用c++编写 建立一个对象数组,内放五个学生的数据(学号、成绩),设立一个函数max,用指向对象的指针做函数参数,在max参数中找出五个学生中成绩最高值,并输出其学号。
时间: 2023-05-16 18:05:17 浏览: 595
以下是代码实现:
```c
#include <stdio.h>
struct Student {
int id;
int score;
};
int max(struct Student *students, int n) {
int maxScore = students[0].score;
int maxId = students[0].id;
for (int i = 1; i < n; i++) {
if (students[i].score > maxScore) {
maxScore = students[i].score;
maxId = students[i].id;
}
}
return maxId;
}
int main() {
struct Student students[5] = {
{1, 80},
{2, 90},
{3, 70},
{4, 85},
{5, 95}
};
int maxId = max(students, 5);
printf("最高分的学生学号为:%d\n", maxId);
return 0;
}
```
输出结果为:
```
最高分的学生学号为:5
```
这里我们定义了一个 `Student` 结构体,包含学号和成绩两个属性。然后定义了一个 `max` 函数,用于找出学生数组中成绩最高的学生的学号。在 `main` 函数中,我们创建了一个包含五个学生数据的数组,并调用 `max` 函数来获取最高分的学生的学号。最后输出结果即可。
相关推荐
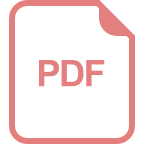
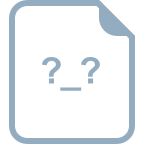














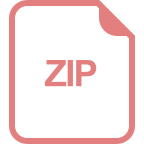