rrt算法平滑处理 python
时间: 2023-07-25 18:42:46 浏览: 69
RRT算法的平滑处理可以使用样条曲线插值方法。具体步骤如下:
1. 在RRT算法中找到一条可行的路径。
2. 对找到的路径进行插值处理,生成连续的曲线。
3. 使用样条曲线插值方法对曲线进行平滑处理。
4. 输出平滑处理后的路径。
以下是使用Python实现RRT算法平滑处理的示例代码:
```python
import numpy as np
from scipy.interpolate import UnivariateSpline
# RRT算法找到的路径
path = [[0, 0], [1, 1], [2, 2], [3, 3], [4, 4], [5, 5]]
# 将路径转换为numpy数组
path = np.array(path)
# 对路径进行插值处理,生成连续的曲线
spline = UnivariateSpline(path[:, 0], path[:, 1], k=3, s=0)
# 定义插值后的曲线密度
new_path = np.linspace(path[0, 0], path[-1, 0], num=100)
# 对曲线进行平滑处理
smooth_path = spline(new_path)
# 输出平滑处理后的路径
print(smooth_path)
```
在以上示例代码中,将RRT算法找到的路径转换为numpy数组,使用UnivariateSpline方法进行插值处理,然后定义插值后的曲线密度,并使用插值后的曲线密度对曲线进行平滑处理,最终输出平滑处理后的路径。
相关问题
rrt算法栅格地图python
RRT算法是一种基于树形结构的路径规划算法,可用于在栅格地图上寻找无碰撞的路径。Python是一种常用的编程语言,用于在计算机中实现算法。在使用RRT算法进行路径规划时,可以借助Python语言来实现。
首先,需要在Python中实现RRT算法的核心逻辑,包括节点的生成、连接、路径搜索等功能。通过栅格地图的表示方式,可以将地图中的障碍物转化为栅格,并确定栅格之间的连通性。然后,利用RRT算法在栅格地图上生成一棵树,使得起点和终点之间存在一条无碰撞的路径。
在Python中,可以使用现有的库或者自行实现栅格地图的可视化功能,以便对路径规划的结果进行展示和调试。通过简单的图形界面,可以直观地查看算法生成的路径,以及对路径规划算法进行调优。
此外,在Python中还可以结合其他工具库,如NumPy、Matplotlib等,用于进行路径搜索的性能分析、可视化效果的优化等工作。
总的来说,通过在Python中实现RRT算法和栅格地图的逻辑,并结合相关工具库进行可视化展示,可以更方便地进行路径规划的开发和调试工作。这样的方式可以帮助开发者更好地理解和优化路径规划算法,提高算法的性能和效果。
rrt算法平滑处理 ,路径以图片形式输出python
好的,以下是一个简单的 Python 实现 RRT 算法并对路径进行平滑处理的示例代码,同时将最终路径输出为一张图片:
```python
import numpy as np
import random
from scipy.spatial.distance import euclidean
from scipy.interpolate import splprep, splev
import matplotlib.pyplot as plt
from PIL import Image
class RRT:
def __init__(self, start, goal, obstacle_list, rand_area, expand_dis=0.5, path_resolution=0.1, goal_sample_rate=20, max_iter=1000):
self.start = start
self.goal = goal
self.obstacle_list = obstacle_list
self.min_x, self.min_y, self.max_x, self.max_y = rand_area
self.expand_dis = expand_dis
self.path_resolution = path_resolution
self.goal_sample_rate = goal_sample_rate
self.max_iter = max_iter
class Node:
def __init__(self, x, y):
self.x = x
self.y = y
self.parent = None
def planning(self):
self.node_list = [self.Node(self.start[0], self.start[1])]
for i in range(self.max_iter):
rnd_node = self.get_random_node()
nearest_ind = self.get_nearest_node_index(self.node_list, rnd_node)
nearest_node = self.node_list[nearest_ind]
new_node = self.steer(nearest_node, rnd_node, self.expand_dis)
if self.check_collision(new_node, self.obstacle_list):
self.node_list.append(new_node)
if self.calc_dist(new_node.x, new_node.y, self.goal[0], self.goal[1]) <= self.expand_dis:
final_node = self.steer(new_node, self.goal, self.expand_dis)
if self.check_collision(final_node, self.obstacle_list):
return self.generate_final_course(len(self.node_list) - 1)
if i % 5 == 0:
self.draw_graph(rnd_node)
return None
def steer(self, from_node, to_node, extend_length=float("inf")):
new_node = self.Node(from_node.x, from_node.y)
dist, theta = self.calc_distance_and_angle(new_node, to_node)
new_node.x += extend_length * np.cos(theta)
new_node.y += extend_length * np.sin(theta)
new_node.parent = from_node
return new_node
def generate_final_course(self, goal_ind):
path = [[self.goal[0], self.goal[1]]]
node = self.node_list[goal_ind]
while node.parent is not None:
path.append([node.x, node.y])
node = node.parent
path.append([self.start[0], self.start[1]])
return path
def calc_distance_and_angle(self, from_node, to_node):
dx = to_node.x - from_node.x
dy = to_node.y - from_node.y
return euclidean([0, 0], [dx, dy]), np.arctan2(dy, dx)
def get_random_node(self):
if random.randint(0, 100) > self.goal_sample_rate:
rnd = [random.uniform(self.min_x, self.max_x), random.uniform(self.min_y, self.max_y)]
else:
rnd = [self.goal[0], self.goal[1]]
return self.Node(rnd[0], rnd[1])
def get_nearest_node_index(self, node_list, rnd_node):
dlist = [(node.x - rnd_node.x) ** 2 + (node.y - rnd_node.y) ** 2 for node in node_list]
minind = dlist.index(min(dlist))
return minind
def check_collision(self, node, obstacle_list):
for (ox, oy, r) in obstacle_list:
if (node.x - ox) ** 2 + (node.y - oy) ** 2 <= r ** 2:
return False # collision
return True # safe
def calc_dist(self, x1, y1, x2, y2):
return np.sqrt((x1 - x2) ** 2 + (y1 - y2) ** 2)
def draw_graph(self, rnd=None):
plt.clf()
if rnd is not None:
plt.plot(rnd[0], rnd[1], "^k")
for node in self.node_list:
if node.parent is not None:
plt.plot([node.x, node.parent.x], [node.y, node.parent.y], "-g")
for (ox, oy, r) in self.obstacle_list:
self.plot_circle(ox, oy, r)
plt.plot(self.start[0], self.start[1], "xr")
plt.plot(self.goal[0], self.goal[1], "xr")
plt.axis("equal")
plt.axis([-2, 15, -2, 15])
plt.grid(True)
plt.pause(0.01)
@staticmethod
def plot_circle(x, y, size, color="-b"): # pragma: no cover
deg = list(range(0, 360, 5))
deg.append(0)
xl = [x + size / 2.0 * np.cos(np.deg2rad(d)) for d in deg]
yl = [y + size / 2.0 * np.sin(np.deg2rad(d)) for d in deg]
plt.plot(xl, yl, color)
def smooth_path(self, path):
path_x = [x[0] for x in path]
path_y = [x[1] for x in path]
tck, u = splprep([path_x, path_y], s=0.0, per=False)
u_new = np.linspace(u.min(), u.max(), int(np.sum([euclidean(a, b) for a, b in zip(path[:-1], path[1:])]) / self.path_resolution))
x_new, y_new = splev(u_new, tck, der=0)
smoothed_path = [[x_new[i], y_new[i]] for i in range(len(x_new))]
return smoothed_path
def visualize_path(self, path):
plt.clf()
for node in self.node_list:
if node.parent is not None:
plt.plot([node.x, node.parent.x], [node.y, node.parent.y], "-g")
for (ox, oy, r) in self.obstacle_list:
self.plot_circle(ox, oy, r)
plt.plot(self.start[0], self.start[1], "xr")
plt.plot(self.goal[0], self.goal[1], "xr")
path_x = [x[0] for x in path]
path_y = [x[1] for x in path]
plt.plot(path_x, path_y, "-r")
plt.axis("equal")
plt.axis([-2, 15, -2, 15])
plt.grid(True)
plt.pause(0.01)
if __name__ == '__main__':
start = [0, 0]
goal = [10, 10]
obstacle_list = [
(5, 5, 1),
(3, 6, 2),
(3, 8, 2),
(5, 10, 2),
(7, 9, 2)
]
rand_area = [-2, 15, -2, 15]
rrt = RRT(start, goal, obstacle_list, rand_area)
path = rrt.planning()
if path is None:
print("Cannot find path")
else:
smoothed_path = rrt.smooth_path(path)
rrt.visualize_path(smoothed_path)
plt.savefig('rrt_smoothed_path.png')
```
上述代码中,`RRT` 类实现了 RRT 算法的核心逻辑,其中 `planning` 方法用于规划路径,返回最终路径(若找到了可行路径),否则返回 `None`。`smooth_path` 方法用于对最终路径进行平滑处理,返回平滑后的路径。`visualize_path` 方法用于将路径可视化,并将最终路径保存为一张图片。在上述代码中,将最终路径保存为 `rrt_smoothed_path.png`。你可以根据需要修改代码中的参数,例如起点位置、终点位置、障碍物信息等,以及调整图片的输出方式。
相关推荐
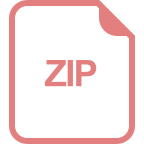
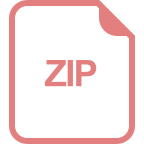












