springboot 查询数据Excle导出
时间: 2023-10-27 18:15:49 浏览: 84
要使用 Spring Boot 导出 Excel,你可以使用 Apache POI 库。以下是一个简单的示例:
1. 添加 Maven 依赖
在 pom.xml 文件中添加以下 Maven 依赖:
```
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
```
2. 创建 POJO 类
创建一个 POJO 类来保存要导出的数据,例如:
```java
public class User {
private String name;
private Integer age;
private String email;
// 省略 getter 和 setter
}
```
3. 创建 Excel 导出服务
创建一个服务类来处理 Excel 导出逻辑,例如:
```java
@Service
public class ExcelExportService {
public void exportUsersToExcel(List<User> users, HttpServletResponse response) throws IOException {
// 创建工作簿
Workbook workbook = new XSSFWorkbook();
// 创建工作表
Sheet sheet = workbook.createSheet("Users");
// 创建表头
Row headerRow = sheet.createRow(0);
headerRow.createCell(0).setCellValue("Name");
headerRow.createCell(1).setCellValue("Age");
headerRow.createCell(2).setCellValue("Email");
// 填充数据
int rowNum = 1;
for (User user : users) {
Row row = sheet.createRow(rowNum++);
row.createCell(0).setCellValue(user.getName());
row.createCell(1).setCellValue(user.getAge());
row.createCell(2).setCellValue(user.getEmail());
}
// 设置响应头
response.setHeader("Content-Disposition", "attachment; filename=users.xlsx");
response.setContentType("application/vnd.openxmlformats-officedocument.spreadsheetml.sheet");
// 写入响应体
workbook.write(response.getOutputStream());
// 关闭工作簿
workbook.close();
}
}
```
4. 创建导出接口
创建一个控制器类来处理导出请求,例如:
```java
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private ExcelExportService excelExportService;
@GetMapping("/export")
public void exportUsers(HttpServletResponse response) throws IOException {
List<User> users = // 查询要导出的数据
excelExportService.exportUsersToExcel(users, response);
}
}
```
这样,当你访问 `/users/export` 接口时,就会将查询到的数据导出为 Excel 文件并下载到本地。
阅读全文
相关推荐
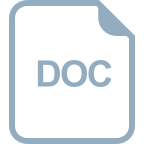
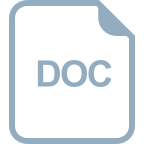
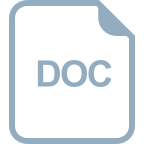
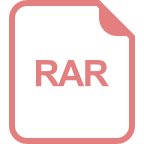
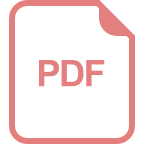



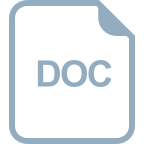








