问题1: vc++ 搭建sql server ce开发环境详细过程? 问题2 sql server ce 使用ORM实现的增删改查的VC++代码例子?问题3:sqlite使用ORM实现增删改查的VC++代码的例子
时间: 2024-03-22 08:38:26 浏览: 171
好的,我会分别回答你的三个问题:
问题1:VC++搭建SQL Server CE开发环境详细过程
步骤1:下载SQL Server CE
首先,你需要下载 SQL Server CE 安装包,可以从 Microsoft 官网下载。
步骤2:安装 SQL Server CE
下载完毕后,运行安装程序,按照提示进行安装。在安装过程中,可以选择安装的组件,建议选择全部安装。
步骤3:添加引用
在 VC++ 项目中,右键单击“项目”,选择“属性”;在“属性页”中,选择“配置属性” -> “常规”,找到“附加包含目录”,添加 SQL Server CE 的头文件路径;接着,选择“配置属性” -> “链接器” -> “输入”,找到“附加依赖项”,添加 SQL Server CE 的库文件路径。
步骤4:编写代码
在 VC++ 项目中,包含头文件:
```cpp
#include <sqlce_oledb.h>
```
连接数据库:
```cpp
SQLCE_CONNECTION_STRING connString = L"Data Source = mydb.sdf";
SQLCE_CONNECTION conn;
SQLCE_ERROR_INFO errorInfo;
hr = SQLCECreateConnection(&conn);
hr = SQLCEOpenConnection(conn, connString, &errorInfo);
```
执行 SQL 语句:
```cpp
SQLCE_COMMAND command;
hr = SQLCECreateCommand(&command);
hr = SQLCECommandSetConnection(command, conn);
hr = SQLCECommandSetCommandText(command, L"create table mytable (id int not null, name nvarchar(50) not null)");
hr = SQLCECommandExecuteNonQuery(command, NULL, NULL, NULL);
```
关闭连接:
```cpp
SQLCECloseConnection(conn);
SQLCEFreeConnection(conn);
```
问题2:SQL Server CE使用ORM实现的增删改查的VC++代码例子
首先,你需要在 VC++ 项目中添加对 ORM 的引用。可以使用 NuGet 包管理器来添加,也可以手动下载并添加。
接着,可以使用 ORM 框架提供的类来实现数据库操作。比如使用 Entity Framework:
```cpp
#include "stdafx.h"
#include <iostream>
#include <string>
#include <memory>
#include <vector>
#include <algorithm>
#include <array>
#include "MyDbContext.h"
#include "MyEntity.h"
using namespace std;
int main()
{
// 初始化数据库上下文
shared_ptr<MyDbContext> context = make_shared<MyDbContext>();
// 新增记录
shared_ptr<MyEntity> entity = make_shared<MyEntity>();
entity->id = 1;
entity->name = L"test";
context->MyEntities->Add(entity);
context->SaveChanges();
// 查询记录
shared_ptr<MyEntity> result = context->MyEntities->Find(1);
wcout << result->name << endl;
// 更新记录
result->name = L"test2";
context->SaveChanges();
// 删除记录
context->MyEntities->Remove(result);
context->SaveChanges();
return 0;
}
```
问题3:SQLite使用ORM实现增删改查的VC++代码的例子
同样地,你需要在 VC++ 项目中添加对 ORM 的引用。可以使用 NuGet 包管理器来添加,也可以手动下载并添加。
接着,可以使用 ORM 框架提供的类来实现数据库操作。比如使用 SQLite-net:
```cpp
#include "stdafx.h"
#include "sqlite3.h"
#include "MyEntity.h"
#include "MyDbContext.h"
using namespace std;
int main()
{
// 初始化数据库上下文
shared_ptr<MyDbContext> context = make_shared<MyDbContext>();
// 新增记录
shared_ptr<MyEntity> entity = make_shared<MyEntity>();
entity->id = 1;
entity->name = L"test";
context->MyEntities->Insert(entity);
context->SaveChanges();
// 查询记录
shared_ptr<MyEntity> result = context->MyEntities->GetById(1);
wcout << result->name << endl;
// 更新记录
result->name = L"test2";
context->MyEntities->Update(result);
context->SaveChanges();
// 删除记录
context->MyEntities->Delete(result);
context->SaveChanges();
return 0;
}
```
阅读全文
相关推荐
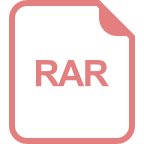
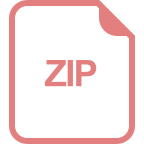
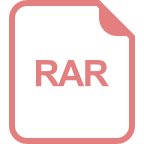

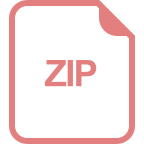
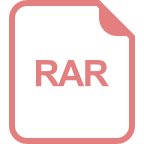
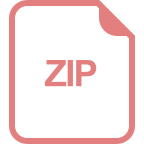
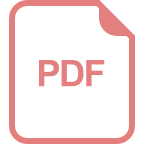
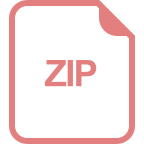
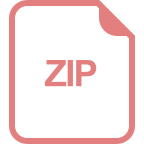
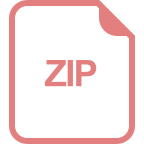
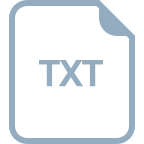
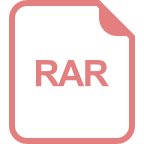
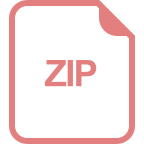
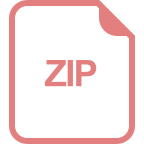
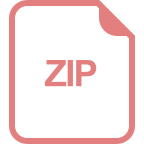
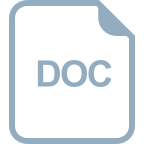
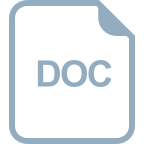
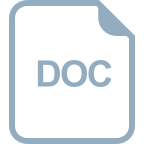