python迷宫可视化
时间: 2023-09-19 18:00:48 浏览: 57
Python迷宫可视化是一种通过Python编程语言创建并展示迷宫的过程。迷宫是由一系列的墙壁和通道组成的,它们可以在程序中以不同的方式表示。
首先,我们可以选择使用Python中的图形库来创建迷宫的可视化。例如,可以使用Pygame库创建一个游戏画布,并在画布上绘制迷宫的墙壁和通道。墙壁可以用不同的颜色和形状表示,而通道可以用不同的颜色和形状表示。
接下来,我们需要确定迷宫的大小和结构。可以通过创建一个二维数组来表示迷宫的格子。每个格子可以是墙壁或通道的一部分。可以使用0表示墙壁,1表示通道。可以使用随机算法生成或手动设置迷宫的结构。
然后,我们可以使用循环来遍历迷宫的二维数组,并根据格子的值在游戏画布上绘制对应的墙壁或通道。可以使用适当的函数和方法来绘制矩形、线段等图形。
最后,我们可以添加一些额外的功能来增强迷宫可视化的体验。例如,可以实现一个小人(用一个特殊的图形表示)在迷宫中移动的方法。可以使用键盘事件来控制小人的移动,并在移动的过程中更新游戏画布。还可以在游戏画布上添加一些特殊区域或物体,如目标点、陷阱等,以增加迷宫的难度和乐趣。
综上所述,Python迷宫可视化是一种通过编程语言创建、展示和探索迷宫的方法。通过使用适当的库和算法,可以实现一个有趣和可交互的迷宫可视化程序。这个程序可以用来教学、娱乐或解决实际问题,如路径规划等。
相关问题
Python怎么可视化地寻找迷宫最优路径?
在Python中,你可以使用一些可视化库来寻找迷宫的最优路径,其中一个常用的库是`matplotlib`。下面是一个简单的示例代码,演示了如何使用`matplotlib`可视化地寻找迷宫的最优路径:
```python
import matplotlib.pyplot as plt
def visualize_maze(maze, path):
# 获取迷宫的尺寸
rows = len(maze)
cols = len(maze[0])
# 绘制迷宫
plt.figure(figsize=(cols, rows))
plt.imshow(maze, cmap='binary')
# 绘制最优路径
x = []
y = []
for point in path:
x.append(point[1])
y.append(point[0])
plt.plot(x, y, color='red', linewidth=2)
# 设置坐标轴
plt.xticks(range(cols))
plt.yticks(range(rows))
plt.gca().invert_yaxis()
# 显示图像
plt.show()
# 示例迷宫
maze = [
[0, 0, 1, 0, 0],
[0, 1, 0, 1, 0],
[0, 0, 1, 0, 1],
[1, 0, 0, 1, 0],
[0, 1, 0, 0, 0]
]
# 示例最优路径
path = [(0, 0), (1, 0), (2, 0), (2, 1), (2, 2), (3, 2), (4, 2), (4, 3), (4, 4)]
# 可视化迷宫和最优路径
visualize_maze(maze, path)
```
在上面的代码中,`maze`表示迷宫的二维数组,其中0表示可通行的路径,1表示障碍物。`path`表示最优路径,是一个由坐标点组成的列表。通过调用`visualize_maze`函数,你可以将迷宫和最优路径以可视化的方式展示出来。
当然,你也可以根据自己的需求使用其他可视化库或添加更多的功能来优化这个示例代码。
python迷宫小游戏代码_python迷宫游戏,迷宫生成,解决与可视化
以下是一个简单的Python迷宫小游戏代码,包括迷宫生成、解决和可视化。
```python
import random
# 定义迷宫类
class Maze:
def __init__(self, width=10, height=10):
self.width = width
self.height = height
self.grid = self.prepare_grid()
self.configure_cells()
def prepare_grid(self):
return [[Cell(x, y) for y in range(self.height)] for x in range(self.width)]
def configure_cells(self):
for row in self.grid:
for cell in row:
x, y = cell.x, cell.y
cell.north = self.get_cell(x, y-1)
cell.east = self.get_cell(x+1, y)
cell.south = self.get_cell(x, y+1)
cell.west = self.get_cell(x-1, y)
def get_cell(self, x, y):
if x < 0 or y < 0 or x >= self.width or y >= self.height:
return None
return self.grid[x][y]
def __str__(self):
output = "+" + "---+" * self.width + "\n"
for row in self.grid:
top = "|"
bottom = "+"
for cell in row:
if cell is None:
cell = Cell(-1, -1)
body = " "
east_boundary = " " if cell.linked(cell.east) else "|"
top += body + east_boundary
south_boundary = " " if cell.linked(cell.south) else "---"
corner = "+"
bottom += south_boundary + corner
output += top + "\n"
output += bottom + "\n"
return output
# 定义细胞类
class Cell:
def __init__(self, x, y):
self.x = x
self.y = y
self.north = None
self.east = None
self.south = None
self.west = None
self.links = {}
def link(self, cell, bidi=True):
self.links[cell] = True
if bidi:
cell.link(self, False)
return self
def unlink(self, cell, bidi=True):
del self.links[cell]
if bidi:
cell.unlink(self, False)
return self
def linked(self, cell):
return cell is not None and cell in self.links
def neighbors(self):
neighbors = []
if self.north:
neighbors.append(self.north)
if self.east:
neighbors.append(self.east)
if self.south:
neighbors.append(self.south)
if self.west:
neighbors.append(self.west)
return neighbors
# 定义深度优先搜索算法
def recursive_backtracker(maze, current=None):
if current is None:
current = maze.grid[0][0]
stack = [current]
while stack:
current = stack[-1]
neighbors = [n for n in current.neighbors() if not current.linked(n)]
if not neighbors:
stack.pop()
else:
next_cell = random.choice(neighbors)
current.link(next_cell)
stack.append(next_cell)
# 定义广度优先搜索算法
def bfs(maze, start=None, end=None):
if start is None:
start = maze.grid[0][0]
if end is None:
end = maze.grid[maze.width-1][maze.height-1]
queue = [(start, [start])]
visited = set()
while queue:
(vertex, path) = queue.pop(0)
if vertex == end:
return path
if vertex not in visited:
visited.add(vertex)
for neighbor in [n for n in vertex.neighbors() if vertex.linked(n)]:
queue.append((neighbor, path + [neighbor]))
return None
# 定义主函数
def main():
maze = Maze(10, 10)
recursive_backtracker(maze)
print(maze)
path = bfs(maze)
if path:
for cell in path:
maze.grid[cell.x][cell.y] = "P"
print(maze)
if __name__ == "__main__":
main()
```
运行上面的代码会生成一个10x10的迷宫,然后使用深度优先搜索算法生成迷宫,并使用广度优先搜索算法找到从左上角到右下角的最短路径,并将路径用字母"P"标记在迷宫中。
相关推荐
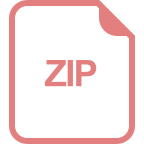
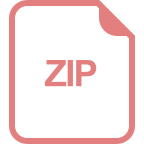
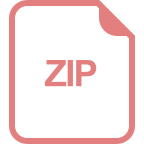












