python threading结束线程 代码
时间: 2023-07-25 17:43:58 浏览: 204
以下是在 Python 中使用 threading 模块的示例代码,演示如何结束一个线程:
```python
import threading
import time
def thread_func():
# 线程函数执行的逻辑
print("Thread is running")
# 结束线程的方法一:在函数内部使用 return 语句
return
t = threading.Thread(target=thread_func) # 创建一个新线程
t.start() # 启动线程
# 结束线程的方法二:等待线程执行完毕
t.join() # 等待线程执行完毕
# 结束线程的方法三:强制结束线程
# t._stop()
```
在上面的示例代码中,我们首先定义了一个名为 `thread_func` 的线程函数,它在执行时会输出一条信息。在主函数中,我们创建了一个新线程 `t`,并将 `thread_func` 函数作为参数传递给它。接着,我们可以通过调用 `t.start()` 方法来启动线程 `t`,然后可以通过调用 `t.join()` 方法来等待线程 `t` 执行完毕。另外,如果需要强制结束线程 `t` 的执行,可以调用 `_stop()` 方法。需要注意的是,这种方法可能会导致一些资源没有被释放,因此应该谨慎使用。
相关问题
python threading销毁线程
在 Python 中,线程的销毁有两种方式:自然结束和强制终止。
1. 自然结束:线程会在完成其任务后自动退出。你可以使用 `threading.Thread.join()` 方法等待线程的完成。这个方法会阻塞当前线程,直到被调用的线程执行完毕。
示例代码:
```python
import threading
import time
def my_function():
print("线程开始")
time.sleep(5)
print("线程结束")
thread = threading.Thread(target=my_function)
thread.start()
thread.join() # 等待线程结束
```
2. 强制终止:如果你需要在某个特定的时间点终止线程,你可以使用线程对象的 `threading.Thread.stop()` 方法。但是需要注意,这个方法在 Python 3 中已经被标记为废弃,因为它可能会导致一些不可预测的问题。推荐的做法是使用一些标志位或者其他方式来控制线程的退出。
示例代码:
```python
import threading
import time
def my_function():
print("线程开始")
while not stop_flag: # 使用标志位控制循环退出
time.sleep(1)
print("线程结束")
stop_flag = False
thread = threading.Thread(target=my_function)
thread.start()
time.sleep(5) # 等待一段时间
stop_flag = True # 修改标志位,终止线程
```
需要注意的是,强制终止线程可能会导致资源泄漏或者不一致的状态,所以最好使用自然结束的方式来销毁线程。
Python threading 多线程
Python threading 是 Python 中用于实现多线程编程的模块。它允许程序在不同的线程中并发地执行多个任务,从而提高程序的执行效率。下面是一个简单的示例,展示了如何使用 threading 模块创建和启动一个线程:
```python
import threading
def print_numbers():
for i in range(1, 6):
print(i)
def print_letters():
for letter in ['A', 'B', 'C', 'D', 'E']:
print(letter)
# 创建子线程
thread1 = threading.Thread(target=print_numbers)
thread2 = threading.Thread(target=print_letters)
# 启动子线程
thread1.start()
thread2.start()
# 等待子线程结束
thread1.join()
thread2.join()
```
这段代码创建了两个线程,分别执行 `print_numbers()` 和 `print_letters()` 函数。通过 `thread1.start()` 和 `thread2.start()` 启动线程,并通过 `thread1.join()` 和 `thread2.join()` 等待线程执行完毕。
阅读全文
相关推荐















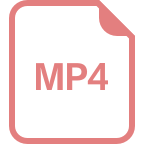