#include <iostream>using namespace std;void fibonacci(int n, int &f0, int &f1) { if (n == 0) { f0 = 0; f1 = 1; } else { fibonacci(n - 1, f1, f0); f1 += f0; }}int main() { int n; cout << "Please input the number of terms of the Fibonacci sequence: "; cin >> n; cout << "The first " << n << " terms of the Fibonacci sequence are: "; int f0 = 0, f1 = 1; for (int i = 0; i < n; i++) { cout << f0 << " "; fibonacci(i, f0, f1); } cout << endl; return 0;} 翻译成mips汇编程序
时间: 2024-01-20 22:02:04 浏览: 30
下面是翻译成MIPS汇编程序的代码:
```
.data
output: .asciiz "The first %d terms of the Fibonacci sequence are: \n"
.align 2
f0: .word 0
f1: .word 1
.text
.globl main
main:
li $v0, 4 # print the output message
la $a0, output
syscall
li $v0, 5 # read in the value of n
syscall
move $s0, $v0 # save the value of n in register s0
la $s1, f0 # load the address of f0 into register s1
la $s2, f1 # load the address of f1 into register s2
addi $s3, $zero, 0 # initialize the counter to 0
j fib # jump to the fib subroutine
fib:
beq $s3, $s0, done # if the counter reaches n, exit the subroutine
lw $t0, 0($s1) # load the value of f0 into register t0
lw $t1, 0($s2) # load the value of f1 into register t1
addi $sp, $sp, -8 # allocate space on the stack for the two arguments
sw $s2, 4($sp) # store the address of f1 on the stack
sw $s1, 0($sp) # store the address of f0 on the stack
addi $s0, $s0, -1 # decrement the value of n
jal fib # recursive call to fib(n-1)
addi $s0, $s0, 1 # increment the value of n
lw $s1, 0($sp) # restore the address of f0 from the stack
lw $s2, 4($sp) # restore the address of f1 from the stack
addi $sp, $sp, 8 # deallocate space on the stack
add $t2, $t0, $t1 # calculate the next term in the sequence
sw $t1, 0($s1) # store the value of f1 in f0
sw $t2, 0($s2) # store the value of f2 in f1
li $v0, 1 # print the current term
move $a0, $t0
syscall
li $v0, 4 # print a space
la $a0, " "
syscall
addi $s3, $s3, 1 # increment the counter
j fib # jump to the beginning of the subroutine
done:
li $v0, 10 # exit the program
syscall
```
这个程序使用了递归的方式来计算斐波那契数列的每一项,并将它们打印出来。在每一次递归调用中,程序使用栈来保存f0和f1两个变量的地址,并将n的值减1传递给递归函数。在递归函数返回时,程序恢复f0和f1的地址,并将它们的值更新为下一个斐波那契数。最后,程序打印出计算出的斐波那契数列的每一项。
相关推荐
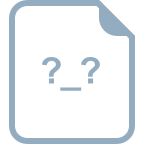
















