谱聚类算法python代码
时间: 2023-05-25 12:06:30 浏览: 175
以下是谱聚类算法的Python代码:
```
import numpy as np
from sklearn.cluster import KMeans
from sklearn.neighbors import kneighbors_graph
from scipy.sparse.linalg import eigsh
def spectral_clustering(X, n_clusters=8, n_neighbors=10, gamma=1.0):
"""
Implementation of spectral clustering algorithm
using KMeans and nearest neighbor graphs with RBF kernel.
Parameters
----------
X : array-like or sparse matrix, shape (n_samples, n_features)
Input data.
n_clusters : int, optional, default: 8
The dimension of the projection subspace.
n_neighbors : int, optional, default: 10
The number of nearest neighbors to include in the
constructed k-NN matrix.
gamma : float, optional, default: 1.0
Parameter for RBF kernel.
Returns
-------
y_pred : array-like, shape (n_samples,)
Predicted cluster labels.
"""
# Construct k-nearest neighbor graph with RBF kernel
knn_graph = kneighbors_graph(X, n_neighbors=n_neighbors, mode='connectivity', metric='euclidean')
affinity_matrix = np.exp(-gamma * np.square(knn_graph))
# Get Laplacian matrix
degree_matrix = np.diag(np.sum(affinity_matrix, axis=1))
laplacian_matrix = degree_matrix - affinity_matrix
# Compute eigenvectors of Laplacian matrix
_, eigenvectors = eigsh(laplacian_matrix, k=n_clusters, which='SM')
# Normalize eigenvectors row-wise and use KMeans to get labels
row_norm = np.linalg.norm(eigenvectors, axis=1, ord=2)
normalized_eigenvectors = eigenvectors / row_norm[:, np.newaxis]
kmeans = KMeans(n_clusters=n_clusters)
y_pred = kmeans.fit_predict(normalized_eigenvectors)
return y_pred
```
这个实现使用欧几里得距离来构建k-NN矩阵,并使用RBF内核将其转换为相似性矩阵。它还使用Laplacian矩阵表示图形,并计算Laplacian矩阵的前$n\_clusters$个最小特征向量。这些特征向量被归一化,用于聚类标签预测,由KMeans算法执行。
相关推荐
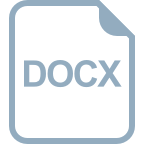









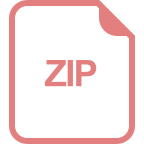
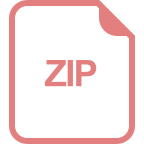
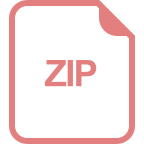