用C++写一个bellman-ford算法
时间: 2023-12-27 08:20:02 浏览: 61
好的,下面是C++实现的Bellman-Ford算法代码:
```c++
#include <iostream>
#include <vector>
#include <climits>
using namespace std;
struct Edge {
int from, to, cost;
Edge(int f, int t, int c) : from(f), to(t), cost(c) {}
};
vector<int> bellmanFord(vector<Edge>& edges, int n, int start) {
vector<int> dist(n, INT_MAX);
dist[start] = 0;
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < edges.size(); j++) {
Edge e = edges[j];
if (dist[e.from] != INT_MAX && dist[e.to] > dist[e.from] + e.cost) {
dist[e.to] = dist[e.from] + e.cost;
}
}
}
return dist;
}
int main() {
int n = 5;
vector<Edge> edges;
edges.push_back(Edge(0, 1, 1));
edges.push_back(Edge(0, 2, 5));
edges.push_back(Edge(1, 2, 2));
edges.push_back(Edge(1, 3, 2));
edges.push_back(Edge(1, 4, 1));
edges.push_back(Edge(2, 3, 1));
edges.push_back(Edge(2, 4, 3));
edges.push_back(Edge(3, 4, 1));
vector<int> dist = bellmanFord(edges, n, 0);
for (int i = 0; i < n; i++) {
cout << "Distance from vertex 0 to vertex " << i << " is " << dist[i] << endl;
}
return 0;
}
```
该算法的输入是边的集合`edges`,顶点总数`n`和起点`start`。输出为从起点到每个顶点的最短距离。实现中使用了一个数组`dist`来存储每个顶点到起点的距离,初始值为无穷大,起点的值为0。然后通过n-1次循环,遍历所有的边,更新`dist`数组中的值。如果某个顶点的距离被更新了,则表明它可以通过更短的路径到达起点。如果在n-1次循环后还存在可以更新的顶点,则说明图中存在负权环路,此时算法无法求出最短路径。
阅读全文
相关推荐
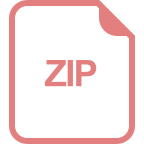

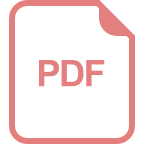
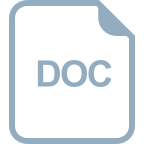
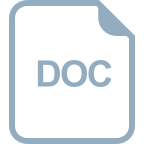
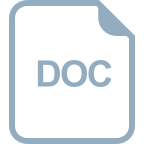
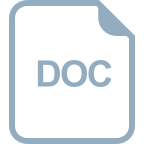

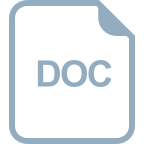
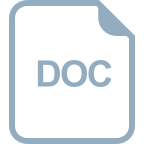
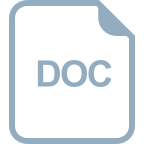


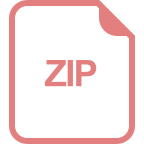
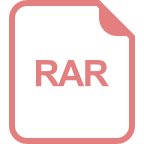