char指向为常量
你可以在函数原型中将 `filename` 的类型修改为 `const char*`,表示指向常量的指针,这样就可以避免在函数中修改指针指向的内容。修改后的函数原型如下:
```
void SaveInt(int *p, int size, int n, const char* filename);
```
同时,你也需要修改函数中使用 `filename` 的地方,将其修改为只读操作,示例代码如下:
```
void SaveInt(int *p, int size, int n, const char* filename) {
FILE *fp;
int i;
if ((fp = fopen(filename, "wb")) == NULL) {
printf("Cannot open file.\n");
return;
}
for (i = 0; i < n; i++) {
fwrite(&p[i], size, 1, fp);
}
fclose(fp);
}
```
这样修改后,就可以避免在函数中修改 `filename` 指向的内容,从而增强程序的安全性和健壮性。
#include <stdio.h> struct stu{ int id; char name[20]; int age; int score; }; int main(){ void readFileForStu(struct stu *p, int num, char filename[],char mode[]); void stdoutForStu(struct stu *p, int num); const int NUM = 2; struct stu students[NUM]; struct stu *p = students; char filename[] = "ex804.txt"; readFileForStu(p, NUM, filename, "r"); stdoutForStu(p, NUM); return 0; } void readFileForStu(struct stu *p, int num, char filename[],char mode[]){ FILE *fp = fopen(filename, mode); if(fp == NULL){ return ; } int i; for(i=0; i<num; i++){ fscanf(fp,"%d %s %d %d",&p[i].id,p[i].name,&p[i].age,&p[i].score); } fclose(fp); } void stdoutForStu(struct stu *p, int num){ int i; for(i=0; i<num; i++){ printf("%d %s %d %d\n",p[i].id,p[i].name,p[i].age,p[i].score); } }设计思想
这段代码实现了从指定文件中读取学生信息,并将读取的结果输出到标准输出中。
在main函数中,定义了一个结构体类型stu,包含学生的id、姓名、年龄和成绩。接着定义了一个常量NUM,指定了需要读取的学生数量,并定义了一个结构体数组students,以及一个指向数组首元素的指针p。还定义了一个要读取的文件名和文件打开模式。
然后调用readFileForStu函数,从指定文件中读取学生信息,将结果存入结构体数组中。接着调用stdoutForStu函数,将学生信息输出到标准输出中。
在readFileForStu函数中,首先通过fopen函数打开指定文件,如果打开失败则返回。然后通过for循环从文件中逐个读取学生信息,将结果存入结构体数组中。
在stdoutForStu函数中,通过for循环将学生信息逐个输出到标准输出中,使用printf函数格式化输出。
总的来说,该代码的设计思想是将读取和输出操作封装成两个函数,提高了代码的可读性和复用性。同时,该代码涉及结构体、文件操作和格式化输出等知识点,可以帮助初学者熟悉这些知识点的使用。
阅读全文
相关推荐
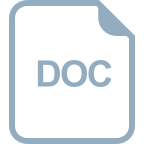
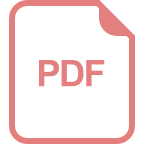
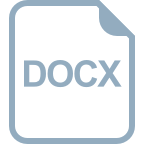












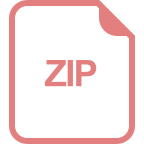