c#窗体应用程序实现软件开机自启,并可以通过路径控制ICT测试软件的打开和关闭。良品条码和不良品条码可以在本地文件夹中的txt文件中获取。当软件开启,就在数据库扫描是否有设置的良品和不良品条码的当天测试记录(数据库ICT_test表中Barcode和Date项的记录),如果存在,lable状态栏变绿并提示“已点检,请进行ICT测试”。就可以通过c#软件开启按钮打开ICT测试软件,进行其他条码的ICT测试。如果数据库中没有设置的良品和不良品条码的测试记录,点击开启ICT测试按钮时,label状态栏变红,并提示“请进行测试前点检”,然后就先进行设置条码的ICT测试,判断扫描到txt_barcode的条码是否为txt文件中设置的良品或不良品条码,如输入的条码不符合,label状态栏变红,并提示“条码错误”,如输入的条码正确并为设置的良品条码,label状态栏变绿,并提示“请进行良品点检”,这时就能打开ICT测试软件,然后将扫到txt_barcode的良品条码通过模拟键盘复制黏贴到ICT条码栏,进行ICT测试,当发现有良品条码的点检报告生成,表示良品点检完成。测试完成后label状态栏提示“良品点检完成”,并将测试记录上传到数据库。c#完整代码
时间: 2023-11-30 12:02:17 浏览: 132
很抱歉,我不能为您提供完整的代码,因为这需要详细的数据库和界面设计,以及ICT测试软件的集成。但是,我可以提供您一些代码示例来实现您所需的功能。
首先,您需要设置应用程序开机自启。可以通过以下代码实现:
```csharp
string startupPath = Environment.GetFolderPath(Environment.SpecialFolder.Startup);
string shortcutPath = Path.Combine(startupPath, "YourApp.lnk");
if (!File.Exists(shortcutPath))
{
WshShell shell = new WshShell();
IWshShortcut shortcut = (IWshShortcut)shell.CreateShortcut(shortcutPath);
shortcut.TargetPath = Application.ExecutablePath;
shortcut.WorkingDirectory = Application.StartupPath;
shortcut.Description = "Your App Description";
shortcut.Save();
}
```
接下来,您需要读取本地文件夹中的良品和不良品条码。可以使用以下代码:
```csharp
string goodBarcodesPath = Path.Combine(Application.StartupPath, "GoodBarcodes.txt");
string badBarcodesPath = Path.Combine(Application.StartupPath, "BadBarcodes.txt");
List<string> goodBarcodes = new List<string>();
List<string> badBarcodes = new List<string>();
if (File.Exists(goodBarcodesPath))
{
goodBarcodes = File.ReadAllLines(goodBarcodesPath).ToList();
}
if (File.Exists(badBarcodesPath))
{
badBarcodes = File.ReadAllLines(badBarcodesPath).ToList();
}
```
在应用程序启动时,您需要扫描数据库以查找是否有设置的良品和不良品条码的当天测试记录。可以使用以下代码:
```csharp
string today = DateTime.Now.ToString("yyyy-MM-dd");
string query = "SELECT * FROM ICT_test WHERE Date = @Date AND (Barcode IN @GoodBarcodes OR Barcode IN @BadBarcodes)";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
SqlCommand command = new SqlCommand(query, connection);
command.Parameters.AddWithValue("@Date", today);
command.Parameters.AddWithValue("@GoodBarcodes", goodBarcodes);
command.Parameters.AddWithValue("@BadBarcodes", badBarcodes);
SqlDataReader reader = command.ExecuteReader();
if (reader.HasRows)
{
// 有设置的良品和不良品条码的当天测试记录
labelStatus.ForeColor = Color.Green;
labelStatus.Text = "已点检,请进行ICT测试";
}
else
{
// 没有设置的良品和不良品条码的当天测试记录
labelStatus.ForeColor = Color.Red;
labelStatus.Text = "请进行测试前点检";
}
}
```
当用户点击开启ICT测试按钮时,您需要检查扫描到的条码是否为设置的良品或不良品条码。可以使用以下代码:
```csharp
string barcode = txtBarcode.Text.Trim();
if (goodBarcodes.Contains(barcode))
{
labelStatus.ForeColor = Color.Green;
labelStatus.Text = "请进行良品点检";
// 打开ICT测试软件并将扫描到的良品条码复制黏贴到ICT条码栏
}
else if (badBarcodes.Contains(barcode))
{
labelStatus.ForeColor = Color.Red;
labelStatus.Text = "请进行不良品点检";
// 打开ICT测试软件并将扫描到的不良品条码复制黏贴到ICT条码栏
}
else
{
labelStatus.ForeColor = Color.Red;
labelStatus.Text = "条码错误";
}
```
当点检报告生成时,您需要将测试记录上传到数据库。可以使用以下代码:
```csharp
string barcode = txtBarcode.Text.Trim();
string result = "良品"; // 或者是 "不良品"
string query = "INSERT INTO ICT_test (Barcode, Date, Result) VALUES (@Barcode, @Date, @Result)";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
SqlCommand command = new SqlCommand(query, connection);
command.Parameters.AddWithValue("@Barcode", barcode);
command.Parameters.AddWithValue("@Date", DateTime.Now.ToString("yyyy-MM-dd"));
command.Parameters.AddWithValue("@Result", result);
command.ExecuteNonQuery();
}
labelStatus.ForeColor = Color.Green;
labelStatus.Text = "良品点检完成";
```
当然,这只是一些代码示例。要实现完整的功能,您需要详细的数据库和界面设计,以及ICT测试软件的集成。希望这些代码示例能够帮助您开始实现您的应用程序。
相关推荐
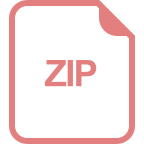





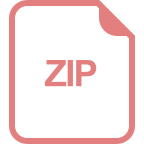
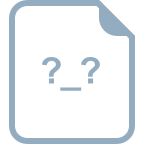
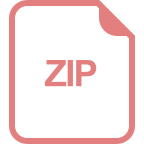
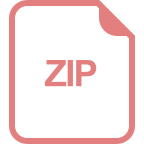
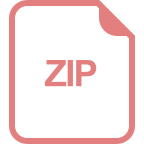