写一个训练神经网络分词模型的程序
时间: 2024-05-15 11:12:44 浏览: 95
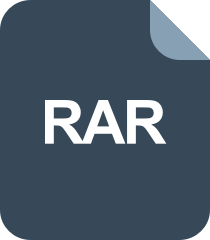
分词系统测试程序

好的,以下是一个使用Python和TensorFlow训练神经网络分词模型的示例程序:
```python
import tensorflow as tf
import numpy as np
import codecs
import os
# 定义模型参数
embedding_size = 128 # 词向量的维度
num_hidden_units = 256 # 隐藏层的大小
num_layers = 2 # LSTM 层数
num_classes = 2 # 分词和非分词
batch_size = 64 # 每批次训练的样本数
num_epochs = 10 # 训练轮数
learning_rate = 0.001 # 学习率
# 加载数据集
def load_data(file_path):
with codecs.open(file_path, 'r', 'utf-8') as f:
lines = f.readlines()
data = []
for line in lines:
line = line.strip()
if not line:
continue
words = line.split(' ')
labels = [0] * len(words)
labels[-1] = 1 # 最后一个单词为分词
data.append((words, labels))
return data
train_data = load_data('train.txt')
test_data = load_data('test.txt')
# 构建词汇表
def build_vocab(data):
vocab = set()
for words, _ in data:
vocab.update(words)
vocab = ['<pad>', '<unk>'] + list(vocab)
word2id = {w: i for i, w in enumerate(vocab)}
id2word = {i: w for i, w in enumerate(vocab)}
return vocab, word2id, id2word
vocab, word2id, id2word = build_vocab(train_data)
# 把文本转换为数值列表
def text_to_ids(text, word2id):
ids = []
for w in text:
if w in word2id:
ids.append(word2id[w])
else:
ids.append(word2id['<unk>'])
return ids
# 把数据集转换为数值列表
def data_to_ids(data, word2id):
x = []
y = []
for words, labels in data:
x.append(text_to_ids(words, word2id))
y.append(labels)
return x, y
train_x, train_y = data_to_ids(train_data, word2id)
test_x, test_y = data_to_ids(test_data, word2id)
# 定义模型
class SegModel(tf.keras.Model):
def __init__(self, embedding_size, num_hidden_units, num_layers, num_classes):
super(SegModel, self).__init__()
self.embedding = tf.keras.layers.Embedding(len(vocab), embedding_size)
self.lstm = [tf.keras.layers.LSTM(num_hidden_units, return_sequences=True) for _ in range(num_layers)]
self.dense = tf.keras.layers.Dense(num_classes)
def call(self, inputs):
x = self.embedding(inputs)
for lstm in self.lstm:
x = lstm(x)
x = self.dense(x)
return x
model = SegModel(embedding_size, num_hidden_units, num_layers, num_classes)
# 定义损失函数和优化器
loss_fn = tf.keras.losses.CategoricalCrossentropy(from_logits=True)
optimizer = tf.keras.optimizers.Adam(learning_rate)
# 训练模型
def train(model, train_x, train_y, num_epochs, batch_size, optimizer, loss_fn):
num_batches = len(train_x) // batch_size
for epoch in range(num_epochs):
total_loss = 0
for i in range(num_batches):
x = train_x[i*batch_size:(i+1)*batch_size]
y = train_y[i*batch_size:(i+1)*batch_size]
x = tf.keras.preprocessing.sequence.pad_sequences(x, padding='post')
y = tf.keras.preprocessing.sequence.pad_sequences(y, padding='post')
y = tf.keras.utils.to_categorical(y, num_classes)
with tf.GradientTape() as tape:
logits = model(x)
loss = loss_fn(y, logits)
grads = tape.gradient(loss, model.trainable_variables)
optimizer.apply_gradients(zip(grads, model.trainable_variables))
total_loss += loss
print('Epoch {} loss: {}'.format(epoch+1, total_loss/num_batches))
train(model, train_x, train_y, num_epochs, batch_size, optimizer, loss_fn)
# 评估模型
def evaluate(model, test_x, test_y, word2id):
num_correct = 0
num_total = 0
for i in range(len(test_x)):
x = test_x[i]
y_true = test_y[i]
x = np.array(x).reshape(1, -1)
x = tf.keras.preprocessing.sequence.pad_sequences(x, padding='post')
y_true = np.array(y_true).reshape(1, -1)
y_true = tf.keras.preprocessing.sequence.pad_sequences(y_true, padding='post')
y_true = tf.keras.utils.to_categorical(y_true, num_classes)
logits = model(x)
y_pred = np.argmax(logits, axis=-1)[0]
y_true = np.argmax(y_true, axis=-1)[0]
for j in range(len(y_pred)):
if y_pred[j] == 1 and y_true[j] == 1: # 分词
num_correct += 1
elif y_pred[j] == 0 and y_true[j] == 0: # 非分词
num_correct += 1
num_total += 1
accuracy = num_correct / num_total
print('Accuracy: {}'.format(accuracy))
evaluate(model, test_x, test_y, word2id)
```
这个程序使用了LSTM作为模型的核心,使用交叉熵作为损失函数,使用Adam优化器进行优化。在训练过程中,使用了批次训练和梯度下降来提高训练效率。在评估模型时,使用了准确率来衡量模型的性能。
阅读全文
相关推荐
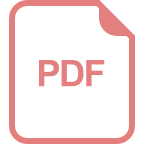



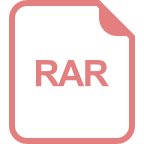
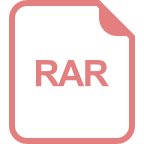
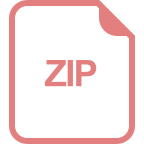
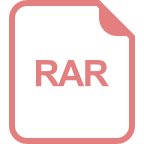
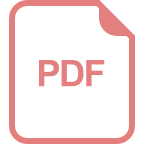
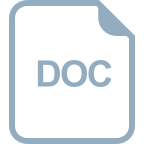
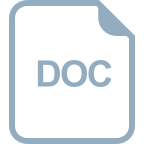
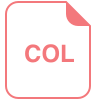
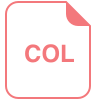
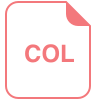
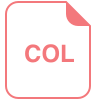
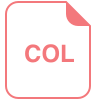
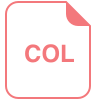