写verilog关于Spi在线逻辑升级接受bit文件,通过串口接收
时间: 2023-11-27 13:54:23 浏览: 31
以下是一个简单的Verilog代码,用于实现SPI在线逻辑升级接收bit文件,并通过串口进行通信。这个代码仅供参考,具体实现可能因不同的应用场景而有所不同。
```
module spi_logic_upgrade (
input clk,
input reset,
input spi_clk,
input spi_cs_n,
input spi_mosi,
output spi_miso,
input uart_rx,
output uart_tx
);
// 定义一些常量
parameter STATE_IDLE = 2'b00;
parameter STATE_HEADER_RECEIVED = 2'b01;
parameter STATE_DATA_RECEIVED = 2'b10;
// 定义一些变量
reg [7:0] header;
reg [31:0] data;
reg [1:0] state;
reg [7:0] count;
reg [31:0] crc;
// 定义一些常量
localparam HEADER_START = 8'hAA;
localparam HEADER_END = 8'h55;
// 定义一些子模块
wire [7:0] crc_value;
crc16 crc_inst (
.clk(clk),
.reset(reset),
.data({header, data[count]}),
.crc(crc_value)
);
// SPI接口
spi_slave spi_inst (
.clk(clk),
.cs_n(spi_cs_n),
.mosi(spi_mosi),
.miso(spi_miso),
.sclk(spi_clk),
.data_out({crc_value[7:0], crc_value[15:8]})
);
// 串口接口
uart_rx uart_rx_inst (
.clk(clk),
.reset(reset),
.uart_rx(uart_rx),
.data({header, data[count]})
);
uart_tx uart_tx_inst (
.clk(clk),
.reset(reset),
.data({crc_value[7:0], crc_value[15:8]}),
.uart_tx(uart_tx)
);
// 状态机
always @(posedge clk or posedge reset) begin
if (reset) begin
state <= STATE_IDLE;
count <= 0;
crc <= 0;
end else begin
case (state)
STATE_IDLE: begin
if (header == HEADER_START) begin
state <= STATE_HEADER_RECEIVED;
count <= 0;
crc <= 0;
end
end
STATE_HEADER_RECEIVED: begin
if (header == HEADER_END) begin
state <= STATE_DATA_RECEIVED;
count <= 0;
crc <= 0;
end else begin
count <= count + 1;
crc <= crc_value;
end
end
STATE_DATA_RECEIVED: begin
if (count == 31) begin
state <= STATE_IDLE;
count <= 0;
crc <= 0;
end else begin
count <= count + 1;
crc <= crc_value;
end
end
default: begin
state <= STATE_IDLE;
count <= 0;
crc <= 0;
end
endcase
end
end
endmodule
// CRC16模块
module crc16 (
input clk,
input reset,
input [15:0] data,
output reg [15:0] crc
);
reg [15:0] poly;
reg [15:0] shift_reg;
initial begin
poly = 16'h8005;
shift_reg = 16'hFFFF;
end
always @(posedge clk or posedge reset) begin
if (reset) begin
shift_reg <= 16'hFFFF;
end else begin
for (int i = 0; i < 16; i = i + 1) begin
if ((shift_reg[15] ^ data[i]) == 1) begin
shift_reg = {shift_reg[14:0], 1'b1} ^ poly;
end else begin
shift_reg = {shift_reg[14:0], 1'b0};
end
end
end
end
assign crc = shift_reg;
endmodule
// SPI从设备模块
module spi_slave (
input clk,
input cs_n,
input mosi,
output miso,
input sclk,
output [15:0] data_out
);
reg [15:0] data_in;
reg [3:0] bit_count;
reg [15:0] data_out_reg;
reg [1:0] state;
// 定义一些常量
localparam STATE_IDLE = 2'b00;
localparam STATE_RECEIVING = 2'b01;
// SPI状态机
always @(posedge sclk) begin
if (cs_n == 1'b0) begin
if (state == STATE_IDLE) begin
bit_count <= 0;
state <= STATE_RECEIVING;
end else begin
bit_count <= bit_count + 1;
data_in[bit_count] <= mosi;
if (bit_count == 15) begin
data_out_reg <= data_in;
state <= STATE_IDLE;
end
end
end
end
// SPI输出数据
assign miso = (cs_n == 1'b0) ? data_out_reg[bit_count] : 1'bZ;
assign data_out = (cs_n == 1'b0) ? data_out_reg : 16'hFFFF;
endmodule
// 串口接收模块
module uart_rx (
input clk,
input reset,
input uart_rx,
output reg [7:0] data
);
reg [3:0] bit_count;
reg [1:0] state;
reg [7:0] shift_reg;
// 定义一些常量
localparam STATE_IDLE = 2'b00;
localparam STATE_RECEIVING = 2'b01;
// 串口状态机
always @(posedge clk or posedge reset) begin
if (reset) begin
state <= STATE_IDLE;
bit_count <= 0;
end else begin
case (state)
STATE_IDLE: begin
if (uart_rx == 1'b0) begin
state <= STATE_RECEIVING;
bit_count <= 0;
shift_reg <= 0;
end
end
STATE_RECEIVING: begin
bit_count <= bit_count + 1;
shift_reg <= {shift_reg[6:0], uart_rx};
if (bit_count == 7) begin
state <= STATE_IDLE;
data <= shift_reg;
end
end
default: begin
state <= STATE_IDLE;
bit_count <= 0;
end
endcase
end
end
endmodule
// 串口发送模块
module uart_tx (
input clk,
input reset,
input [7:0] data,
output reg uart_tx
);
reg [3:0] bit_count;
reg [1:0] state;
reg [7:0] shift_reg;
// 定义一些常量
localparam STATE_IDLE = 2'b00;
localparam STATE_SENDING = 2'b01;
// 串口状态机
always @(posedge clk or posedge reset) begin
if (reset) begin
state <= STATE_IDLE;
bit_count <= 0;
end else begin
case (state)
STATE_IDLE: begin
shift_reg <= data;
bit_count <= 0;
uart_tx <= 1'b1;
state <= STATE_SENDING;
end
STATE_SENDING: begin
bit_count <= bit_count + 1;
uart_tx <= shift_reg[0];
shift_reg <= {shift_reg[6:0], 1'b0};
if (bit_count == 7) begin
state <= STATE_IDLE;
uart_tx <= 1'b1;
end
end
default: begin
state <= STATE_IDLE;
bit_count <= 0;
uart_tx <= 1'b1;
end
endcase
end
end
endmodule
```
相关推荐
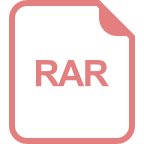
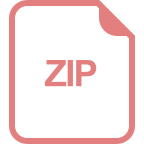
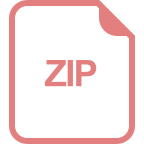













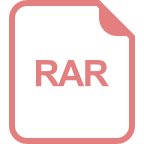
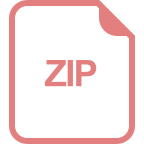