Construct a function lengthNum so that it will only return the length of a list of numbers. (i.e. it will not work for any other type of list, e.g. a list of String).
时间: 2024-01-08 14:02:37 浏览: 29
Here's a possible implementation of the `lengthNum` function in Python:
```python
def lengthNum(lst):
"""
Returns the length of a list of numbers.
Args:
lst: A list of numbers.
Returns:
The length of the list.
Raises:
ValueError: If the list contains non-numeric elements.
"""
for num in lst:
if not isinstance(num, (int, float)):
raise ValueError("List contains non-numeric elements")
return len(lst)
```
The function takes a list `lst` as input and iterates over its elements to check if they are either integers or floats (using the `isinstance` function). If any element is not a number, it raises a `ValueError`. Otherwise, it returns the length of the list using the built-in `len` function.
Here's an example usage of the function:
```python
>>> nums = [1, 2, 3, 4, 5]
>>> lengthNum(nums)
5
>>> nums = [1, 2, 3, "four", 5]
>>> lengthNum(nums)
ValueError: List contains non-numeric elements
```
相关推荐
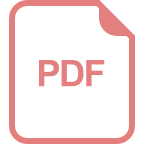
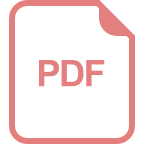
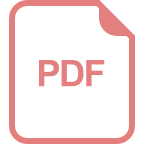














