用python里的pygame库设计一个迷宫小游戏,能随机生成地图,通过键盘控制在迷宫中行走
时间: 2023-06-12 12:07:17 浏览: 93
好的,下面是一个简单的迷宫小游戏的实现,使用了Python中的Pygame库。
首先需要安装Pygame库,可以通过以下命令安装:
```
pip install pygame
```
然后可以使用以下代码实现迷宫小游戏:
```python
import pygame
import random
# 定义迷宫的大小
MAZE_WIDTH = 640
MAZE_HEIGHT = 480
# 定义迷宫的格子大小
CELL_SIZE = 20
# 定义迷宫的行列数
ROW_COUNT = MAZE_HEIGHT // CELL_SIZE
COL_COUNT = MAZE_WIDTH // CELL_SIZE
# 定义迷宫中的墙壁和通道
WALL = 0
PATH = 1
# 定义迷宫的颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
class Maze:
def __init__(self):
self.grid = [[WALL for col in range(COL_COUNT)] for row in range(ROW_COUNT)]
self.generate()
def generate(self):
# 随机选择一个起始点
start_row = random.randint(0, ROW_COUNT - 1)
start_col = random.randint(0, COL_COUNT - 1)
# 将起始点标记为通道
self.grid[start_row][start_col] = PATH
# 递归生成迷宫
self._generate_recursive(start_row, start_col)
def _generate_recursive(self, row, col):
# 随机打乱四个方向的顺序
directions = [(0, -1), (0, 1), (-1, 0), (1, 0)]
random.shuffle(directions)
# 尝试向四个方向扩展迷宫
for direction in directions:
dr, dc = direction
new_row = row + 2 * dr
new_col = col + 2 * dc
# 如果新的位置在迷宫范围内,并且是墙壁
if 0 <= new_row < ROW_COUNT and 0 <= new_col < COL_COUNT and self.grid[new_row][new_col] == WALL:
# 将当前位置和新位置之间的墙壁打通
self.grid[row + dr][col + dc] = PATH
self.grid[new_row][new_col] = PATH
# 递归生成迷宫
self._generate_recursive(new_row, new_col)
def draw(self, surface):
for row in range(ROW_COUNT):
for col in range(COL_COUNT):
if self.grid[row][col] == WALL:
pygame.draw.rect(surface, BLACK, (col * CELL_SIZE, row * CELL_SIZE, CELL_SIZE, CELL_SIZE))
else:
pygame.draw.rect(surface, WHITE, (col * CELL_SIZE, row * CELL_SIZE, CELL_SIZE, CELL_SIZE))
class Player:
def __init__(self):
self.row = 1
self.col = 1
def move(self, direction, maze):
dr, dc = direction
new_row = self.row + dr
new_col = self.col + dc
# 如果新的位置在迷宫范围内,并且是通道
if 0 <= new_row < ROW_COUNT and 0 <= new_col < COL_COUNT and maze.grid[new_row][new_col] == PATH:
self.row = new_row
self.col = new_col
def draw(self, surface):
pygame.draw.circle(surface, (255, 0, 0), (self.col * CELL_SIZE + CELL_SIZE // 2, self.row * CELL_SIZE + CELL_SIZE // 2), CELL_SIZE // 2)
def main():
pygame.init()
screen = pygame.display.set_mode((MAZE_WIDTH, MAZE_HEIGHT))
clock = pygame.time.Clock()
maze = Maze()
player = Player()
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
return
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
player.move((-1, 0), maze)
elif event.key == pygame.K_DOWN:
player.move((1, 0), maze)
elif event.key == pygame.K_LEFT:
player.move((0, -1), maze)
elif event.key == pygame.K_RIGHT:
player.move((0, 1), maze)
# 绘制迷宫和玩家
maze.draw(screen)
player.draw(screen)
# 更新屏幕
pygame.display.update()
# 控制游戏帧率
clock.tick(30)
if __name__ == '__main__':
main()
```
运行以上代码后,可以通过方向键控制玩家在迷宫中行走。每次运行游戏时,都会生成一个随机的迷宫。
相关推荐
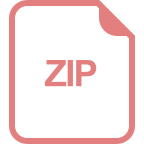
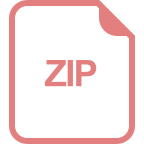
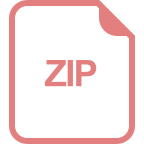














