GPIO_InitTypeDef GPIO_InitStructure;USART_InitTypeDef USART_InitStructure;// 初始化GPIO口RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AIN;GPIO_Init(GPIOA, &GPIO_InitStructure);// 初始化串口RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);USART_InitStructure.USART_BaudRate = 115200;USART_InitStructure.USART_WordLength = USART_WordLength_8b;USART_InitStructure.USART_StopBits = USART_StopBits_1;USART_InitStructure.USART_Parity = USART_Parity_No;USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;USART_InitStructure.USART_Mode = USART_Mode_Tx;USART_Init(USART1, &USART_InitStructure);USART_Cmd(USART1, ENABLE);ADC_InitTypeDef ADC_InitStructure;TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;NVIC_InitTypeDef NVIC_InitStructure;// 初始化ADC模块RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1, ENABLE);ADC_InitStructure.ADC_Mode = ADC_Mode_Independent;ADC_InitStructure.ADC_ScanConvMode = DISABLE;ADC_InitStructure.ADC_ContinuousConvMode = ENABLE;ADC_InitStructure.ADC_ExternalTrigConv = ADC_ExternalTrigConv_T3_TRGO;ADC_InitStructure.ADC_DataAlign = ADC_DataAlign_Right;ADC_InitStructure.ADC_NbrOfChannel = 1;ADC_Init(ADC1, &ADC_InitStructure);ADC_Cmd(ADC1, ENABLE);// 初始化定时器RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM3, ENABLE);TIM_TimeBaseStructure.TIM_Period = 72000000 / 1000 - 1; // 计数器自动重装值TIM_TimeBaseStructure.TIM_Prescaler = 72 - 1; // 分频系数TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1; // 时钟分割TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up; // 计数器向上计数TIM_TimeBaseInit(TIM3, &TIM_TimeBaseStructure);// 配置定时器触发ADC采样TIM_SelectOutputTrigger(TIM3, TIM_TRGOSource_Update);ADC_ExternalTrigConvCmd(ADC1, ENABLE);// 初始化定时器中断TIM_ITConfig(TIM3, TIM_IT_Update, ENABLE);NVIC_InitStructure.NVIC_IRQChannel = TIM3_IRQn;NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;NVIC_Init(&NVIC_InitStructure);void TIM3_IRQHandler(void) { if (TIM_GetITStatus(TIM3, TIM_IT_Update) != RESET) { TIM_ClearITPendingBit(TIM3, TIM_IT_Update); ADC_SoftwareStartConvCmd(ADC1, ENABLE); while (ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC) == RESET); uint16_t adcValue = ADC_GetConversionValue(ADC1); USART_SendData(USART1, adcValue >> 8); while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET); USART_SendData(USART1, adcValue & 0xff); while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET); }}
时间: 2023-08-29 14:08:12 浏览: 131
这段代码是 STM32F103C8T6 开发板使用 ADC 和 USART 模块进行模拟量采集并通过串口传输的代码。
1. 初始化 GPIO 口和串口
2. 初始化 ADC 模块
3. 初始化定时器,配置定时器触发 ADC 采样和定时器中断
4. 中断服务函数中,通过 ADC 获取模拟量数值,然后通过 USART 将数值通过串口传输出去
代码注释如下:
```
GPIO_InitTypeDef GPIO_InitStructure; // 定义 GPIO 初始化结构体
USART_InitTypeDef USART_InitStructure; // 定义 USART 初始化结构体
ADC_InitTypeDef ADC_InitStructure; // 定义 ADC 初始化结构体
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure; // 定义定时器初始化结构体
NVIC_InitTypeDef NVIC_InitStructure; // 定义中断初始化结构体
// 初始化 GPIO 口
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE); // 使能 GPIOA 时钟
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0; // 初始化 GPIOA 的第 0 位
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AIN; // GPIOA 的第 0 位设置为模拟输入模式
GPIO_Init(GPIOA, &GPIO_InitStructure); // 初始化 GPIOA
// 初始化串口
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE); // 使能 USART1 时钟
USART_InitStructure.USART_BaudRate = 115200; // 设置波特率为 115200
USART_InitStructure.USART_WordLength = USART_WordLength_8b; // 每个数据帧 8 位
USART_InitStructure.USART_StopBits = USART_StopBits_1; // 一个停止位
USART_InitStructure.USART_Parity = USART_Parity_No; // 无奇偶校验
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None; // 无硬件流控制
USART_InitStructure.USART_Mode = USART_Mode_Tx; // USART1 发送模式
USART_Init(USART1, &USART_InitStructure); // 初始化 USART1
USART_Cmd(USART1, ENABLE); // 使能 USART1
// 初始化 ADC 模块
RCC_APB2PeriphClockCmd(RCC_APB2Periph_ADC1, ENABLE); // 使能 ADC1 时钟
ADC_InitStructure.ADC_Mode = ADC_Mode_Independent; // 独立模式
ADC_InitStructure.ADC_ScanConvMode = DISABLE; // 禁止扫描模式
ADC_InitStructure.ADC_ContinuousConvMode = ENABLE; // 连续转换模式
ADC_InitStructure.ADC_ExternalTrigConv = ADC_ExternalTrigConv_T3_TRGO; // 选择定时器 3 触发采样
ADC_InitStructure.ADC_DataAlign = ADC_DataAlign_Right; // 数据右对齐
ADC_InitStructure.ADC_NbrOfChannel = 1; // 采样通道数为 1
ADC_Init(ADC1, &ADC_InitStructure); // 初始化 ADC1
ADC_Cmd(ADC1, ENABLE); // 使能 ADC1
// 初始化定时器
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM3, ENABLE); // 使能 TIM3 时钟
TIM_TimeBaseStructure.TIM_Period = 72000000 / 1000 - 1; // 设置计数器自动重装值,即定时器周期为 1ms
TIM_TimeBaseStructure.TIM_Prescaler = 72 - 1; // 设置分频系数,即定时器时钟为 1MHz
TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1; // 设置时钟分割
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up; // 计数器向上计数
TIM_TimeBaseInit(TIM3, &TIM_TimeBaseStructure); // 初始化定时器
TIM_SelectOutputTrigger(TIM3, TIM_TRGOSource_Update); // 配置定时器触发 ADC 采样
ADC_ExternalTrigConvCmd(ADC1, ENABLE); // 使能 ADC 外部触发转换
// 初始化定时器中断
TIM_ITConfig(TIM3, TIM_IT_Update, ENABLE); // 使能定时器更新中断
NVIC_InitStructure.NVIC_IRQChannel = TIM3_IRQn; // 设置定时器 3 的中断向量
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0; // 中断抢占优先级为 0
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0; // 中断响应优先级为 0
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; // 使能中断
NVIC_Init(&NVIC_InitStructure); // 初始化中断向量表
// 定时器中断服务函数
void TIM3_IRQHandler(void) {
if (TIM_GetITStatus(TIM3, TIM_IT_Update) != RESET) { // 判断是否为定时器更新中断
TIM_ClearITPendingBit(TIM3, TIM_IT_Update); // 清除定时器更新中断标志位
ADC_SoftwareStartConvCmd(ADC1, ENABLE); // 开始 ADC 转换
while (ADC_GetFlagStatus(ADC1, ADC_FLAG_EOC) == RESET); // 等待 ADC 转换完成
uint16_t adcValue = ADC_GetConversionValue(ADC1); // 获取 ADC 转换结果
USART_SendData(USART1, adcValue >> 8); // 发送高 8 位
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET); // 等待数据发送完成
USART_SendData(USART1, adcValue & 0xff); // 发送低 8 位
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET); // 等待数据发送完成
}
}
```
相关推荐
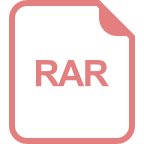
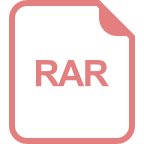















