Error in pmatch(use, c("all.obs", "complete.obs", "pairwise.complete.obs", : object 'use' not found怎么解决
时间: 2023-02-07 07:14:18 浏览: 70
这个错误的意思是在 pmatch 函数中找不到名为 "use" 的对象。要解决这个错误,你需要确保在当前的工作环境中存在一个名为 "use" 的对象。你可以使用 `ls()` 函数查看当前工作环境中有哪些对象,或者使用 `exists("use")` 函数来检查是否存在名为 "use" 的对象。如果 "use" 对象不存在,你需要创建它或者检查代码中是否有其他错误导致 "use" 对象没有被正确创建。
相关问题
详细说明regex.h 中函数的使用方法
regex.h 头文件中定义了一些用于处理正则表达式的函数。下面是它们的详细说明:
1. int regcomp(regex_t *preg, const char *regex, int cflags)
该函数用于编译一个正则表达式,将其转换为一个 regex_t 类型的对象。它的参数说明如下:
- preg:指向一个 regex_t 类型的对象的指针,用于保存编译后的正则表达式。
- regex:要编译的正则表达式。
- cflags:编译选项,可以是以下值的按位或运算结果:
- REG_EXTENDED:使用扩展正则表达式语法。
- REG_ICASE:不区分大小写。
- REG_NOSUB:不返回子匹配。
- REG_NEWLINE:换行符也是一个有效字符。
返回值为 0 表示编译成功,否则表示编译失败。如果编译失败,可以通过调用 regerror 函数获取错误信息。
2. size_t regerror(int errcode, const regex_t *preg, char *errbuf, size_t errbuf_size)
该函数用于获取编译或执行正则表达式时的错误信息。它的参数说明如下:
- errcode:错误码,通常是 regcomp 或 regexec 函数返回的值。
- preg:指向编译后的正则表达式对象的指针。
- errbuf:用于存储错误信息的缓冲区。
- errbuf_size:缓冲区的大小。
返回值为存储在缓冲区中的错误信息的字节数。
3. int regexec(const regex_t *preg, const char *string, size_t nmatch, regmatch_t pmatch[], int eflags)
该函数用于执行一个正则表达式。它的参数说明如下:
- preg:指向编译后的正则表达式对象的指针。
- string:要匹配的字符串。
- nmatch:pmatch 数组的大小,即最多能存储多少个子匹配结果。
- pmatch:一个 regmatch_t 类型的数组,用于存储子匹配结果的位置信息。
- eflags:执行选项,可以是以下值的按位或运算结果:
- REG_NOTBOL:字符串不是行首。
- REG_NOTEOL:字符串不是行尾。
- REG_STARTEND:指定匹配的起始位置和结束位置。
返回值为 0 表示匹配成功,否则表示匹配失败。如果匹配失败,可以通过调用 regerror 函数获取错误信息。
4. void regfree(regex_t *preg)
该函数用于释放一个编译后的正则表达式对象占用的内存。
使用以上函数需要注意的是,正则表达式的语法是非常复杂的,不同的实现对于语法的支持也有所差异。因此,在编写正则表达式时需要仔细阅读相关文档,并且进行充分的测试验证,以确保正则表达式的正确性和实用性。
用c++正则表达式提取http://192.168.1.29:8060/api/v1.0/trans/dst_url中的IP、端口、路径内容
### 回答1:
根据您提供的信息,我理解您需要用 C 语言正则表达式提取 HTTP 请求中的 IP、端口和路径。下面是一些示例代码,希望能帮助您实现所需的功能。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <regex.h>
int main() {
char *pattern = "http://([0-9]+\\.[0-9]+\\.[0-9]+\\.[0-9]+):([0-9]+)/(.*)";
char *string = "http://192.168.1.29:8060/api/v1.0/trans/dst_url";
regex_t reg;
regmatch_t pmatch[4];
int status = regcomp(®, pattern, REG_EXTENDED);
if (status != 0) {
printf("Regex compilation failed\n");
return 1;
}
status = regexec(®, string, 4, pmatch, 0);
if (status == REG_NOMATCH) {
printf("Regex match failed\n");
return 1;
}
char ip[16], port[6], path[256];
memset(ip, 0, sizeof(ip));
memset(port, 0, sizeof(port));
memset(path, 0, sizeof(path));
strncpy(ip, string + pmatch[1].rm_so, pmatch[1].rm_eo - pmatch[1].rm_so);
strncpy(port, string + pmatch[2].rm_so, pmatch[2].rm_eo - pmatch[2].rm_so);
strncpy(path, string + pmatch[3].rm_so, pmatch[3].rm_eo - pmatch[3].rm_so);
printf("IP: %s\n", ip);
printf("Port: %s\n", port);
printf("Path: %s\n", path);
regfree(®);
return 0;
}
```
此代码将打印:
```
IP: 192.168.1.29
Port: 8060
Path: api/v1.0/trans/dst_url
```
希望这可以回答您的问题。如果您有任何其他问题,请告诉我。
### 回答2:
使用C语言的正则表达式库可以提取出URL中的IP、端口和路径内容。下面是一个可能的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <regex.h>
int main() {
char *url = "http://192.168.1.29:8060/api/v1.0/trans/dst_url";
regex_t regex;
regmatch_t matches[10];
char *pattern = "http://([0-9.]+):([0-9]+)/([a-zA-Z0-9_/]+)";
// 编译正则表达式
if(regcomp(®ex, pattern, REG_EXTENDED) != 0) {
printf("正则表达式编译失败\n");
return -1;
}
// 执行正则匹配
int result = regexec(®ex, url, 10, matches, 0);
if(result != 0) {
printf("未找到匹配的内容\n");
return -1;
}
// 提取匹配到的IP、端口和路径内容
char *ip = malloc(matches[1].rm_eo - matches[1].rm_so + 1);
char *port = malloc(matches[2].rm_eo - matches[2].rm_so + 1);
char *path = malloc(matches[3].rm_eo - matches[3].rm_so + 1);
strncpy(ip, url + matches[1].rm_so, matches[1].rm_eo - matches[1].rm_so);
strncpy(port, url + matches[2].rm_so, matches[2].rm_eo - matches[2].rm_so);
strncpy(path, url + matches[3].rm_so, matches[3].rm_eo - matches[3].rm_so);
ip[matches[1].rm_eo - matches[1].rm_so] = '\0';
port[matches[2].rm_eo - matches[2].rm_so] = '\0';
path[matches[3].rm_eo - matches[3].rm_so] = '\0';
printf("IP: %s\n", ip);
printf("端口: %s\n", port);
printf("路径: %s\n", path);
// 释放内存并清理正则表达式对象
regfree(®ex);
free(ip);
free(port);
free(path);
return 0;
}
```
以上代码中使用了正则表达式 `http://([0-9.]+):([0-9]+)/([a-zA-Z0-9_/]+)` 来匹配URL中的IP、端口和路径。其中 `([0-9.]+)` 匹配IP地址部分, `([0-9]+)` 匹配端口部分, `([a-zA-Z0-9_/]+)` 匹配路径部分。最后通过 `regexec` 和 `regmatch_t` 结构体来获取匹配到的内容,并将其拷贝到新的内存空间中。最后打印出IP、端口和路径内容,并释放相应的内存。
相关推荐
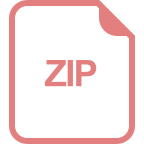
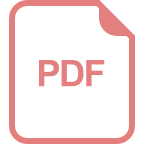
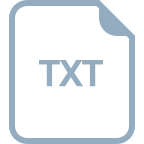













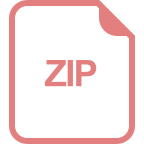