请用python写语言一个俄罗斯方块游戏
时间: 2023-12-18 14:04:47 浏览: 94
好的,我可以给您提供一个简易版的俄罗斯方块游戏代码,您可以在此基础上进行改进和完善。
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置游戏窗口大小
screen_width = 300
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置游戏标题
pygame.display.set_caption("俄罗斯方块")
# 定义方块大小
block_size = 30
# 定义方块的形状
shapes = [
[[1, 1, 1],
[0, 1, 0]],
[[0, 2, 2],
[2, 2, 0]],
[[3, 3, 0],
[0, 3, 3]],
[[4, 0, 0],
[4, 4, 4]],
[[0, 0, 5],
[5, 5, 5]],
[[6, 6],
[6, 6]],
[[7, 7, 7, 7]]
]
# 定义方块颜色
colors = [(0, 0, 0), # 黑色
(255, 0, 0), # 红色
(0, 255, 0), # 绿色
(0, 0, 255), # 蓝色
(255, 255, 0), # 黄色
(255, 0, 255), # 紫色
(0, 255, 255), # 青色
(192, 192, 192)] # 灰色
# 随机生成一个方块
current_shape = random.choice(shapes)
current_color = random.choice(colors)
# 定义方块的初始位置
current_row = 0
current_col = 4
# 定义游戏区域
board = [[0 for i in range(10)] for j in range(20)]
# 定义游戏结束标志
game_over = False
# 定义得分
score = 0
# 定义字体
font = pygame.font.Font(None, 36)
# 定义方块下落速度
speed = 1
# 定义计时器
timer = 0
# 游戏循环
while not game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
if current_col > 0:
current_col -= 1
elif event.key == pygame.K_RIGHT:
if current_col < 10 - len(current_shape[0]):
current_col += 1
elif event.key == pygame.K_UP:
current_shape = [[current_shape[j][i] for j in range(len(current_shape))] for i in range(len(current_shape[0]) - 1, -1, -1)]
elif event.key == pygame.K_DOWN:
speed = 10
# 更新计时器
timer += 1
# 方块下落
if timer % (10 - speed) == 0:
if current_row < 20 - len(current_shape):
current_row += 1
else:
# 将方块放入游戏区域
for i in range(len(current_shape)):
for j in range(len(current_shape[0])):
if current_shape[i][j] != 0:
board[current_row + i][current_col + j] = current_color
# 消除满行
for i in range(len(board)):
if 0 not in board[i]:
board.pop(i)
board.insert(0, [0 for j in range(10)])
score += 10
# 生成新的方块
current_shape = random.choice(shapes)
current_color = random.choice(colors)
current_row = 0
current_col = 4
# 绘制游戏区域
screen.fill((255, 255, 255))
for i in range(len(board)):
for j in range(len(board[0])):
if board[i][j] != 0:
pygame.draw.rect(screen, colors[board[i][j]], (j * block_size, i * block_size, block_size, block_size))
# 绘制当前方块
for i in range(len(current_shape)):
for j in range(len(current_shape[0])):
if current_shape[i][j] != 0:
pygame.draw.rect(screen, current_color, ((current_col + j) * block_size, (current_row + i) * block_size, block_size, block_size))
# 绘制得分
score_text = font.render("得分:" + str(score), True, (0, 0, 0))
screen.blit(score_text, (10, 10))
# 更新屏幕
pygame.display.update()
# 退出pygame
pygame.quit()
```
阅读全文
相关推荐
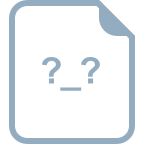











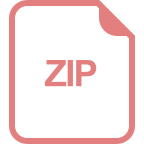
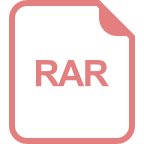
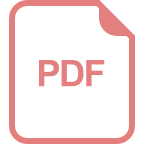