设置时间片大小(3~6),使用时间片轮转调度算法实现处理机调度
时间: 2024-01-29 07:02:28 浏览: 117
好的,根据您的要求,我来回答问题。
时间片轮转调度算法是一种很常见的调度算法,其基本思想是将所有就绪进程按照先来先服务的原则依次放入一个就绪队列中,并将CPU的时间分成若干个时间片,每个进程分配一个时间片来占用CPU,若一个进程在一个时间片内没有完成,则该进程重新加入就绪队列的末尾,等待下一次调度。
根据您的要求,假设我们设置时间片大小为3~6之间的随机数,具体实现算法如下:
1. 定义一个时间片大小变量time_slice,使用rand()函数生成随机数,然后对其取模3,再加上3,保证时间片大小在3~6之间。
```c
srand(time(NULL)); // 初始化随机数种子
int time_slice = rand() % 3 + 3; // 生成3~6之间的随机数
```
2. 定义一个就绪队列,将所有就绪进程按照先来先服务的原则依次放入队列中。
```c
typedef struct PCB {
int id; // 进程ID
int status; // 进程状态
int run_time; // 运行时间
int priority; // 优先级
struct PCB *next; // 指向下一个进程的指针
} PCB;
PCB *ready_queue = NULL; // 就绪队列头指针
void add_process_to_ready_queue(PCB *new_process) {
if(!ready_queue) { // 就绪队列为空,直接将新进程加入队列
ready_queue = new_process;
} else { // 就绪队列不为空,将新进程加入队列末尾
PCB *p = ready_queue;
while(p->next) {
p = p->next;
}
p->next = new_process;
}
}
```
3. 在主函数中,定义一个循环,每次从就绪队列中取出一个进程,让其运行一个时间片,若该进程运行时间小于等于时间片,则直接结束该进程,并打印该进程的信息;若该进程运行时间大于时间片,则将该进程重新加入就绪队列的末尾,并更新该进程的运行时间。
```c
int main() {
srand(time(NULL)); // 初始化随机数种子
int time_slice = rand() % 3 + 3; // 生成3~6之间的随机数
// 创建8个进程并加入就绪队列
for(int i = 1; i <= 8; i++) {
PCB *new_process = (PCB *)malloc(sizeof(PCB));
new_process->id = i;
new_process->status = 1; // 就绪状态
new_process->run_time = rand() % 15 + 1; // 随机生成1~15之间的运行时间
new_process->priority = 0;
new_process->next = NULL;
add_process_to_ready_queue(new_process);
}
// 时间片轮转调度
while(ready_queue) { // 就绪队列不为空
PCB *running_process = ready_queue; // 取出队列头进程
ready_queue = ready_queue->next;
running_process->next = NULL;
if(running_process->run_time <= time_slice) { // 进程运行时间小于等于时间片
printf("进程%d运行结束,运行时间为%d\n", running_process->id, running_process->run_time);
free(running_process);
} else { // 进程运行时间大于时间片
running_process->run_time -= time_slice;
add_process_to_ready_queue(running_process);
}
}
return 0;
}
```
以上就是使用时间片轮转调度算法实现处理机调度的基本实现方法。希望能够对您有所帮助。
阅读全文
相关推荐
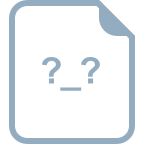
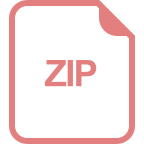
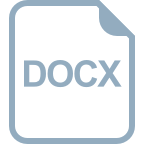
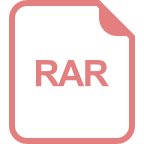
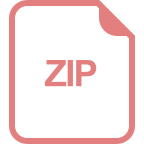
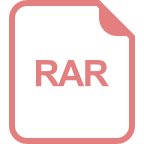
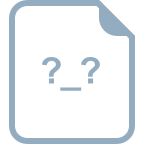
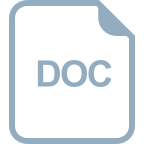
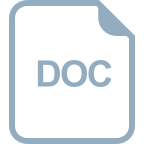
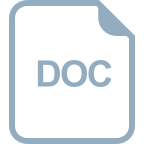
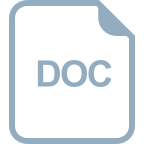
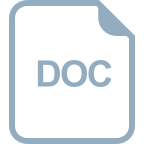