本题要求编写程序,输入10个字符,统计其中英文字母、数字字符和其他字符的个数。 输入格式: 输入为10个字符。最后一个回车表示输入结束,不算在内。 输出格式: 在一行内按照 letter = 英文字母个数, digit = 数字字符个数, other = 其他字符个数 的格式输出。请注意,等号的左右各有一个空格,逗号后有一个空格。用c语言编程
时间: 2024-03-10 14:45:55 浏览: 42
以下是用C语言实现的程序:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char c;
int letter = 0, digit = 0, other = 0;
printf("请输入10个字符:\n");
for (int i = 0; i < 10; i++) {
scanf(" %c", &c);
if (isalpha(c)) {
letter++;
} else if (isdigit(c)) {
digit++;
} else {
other++;
}
}
printf("letter = %d, digit = %d, other = %d\n", letter, digit, other);
return 0;
}
```
程序首先定义了三个变量,分别用于统计英文字母、数字字符和其他字符的个数。然后使用循环输入10个字符,并使用`isalpha()`函数、`isdigit()`函数判断字符的类型,根据不同的类型更新对应变量的值。最后输出统计结果。
需要注意的是,为了避免输入的字符被当做上一次输入的残留字符处理,输入字符时使用了空格跳过前面的空白字符。
相关问题
用C语言写 本题要求编写程序,输入10个字符,统计其中英文字母、数字字符和其他字符的个数。
在C语言中,你可以通过使用标准库函数以及一些基本的数据结构来实现这个功能。下面是一个简单的示例程序:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char input[10];
int alpha_count = 0, digit_count = 0, other_count = 0;
// 输入10个字符
printf("请输入10个字符:");
for (int i = 0; i < 10 && scanf("%c", &input[i]) == 1; i++) {
// 判断字符类型
if (isalpha(input[i])) { // 英文字母
alpha_count++;
} else if (isdigit(input[i])) { // 数字字符
digit_count++;
} else { // 其他字符
other_count++;
}
}
// 检查是否读取了完整的10个字符
if (i == 10) {
printf("统计结果:
- 英文字母: %d\n",
alpha_count);
printf("- 数字字符: %d\n",
digit_count);
printf("- 其他字符: %d\n", other_count);
} else {
printf("输入过长,请输入10个字符。\n");
}
return 0;
}
```
在这个程序中,`scanf`用于从用户那里获取字符,`isalpha`和`isdigit`分别检查字符是否为字母和数字。`ctype.h`头文件包含了这两个函数。最后,我们根据字符类型累加计数并输出结果。
本题要求编写程序,输入10个字符,统计其中英文字母、数字字符和其他字符的个数。
下面是一个 Python 程序的示例:
```python
text = input("请输入10个字符:")
letter_count = 0
digit_count = 0
other_count = 0
for char in text:
if char.isalpha():
letter_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
print("英文字母的个数:", letter_count)
print("数字字符的个数:", digit_count)
print("其他字符的个数:", other_count)
```
该程序首先提示用户输入 10 个字符,然后使用 for 循环遍历每个字符,使用 `isalpha()` 和 `isdigit()` 判断字符是否为英文字母或数字,并统计英文字母、数字字符和其他字符的个数。最后输出统计结果。
阅读全文
相关推荐
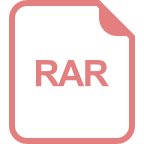
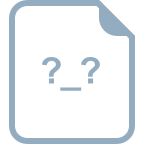
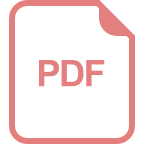













