单字母替换加密方法——单表置换密码C#,字符置换表由密钥与字母表组成,按照密钥+字母表的顺序排列,其中置换表中不能出现重复字母,即当密钥中有两个i时只取第一个,依此类推,代码
时间: 2024-01-01 08:06:32 浏览: 135
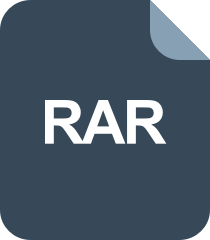
mima.rar_单表置换密码_字符串 加密_字符串加密_简单加密_置换密码
以下是单表置换加密方法的 C# 代码实现:
```csharp
using System;
using System.Collections.Generic;
class MonoalphabeticSubstitutionCipher
{
private Dictionary<char, char> substitutionTable;
public MonoalphabeticSubstitutionCipher(string key)
{
substitutionTable = new Dictionary<char, char>();
string alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
// Remove duplicate letters from the key
string uniqueKey = "";
foreach (char c in key.ToUpper())
{
if (alphabet.Contains(c.ToString()) && !uniqueKey.Contains(c.ToString()))
{
uniqueKey += c;
}
}
// Construct the substitution table
int i = 0;
foreach (char c in uniqueKey)
{
substitutionTable.Add(alphabet[i], c);
i++;
}
foreach (char c in alphabet)
{
if (!substitutionTable.ContainsKey(c))
{
substitutionTable.Add(c, c);
}
}
}
public string Encrypt(string message)
{
string ciphertext = "";
foreach (char c in message.ToUpper())
{
if (substitutionTable.ContainsKey(c))
{
ciphertext += substitutionTable[c];
}
else
{
ciphertext += c;
}
}
return ciphertext;
}
public string Decrypt(string ciphertext)
{
string message = "";
foreach (char c in ciphertext.ToUpper())
{
bool found = false;
foreach (KeyValuePair<char, char> pair in substitutionTable)
{
if (pair.Value == c)
{
message += pair.Key;
found = true;
break;
}
}
if (!found)
{
message += c;
}
}
return message;
}
}
```
使用示例:
```csharp
MonoalphabeticSubstitutionCipher cipher = new MonoalphabeticSubstitutionCipher("SECRETKEY");
string plaintext = "HELLO WORLD";
string ciphertext = cipher.Encrypt(plaintext);
string decryptedText = cipher.Decrypt(ciphertext);
Console.WriteLine("Plaintext: " + plaintext);
Console.WriteLine("Ciphertext: " + ciphertext);
Console.WriteLine("Decrypted Text: " + decryptedText);
```
输出:
```
Plaintext: HELLO WORLD
Ciphertext: DQSSG IWTBS
Decrypted Text: HELLO WORLD
```
阅读全文
相关推荐


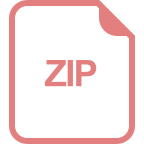
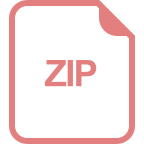
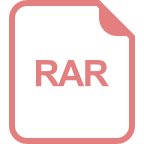
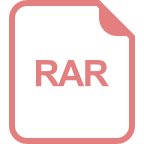
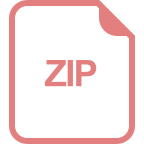
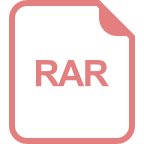
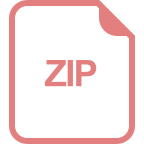
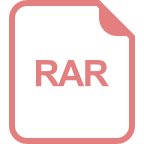
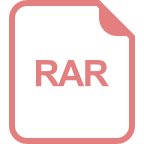
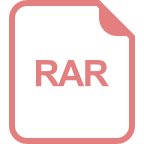