写一段C++代码 要求如下 :定义一个Shape类,派生出Circle,Rectangel等类,并且: 定义area(求面积)、平移(按x轴平移一定距离)、show(显示信息)等函数(以虚函数的形式,体会虚函数、动态绑定、多态的含义)。
时间: 2023-07-16 10:16:06 浏览: 81
以下是符合要求的C++代码:
```c++
#include <iostream>
using namespace std;
class Shape {
public:
virtual double area() = 0; // 纯虚函数,需要在子类中实现
virtual void translate(double x) = 0; // 纯虚函数,需要在子类中实现
virtual void show() = 0; // 纯虚函数,需要在子类中实现
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double area() { return 3.14 * radius * radius; } // 根据圆的公式计算面积
void translate(double x) { cout << "The circle is translated by " << x << " units on the x-axis." << endl; } // 按x轴平移一定距离
void show() { cout << "This is a circle with radius " << radius << "." << endl; } // 显示信息
};
class Rectangle : public Shape {
private:
double length;
double width;
public:
Rectangle(double l, double w) : length(l), width(w) {}
double area() { return length * width; } // 根据矩形的公式计算面积
void translate(double x) { cout << "The rectangle is translated by " << x << " units on the x-axis." << endl; } // 按x轴平移一定距离
void show() { cout << "This is a rectangle with length " << length << " and width " << width << "." << endl; } // 显示信息
};
int main() {
Shape* s; // 定义Shape类的指针
Circle c(2.5);
Rectangle r(3, 4);
s = &c;
s->show();
cout << "The area is " << s->area() << "." << endl;
s->translate(1.5);
s = &r;
s->show();
cout << "The area is " << s->area() << "." << endl;
s->translate(2.5);
return 0;
}
```
在该代码中,我们定义了一个Shape类,它是一个抽象类,包含了纯虚函数area、translate和show。我们从Shape类派生出了Circle和Rectangle类,并重写了父类的纯虚函数。在main函数中,我们定义了一个Shape类型的指针s,并分别将它指向Circle类的对象c和Rectangle类的对象r,通过s调用了各自的函数area、translate和show。由于这些函数是虚函数,因此程序在运行时会根据实际指向的对象,动态绑定并调用子类中的函数,实现了多态的效果。
阅读全文
相关推荐
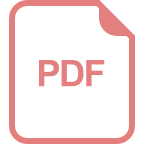
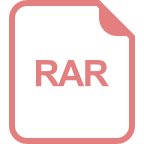
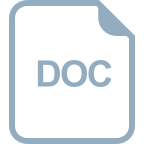
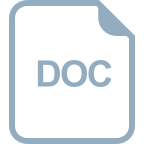











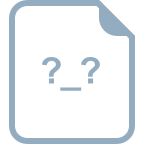
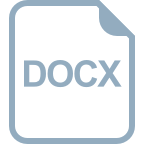