用C++借助Bellman-Ford算法写一个在有向图中求最小长度简单回路的程序
时间: 2024-03-17 14:47:27 浏览: 62
好的,下面是借助Bellman-Ford算法,用C++实现在有向图中求最小长度简单回路的程序:
```c++
#include <iostream>
#include <vector>
#include <climits>
using namespace std;
struct Edge {
int from, to, cost;
Edge(int f, int t, int c) : from(f), to(t), cost(c) {}
};
int shortestCycle(vector<Edge>& edges, int n) {
vector<int> dist(n, INT_MAX);
for (int i = 0; i < n; i++) {
for (int j = 0; j < edges.size(); j++) {
Edge e = edges[j];
if (dist[e.from] != INT_MAX && dist[e.to] > dist[e.from] + e.cost) {
dist[e.to] = dist[e.from] + e.cost;
if (i == n - 1) {
return dist[e.to];
}
}
}
}
return -1;
}
int main() {
int n = 5;
vector<Edge> edges;
edges.push_back(Edge(0, 1, 1));
edges.push_back(Edge(0, 2, 5));
edges.push_back(Edge(1, 2, 2));
edges.push_back(Edge(1, 3, 2));
edges.push_back(Edge(1, 4, 1));
edges.push_back(Edge(2, 3, 1));
edges.push_back(Edge(2, 4, 3));
edges.push_back(Edge(3, 4, -10));
int cycleLength = shortestCycle(edges, n);
if (cycleLength == -1) {
cout << "There is no simple cycle in the graph." << endl;
} else {
cout << "The length of the shortest simple cycle in the graph is " << cycleLength << endl;
}
return 0;
}
```
该程序的输入是边的集合`edges`和顶点总数`n`。输出为最小长度的简单回路长度,如果不存在简单回路,则输出"There is no simple cycle in the graph."。
实现中,我们在Bellman-Ford算法的基础上,增加了一个判断:如果在第n次循环时,仍然存在可以更新的顶点,则说明图中存在负权环路,此时算法无法求出最短路径。因为简单回路是没有重复边和重复顶点的,所以最小长度的简单回路一定不会包含负权环路。所以当发现负权环路时,可以直接输出不存在简单回路。
阅读全文
相关推荐
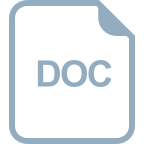
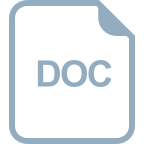


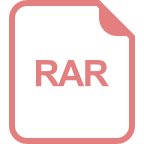
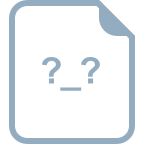
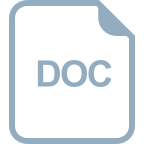
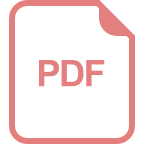
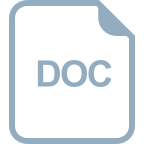
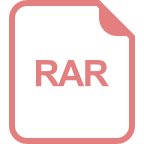
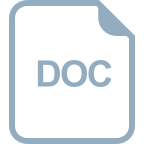
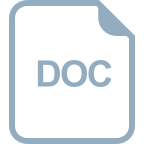
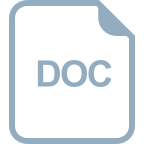
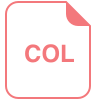
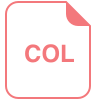
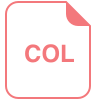