举一个使用torchsummary中summary函数的例子
时间: 2023-07-29 08:12:33 浏览: 48
好的,下面是一个使用torchsummary中summary函数的例子:
```python
import torch
import torch.nn as nn
from torchsummary import summary
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.conv1 = nn.Conv2d(3, 32, kernel_size=3, stride=1, padding=1)
self.bn1 = nn.BatchNorm2d(32)
self.relu1 = nn.ReLU(inplace=True)
self.pool1 = nn.MaxPool2d(kernel_size=2, stride=2)
self.conv2 = nn.Conv2d(32, 64, kernel_size=3, stride=1, padding=1)
self.bn2 = nn.BatchNorm2d(64)
self.relu2 = nn.ReLU(inplace=True)
self.pool2 = nn.MaxPool2d(kernel_size=2, stride=2)
self.conv3 = nn.Conv2d(64, 128, kernel_size=3, stride=1, padding=1)
self.bn3 = nn.BatchNorm2d(128)
self.relu3 = nn.ReLU(inplace=True)
self.pool3 = nn.MaxPool2d(kernel_size=2, stride=2)
self.fc1 = nn.Linear(4 * 4 * 128, 1024)
self.relu4 = nn.ReLU(inplace=True)
self.dropout1 = nn.Dropout2d(p=0.5)
self.fc2 = nn.Linear(1024, 10)
def forward(self, x):
x = self.conv1(x)
x = self.bn1(x)
x = self.relu1(x)
x = self.pool1(x)
x = self.conv2(x)
x = self.bn2(x)
x = self.relu2(x)
x = self.pool2(x)
x = self.conv3(x)
x = self.bn3(x)
x = self.relu3(x)
x = self.pool3(x)
x = x.view(-1, 4 * 4 * 128)
x = self.fc1(x)
x = self.relu4(x)
x = self.dropout1(x)
x = self.fc2(x)
return x
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
model = Net().to(device)
summary(model, input_size=(3, 32, 32))
```
在这个例子中,我们定义了一个名为Net的类,它继承了nn.Module类,并实现了一个卷积神经网络。我们使用torchsummary中的summary函数来打印网络的结构和参数数量。在调用summary函数时,我们将模型和输入的大小作为参数传递给它。运行程序后,我们可以看到输出的结构和参数数量,如下所示:
```
----------------------------------------------------------------
Layer (type) Output Shape Param #
================================================================
Conv2d-1 [-1, 32, 32, 32] 896
BatchNorm2d-2 [-1, 32, 32, 32] 64
ReLU-3 [-1, 32, 32, 32] 0
MaxPool2d-4 [-1, 32, 16, 16] 0
Conv2d-5 [-1, 64, 16, 16] 18,496
BatchNorm2d-6 [-1, 64, 16, 16] 128
ReLU-7 [-1, 64, 16, 16] 0
MaxPool2d-8 [-1, 64, 8, 8] 0
Conv2d-9 [-1, 128, 8, 8] 73,856
BatchNorm2d-10 [-1, 128, 8, 8] 256
ReLU-11 [-1, 128, 8, 8] 0
MaxPool2d-12 [-1, 128, 4, 4] 0
Linear-13 [-1, 1024] 2,097,152
ReLU-14 [-1, 1024] 0
Dropout2d-15 [-1, 1024] 0
Linear-16 [-1, 10] 10,250
================================================================
Total params: 2,200,098
Trainable params: 2,200,098
Non-trainable params: 0
----------------------------------------------------------------
```
相关推荐
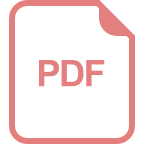
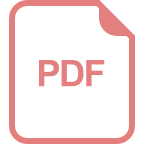
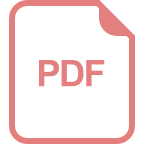














