def __str__(self): """ Model prints with number of trainable parameters """ model_parameters = filter(lambda p: p.requires_grad, self.parameters()) params = sum([np.prod(p.size()) for p in model_parameters]) return super().__str__() + f'\nTrainable parameters : {params}'
时间: 2024-04-29 07:19:34 浏览: 25
这段代码实现了模型对象的打印功能,输出模型的名称和可训练参数的数量。
具体来说,`filter(lambda p: p.requires_grad, self.parameters())` 是获取当前模型中所有需要梯度更新的参数列表。`p.requires_grad` 表示该参数是否需要梯度更新,即是否是可训练的参数。`self.parameters()` 是获取模型中所有的参数列表。
接下来,`sum([np.prod(p.size()) for p in model_parameters])` 计算可训练参数的总数量,它遍历模型中所有需要梯度更新的参数,对每个参数的形状进行 `np.prod(p.size())` 的计算,即将参数形状中的每个元素相乘,得到该参数的总大小。最后将所有参数的总大小相加,即可得到模型的可训练参数数量。
最后,`super().__str__()` 会调用父类的 `__str__` 方法,返回模型的名称和结构。`f'\nTrainable parameters : {params}'` 则是将可训练参数数量添加到模型的名称和结构之后,作为最终的字符串返回。
相关问题
def __getitem__(self, index):
As an AI language model, I don't have access to the full context of your code. However, based on the method name "__getitem__", it is likely that this method is being used to implement the indexing behavior for a custom object or data structure.
In Python, the "__getitem__" method is a special method that allows objects to be indexed using square brackets. For example, if you have a custom list-like object, you could implement "__getitem__" to allow indexing like this:
my_list = MyCustomList([1, 2, 3])
print(my_list[0]) # prints 1
The "__getitem__" method takes an index as its argument and should return the value at that index. If the index is invalid, such as being out of range, the method should raise an IndexError.
Here is an example implementation of "__getitem__" for a custom list-like object:
class MyCustomList:
def __init__(self, items):
self.items = items
def __getitem__(self, index):
if index < 0 or index >= len(self.items):
raise IndexError("Index out of range")
return self.items[index]
if user_input == "q":
If the user enters "q", it usually means they want to quit or exit the program. So, you can include some code to handle this input and gracefully exit the program. For example:
```
if user_input.lower() == "q":
print("Goodbye!")
exit()
```
This code checks if the user input is "q", regardless of upper or lower case letters, and if it is, prints a goodbye message and exits the program using the `exit()` function.
相关推荐
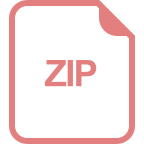
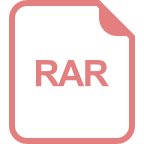












