写一个javaFx中关于AnimationTimer的简单程序
时间: 2023-07-15 13:11:23 浏览: 57
好的,以下是一个简单的 JavaFX 程序,使用 AnimationTimer 来实现动画效果:
```java
import javafx.animation.AnimationTimer;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class AnimationTimerDemo extends Application {
// 画布的宽度和高度
private static final int WIDTH = 400;
private static final int HEIGHT = 400;
// 小球的位置和速度
private double x = 0;
private double y = 0;
private double vx = 2;
private double vy = 3;
@Override
public void start(Stage primaryStage) throws Exception {
// 创建画布
Canvas canvas = new Canvas(WIDTH, HEIGHT);
GraphicsContext gc = canvas.getGraphicsContext2D();
// 创建动画计时器
AnimationTimer timer = new AnimationTimer() {
@Override
public void handle(long now) {
// 每一帧更新小球的位置
update();
// 清空画布
gc.clearRect(0, 0, WIDTH, HEIGHT);
// 绘制小球
gc.setFill(Color.RED);
gc.fillOval(x, y, 20, 20);
}
};
// 启动动画计时器
timer.start();
// 创建场景和舞台
StackPane root = new StackPane(canvas);
Scene scene = new Scene(root, WIDTH, HEIGHT);
primaryStage.setScene(scene);
primaryStage.setTitle("Animation Timer Demo");
primaryStage.show();
}
// 更新小球的位置
private void update() {
x += vx;
y += vy;
if (x < 0 || x > WIDTH - 20) {
vx = -vx;
}
if (y < 0 || y > HEIGHT - 20) {
vy = -vy;
}
}
public static void main(String[] args) {
launch(args);
}
}
```
这个程序会在窗口中绘制一个红色的小球,并让它在画布内弹来弹去。动画效果由 AnimationTimer 来实现。每一帧动画,AnimationTimer 的 handle 方法都会被调用一次,我们在这个方法中更新小球的位置和绘制小球。注意,这个方法的参数 now 是当前时间戳,但我们没有用到它。
相关推荐
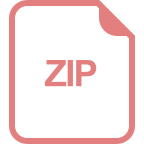
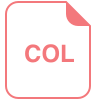
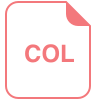
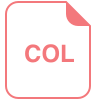
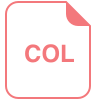
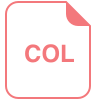









