c++ 创建二叉树 遍历二叉树 计算叶子结点个数和树的深度 二叉树查找指定结点的双亲和兄弟 并用主函数测试
时间: 2024-01-28 08:05:32 浏览: 28
好的,我来为你解答。
首先,我们需要设计一个二叉树的节点结构体,包含左右子树指针和节点值:
```c++
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
};
```
接着,我们可以通过递归的方式来创建二叉树:
```c++
TreeNode* createTree() {
int val;
cin >> val;
if (val == -1) { // -1 表示空节点
return nullptr;
}
TreeNode* root = new TreeNode(val);
root->left = createTree();
root->right = createTree();
return root;
}
```
创建好二叉树后,我们可以通过递归的方式来遍历二叉树:
```c++
void preOrder(TreeNode* root) { // 前序遍历
if (!root) {
return;
}
cout << root->val << " ";
preOrder(root->left);
preOrder(root->right);
}
void inOrder(TreeNode* root) { // 中序遍历
if (!root) {
return;
}
inOrder(root->left);
cout << root->val << " ";
inOrder(root->right);
}
void postOrder(TreeNode* root) { // 后序遍历
if (!root) {
return;
}
postOrder(root->left);
postOrder(root->right);
cout << root->val << " ";
}
```
计算二叉树的叶子节点个数和深度可以通过递归的方式来实现:
```c++
int countLeaf(TreeNode* root) {
if (!root) {
return 0;
}
if (!root->left && !root->right) {
return 1;
}
return countLeaf(root->left) + countLeaf(root->right);
}
int getDepth(TreeNode* root) {
if (!root) {
return 0;
}
int leftDepth = getDepth(root->left);
int rightDepth = getDepth(root->right);
return max(leftDepth, rightDepth) + 1;
}
```
查找指定节点的双亲和兄弟节点可以通过递归的方式来实现:
```c++
void findSibling(TreeNode* root, int val, int& parent, int& sibling) {
if (!root || (!root->left && !root->right)) {
return;
}
if (root->left && root->left->val == val) {
parent = root->val;
sibling = (root->right) ? root->right->val : -1;
return;
}
if (root->right && root->right->val == val) {
parent = root->val;
sibling = (root->left) ? root->left->val : -1;
return;
}
findSibling(root->left, val, parent, sibling);
findSibling(root->right, val, parent, sibling);
}
int findParent(TreeNode* root, int val) {
if (!root || (!root->left && !root->right)) {
return -1;
}
if ((root->left && root->left->val == val) || (root->right && root->right->val == val)) {
return root->val;
}
int leftParent = findParent(root->left, val);
if (leftParent != -1) {
return leftParent;
}
int rightParent = findParent(root->right, val);
if (rightParent != -1) {
return rightParent;
}
return -1;
}
```
最后,我们可以在主函数中测试这些函数:
```c++
int main() {
TreeNode* root = createTree();
cout << "前序遍历:";
preOrder(root);
cout << endl;
cout << "中序遍历:";
inOrder(root);
cout << endl;
cout << "后序遍历:";
postOrder(root);
cout << endl;
cout << "叶子节点个数:" << countLeaf(root) << endl;
cout << "树的深度:" << getDepth(root) << endl;
int parent = -1, sibling = -1;
findSibling(root, 4, parent, sibling);
cout << "节点 4 的双亲为:" << findParent(root, 4) << ",兄弟为:" << sibling << endl;
return 0;
}
```
这样就完成了二叉树的创建、遍历、计算叶子节点个数和深度、查找指定节点的双亲和兄弟节点的操作。
相关推荐
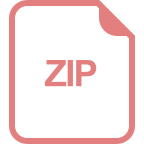
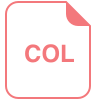
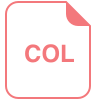
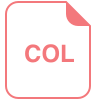
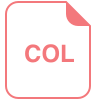
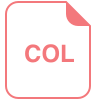









