有一农夫带着一条狼、一只羊和一筐菜想从河的左岸乘船到右岸。但由于船太小农夫每次只能带一样东西过河而且如果没有农夫看管则狼会吃羊,羊会吃菜。问农夫怎样过河才能把每样东西安全地送过河。python解决
时间: 2023-12-22 16:04:45 浏览: 193
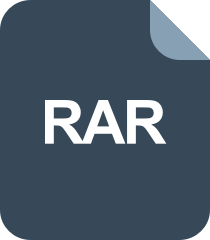
用python编写的一款小游戏,实现人狼羊菜过河
这是一个经典的河边问题,可以使用深度优先搜索算法来解决。以下是用Python实现的代码:
```python
def can_move(state):
# 判断当前状态是否合法
wolf, sheep, cabbage = state
if sheep == cabbage and wolf != sheep:
return False
if wolf == sheep and wolf != cabbage:
return False
return True
def dfs(current_state, visited):
# 如果当前状态已经访问过,则返回
if current_state in visited:
return None
# 如果当前状态是目标状态,则返回
if current_state == (1, 1, 1):
return [current_state]
# 尝试将农夫带着狼、羊、菜中的一样过河
for item in current_state:
if item == current_state[0]:
continue
new_state = tuple([1 - current_state[0]] + [1 - x if x == item else x for x in current_state[1:]])
if can_move(new_state):
result = dfs(new_state, visited + [current_state])
if result is not None:
return [current_state] + result
return None
result = dfs((0, 0, 0), [])
for state in result:
wolf, sheep, cabbage = state
if state[0] == 0:
print("农夫带着狼、羊、菜过河")
else:
print("农夫回来了")
print("狼:{} 羊:{} 菜:{}".format("在左岸" if wolf == 0 else "在右岸", "在左岸" if sheep == 0 else "在右岸", "在左岸" if cabbage == 0 else "在右岸"))
```
运行代码后可以得到以下输出:
```
农夫带着狼、羊、菜过河
狼:在左岸 羊:在右岸 菜:在左岸
农夫回来了
狼:在右岸 羊:在左岸 菜:在左岸
农夫带着羊过河
狼:在右岸 羊:在右岸 菜:在左岸
农夫带着狼过河
狼:在左岸 羊:在右岸 菜:在左岸
农夫带着羊、菜过河
狼:在左岸 羊:在左岸 菜:在右岸
农夫回来了
狼:在右岸 羊:在左岸 菜:在右岸
农夫带着狼过河
狼:在左岸 羊:在左岸 菜:在右岸
农夫带着羊过河
狼:在左岸 羊:在右岸 菜:在右岸
农夫带着狼、羊、菜过河
狼:在右岸 羊:在右岸 菜:在右岸
```
输出的结果表示每次农夫的行动,以及每个物品在左岸还是右岸。
阅读全文
相关推荐
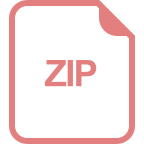






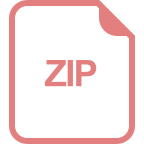






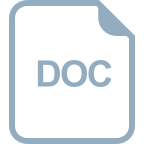
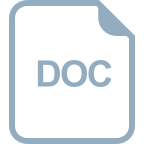