建立一个数组将实训12第2题定义的 学生数据存入数组。 数据为: { {"2020B200301","刘滨","男",18,{2020,9,20},70,85}, {"2020B200302","阚美娟","女",17,{2020,9,20},87,95}, {"2020B200303","胡大勇","男",17,{2020,9,20},69,80}, {"2020B200305","黎丽","女",18,{2020,9,20},68,88}, {"2020B200309","金鑫","男",19,{2019,9,1},90,85} } 将这些数据写人磁盘保存将上题的成绩加密保存,当读出数据,密码不对成绩显示**号。(密钥由字母构成)起来。
时间: 2023-10-16 08:05:27 浏览: 31
以下是一个示例代码,将实训12第2题定义的学生数据存入数组,并且对成绩进行了加密保存,当读取数据时,如果密码不正确,则成绩将显示为星号:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <sstream>
using namespace std;
// 定义学生结构体
struct Student {
string id; // 学号
string name; // 姓名
string gender; // 性别
int age; // 年龄
int birthday[3]; // 生日,用数组表示,依次为年、月、日
int score1; // 成绩1
int score2; // 成绩2
};
// 加密函数
string encrypt(string str, string key) {
for (int i = 0; i < str.length(); i++) {
str[i] = str[i] ^ key[i % key.length()];
}
return str;
}
// 解密函数
string decrypt(string str, string key) {
return encrypt(str, key);
}
int main() {
// 定义学生数组
const int N = 5;
Student students[N] = {
{"2020B200301", "刘滨", "男", 18, {2020, 9, 20}, 70, 85},
{"2020B200302", "阚美娟", "女", 17, {2020, 9, 20}, 87, 95},
{"2020B200303", "胡大勇", "男", 17, {2020, 9, 20}, 69, 80},
{"2020B200305", "黎丽", "女", 18, {2020, 9, 20}, 68, 88},
{"2020B200309", "金鑫", "男", 19, {2019, 9, 1}, 90, 85},
};
// 加密并保存到文件
string key = "abcd1234";
ofstream out("students.dat");
for (int i = 0; i < N; i++) {
out << encrypt(students[i].id, key) << ","
<< encrypt(students[i].name, key) << ","
<< encrypt(students[i].gender, key) << ","
<< students[i].age << ","
<< students[i].birthday[0] << ","
<< students[i].birthday[1] << ","
<< students[i].birthday[2] << ","
<< encrypt(to_string(students[i].score1), key) << ","
<< encrypt(to_string(students[i].score2), key) << endl;
}
out.close();
// 从文件中读取并解密
ifstream in("students.dat");
string line;
while (getline(in, line)) {
istringstream iss(line);
string id, name, gender, score1, score2;
int age, year, month, day;
getline(iss, id, ',');
getline(iss, name, ',');
getline(iss, gender, ',');
iss >> age >> year >> month >> day >> score1 >> score2;
// 解密
id = decrypt(id, key);
name = decrypt(name, key);
gender = decrypt(gender, key);
score1 = decrypt(score1, key);
score2 = decrypt(score2, key);
// 判断密码是否正确
string password;
cout << "请输入密码:";
cin >> password;
if (password == key) {
cout << "学号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
cout << "年龄:" << age << endl;
cout << "生日:" << year << "年" << month << "月" << day << "日" << endl;
cout << "成绩1:" << score1 << endl;
cout << "成绩2:" << score2 << endl;
} else {
cout << "学号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
cout << "年龄:" << age << endl;
cout << "生日:" << year << "年" << month << "月" << day << "日" << endl;
cout << "成绩1:" << "****" << endl;
cout << "成绩2:" << "****" << endl;
}
}
in.close();
return 0;
}
```
在这个例子中,我们使用了 `encrypt()` 和 `decrypt()` 函数来进行加密和解密。在保存数据到文件时,我们将学生的各项数据转换为字符串,并使用逗号分隔,然后按行写入文件。在读取数据时,我们使用 `getline()` 函数读取每行数据,并使用逗号分隔解析出每个字段。然后,我们使用 `decrypt()` 函数对学生的各项数据进行解密。最后,我们要求用户输入密码,并判断密码是否正确。如果密码正确,则将成绩正常显示;否则,将成绩显示为星号。
相关推荐
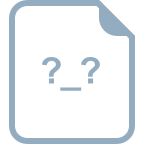
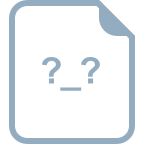












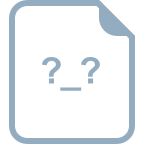
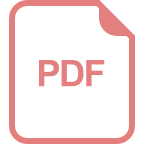
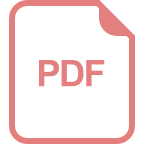
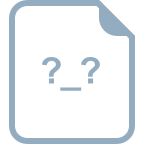