quarkus 下载图片打包成zip文件让web端下载
时间: 2024-01-07 15:05:56 浏览: 28
你可以使用 Quarkus 的 RESTful API 创建一个端点,该端点将接受图片的 URL,并使用 Java ImageIO 库将其下载到本地,然后将所有下载的图片打包成 ZIP 文件并下载到客户端。以下是一个简单的示例代码:
```java
import java.awt.image.BufferedImage;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
import javax.imageio.ImageIO;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.QueryParam;
import javax.ws.rs.core.Response;
import org.jboss.resteasy.annotations.jaxrs.QueryParam;
@Path("/images")
public class ImageResource {
@GET
@Path("/download")
@Produces("application/zip")
public Response downloadImagesAsZip(@QueryParam("urls") List<String> urls) throws IOException {
// Create a ByteArrayOutputStream to store the ZIP file content
ByteArrayOutputStream baos = new ByteArrayOutputStream();
// Create a ZipOutputStream to write the ZIP file content
ZipOutputStream zos = new ZipOutputStream(baos);
// Create a list to store all the downloaded images
List<BufferedImage> images = new ArrayList<>();
// Download all the images and add them to the list
for (String url : urls) {
BufferedImage image = ImageIO.read(new URL(url));
images.add(image);
}
// Write all the images to the ZIP file
for (int i = 0; i < images.size(); i++) {
BufferedImage image = images.get(i);
// Create a new ZIP entry with the image name
ZipEntry entry = new ZipEntry("image_" + i + ".png");
zos.putNextEntry(entry);
// Write the image content to the ZIP file
ImageIO.write(image, "png", zos);
zos.closeEntry();
}
// Close the ZipOutputStream
zos.close();
// Convert the ByteArrayOutputStream to a byte array
byte[] data = baos.toByteArray();
// Create a Response object with the ZIP file content
Response.ResponseBuilder response = Response.ok(data);
// Set the content-disposition header to force the download of the ZIP file
response.header("Content-Disposition", "attachment; filename=images.zip");
// Return the Response object
return response.build();
}
}
```
这个示例代码创建了一个名为 `/images/download` 的端点,它接受一个名为 `urls` 的查询参数,该参数是一个 URL 列表,代表要下载的图片的 URL。该端点会下载所有的图片,并将它们打包成一个名为 `images.zip` 的 ZIP 文件,然后将 ZIP 文件作为响应体发送给客户端。客户端会自动下载 ZIP 文件。
你可以使用类似于以下的 curl 命令来测试该端点:
```
curl -o images.zip "http://localhost:8080/images/download?urls=http://example.com/image1.png&urls=http://example.com/image2.png"
```
该命令会从 `http://example.com/image1.png` 和 `http://example.com/image2.png` 下载两张图片,并将它们打包成一个名为 `images.zip` 的 ZIP 文件,然后将 ZIP 文件保存在当前目录下。
相关推荐
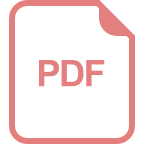














