c++ 创建二叉树 查找指定结点的双亲兄弟 并用主函数测试
时间: 2023-08-06 20:06:58 浏览: 54
好的,以下是代码实现:
```c++
#include<iostream>
using namespace std;
// 二叉树结点的结构体
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
// 创建二叉树
TreeNode* createTree() {
int val;
cin >> val;
if (val == -1) {
return NULL;
}
TreeNode* root = new TreeNode(val);
root->left = createTree();
root->right = createTree();
return root;
}
// 查找指定结点的双亲结点
void findParent(TreeNode* root, TreeNode* node, TreeNode*& parent) {
if (root == NULL) {
return;
}
if (root->left == node || root->right == node) {
parent = root;
return;
}
findParent(root->left, node, parent);
findParent(root->right, node, parent);
}
// 查找指定结点的兄弟结点
void findSibling(TreeNode* root, TreeNode* node, TreeNode*& sibling) {
if (root == NULL || root == node) {
return;
}
if (root->left == node) {
sibling = root->right;
return;
}
if (root->right == node) {
sibling = root->left;
return;
}
findSibling(root->left, node, sibling);
findSibling(root->right, node, sibling);
}
int main() {
cout << "请输入二叉树的结点值,-1代表该结点不存在:" << endl;
TreeNode* root = createTree();
cout << "请输入要查找的结点的值:" << endl;
int val;
cin >> val;
// 查找指定结点
TreeNode* node = root;
while (node != NULL) {
if (node->val == val) {
break;
}
else if (node->val > val) {
node = node->left;
}
else {
node = node->right;
}
}
if (node == NULL) {
cout << "该结点不存在!" << endl;
return 0;
}
// 查找双亲结点
TreeNode* parent = NULL;
findParent(root, node, parent);
if (parent == NULL) {
cout << "该结点没有双亲结点!" << endl;
}
else {
cout << "该结点的双亲结点值为:" << parent->val << endl;
}
// 查找兄弟结点
TreeNode* sibling = NULL;
findSibling(root, node, sibling);
if (sibling == NULL) {
cout << "该结点没有兄弟结点!" << endl;
}
else {
cout << "该结点的兄弟结点值为:" << sibling->val << endl;
}
return 0;
}
```
在主函数中,首先通过 `createTree()` 函数创建二叉树,然后输入要查找的结点的值,通过循环遍历二叉树找到该结点。接着,调用 `findParent()` 函数查找该结点的双亲结点,调用 `findSibling()` 函数查找该结点的兄弟结点。最后输出结果,测试完成。
注意,本代码实现的二叉树是一颗排序二叉树,如果需要实现一般的二叉树,只需要修改 `createTree()` 函数即可。
相关推荐
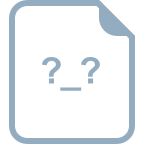
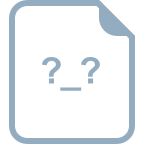















