python生成迷宫小游戏
时间: 2023-07-17 20:58:08 浏览: 64
要创建一个迷宫小游戏,你可以使用Python的Pygame库来实现图形化界面。以下是一个简单的迷宫小游戏的示例代码:
```python
import pygame
import random
# 迷宫大小
WIDTH = 800
HEIGHT = 600
# 迷宫单元格大小
CELL_SIZE = 20
# 颜色定义
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
# 初始化Pygame
pygame.init()
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("迷宫小游戏")
# 创建迷宫地图
maze_width = WIDTH // CELL_SIZE
maze_height = HEIGHT // CELL_SIZE
maze = [['#' for _ in range(maze_width)] for _ in range(maze_height)]
# 随机生成迷宫
def generate_maze(x, y):
directions = [(2, 0), (-2, 0), (0, 2), (0, -2)]
random.shuffle(directions)
for dx, dy in directions:
nx, ny = x + dx, y + dy
if nx >= 0 and ny >= 0 and nx < maze_width and ny < maze_height and maze[ny][nx] == '#':
maze[y][x] = ' '
maze[ny][nx] = ' '
generate_maze(nx, ny)
# 生成迷宫起点和终点
start_x, start_y = random.randint(1, maze_width // 2) * 2, random.randint(1, maze_height // 2) * 2
maze[start_y][start_x] = 'S'
end_x, end_y = random.randint(1, maze_width // 2) * 2, random.randint(1, maze_height // 2) * 2
maze[end_y][end_x] = 'E'
generate_maze(start_x, start_y)
# 游戏循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 绘制迷宫
screen.fill(BLACK)
for y in range(maze_height):
for x in range(maze_width):
rect = pygame.Rect(x * CELL_SIZE, y * CELL_SIZE, CELL_SIZE, CELL_SIZE)
if maze[y][x] == '#':
pygame.draw.rect(screen, WHITE, rect)
elif maze[y][x] == 'S':
pygame.draw.rect(screen, GREEN, rect)
elif maze[y][x] == 'E':
pygame.draw.rect(screen, RED, rect)
pygame.display.flip()
# 退出游戏
pygame.quit()
```
这段代码使用Pygame库来创建一个窗口,并在窗口中绘制迷宫地图。迷宫的生成使用了上述提到的生成迷宫的方法。你可以通过调整`WIDTH`和`HEIGHT`的值来改变窗口大小,通过调整`CELL_SIZE`的值来改变迷宫单元格的大小。
运行代码后,会弹出一个窗口显示迷宫地图。迷宫的起点用绿色方块表示,终点用红色方块表示,墙壁用白色方块表示。你可以按下窗口右上角的关闭按钮来退出游戏。
请注意,这只是一个简单的示例,你可以根据需要进行扩展和修改,如添加人物控制、碰撞检测等功能。
相关推荐
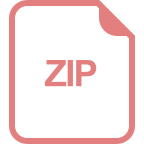
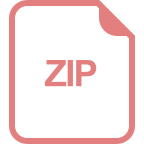
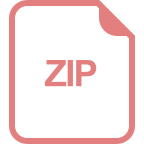
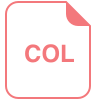
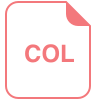
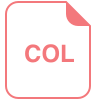











