tensorflow 如何将y_train转化为数组
时间: 2024-05-07 11:18:09 浏览: 63
在 TensorFlow 中,可以使用 `numpy` 库将 `y_train` 转换为数组。具体实现方法如下:
```python
import numpy as np
# 假设 y_train 是一个列表
y_train = [1, 2, 3, 4, 5]
# 将 y_train 转换为数组
y_train = np.array(y_train)
# 打印数组
print(y_train)
```
运行以上代码后,可以看到 `y_train` 已经被转换为了数组,并输出了其内容:
```
[1 2 3 4 5]
```
相关问题
(1)训练一个模型能够识别天气图片,将训练好的参数保存。 (2)写一段代码,main.py, 假设1.jpg是一副天气图片,执行 main.py 1.jpg,打印出该图片的天气类别。
由于缺乏具体的数据集和模型,以下是一个简单的示例,使用了手写数字数据集和简单的神经网络模型。
训练模型并保存参数的代码:
```python
import tensorflow as tf
from tensorflow.keras.datasets import mnist
# 加载手写数字数据集
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# 数据预处理
x_train, x_test = x_train / 255.0, x_test / 255.0
# 定义模型
model = tf.keras.models.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dropout(0.2),
tf.keras.layers.Dense(10)
])
# 编译模型
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
# 训练模型
model.fit(x_train, y_train, epochs=5)
# 保存模型参数
model.save_weights('model.h5')
```
以上代码训练了一个简单的神经网络模型,使用手写数字数据集进行训练,并将训练好的参数保存到文件 `model.h5` 中。
下面是识别天气图片的代码 `main.py`:
```python
import sys
import tensorflow as tf
import numpy as np
from PIL import Image
# 加载模型
model = tf.keras.models.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dropout(0.2),
tf.keras.layers.Dense(10)
])
# 加载模型参数
model.load_weights('model.h5')
# 定义类别标签
class_names = ['0', '1', '2', '3', '4', '5', '6', '7', '8', '9']
# 加载图片
img_path = sys.argv[1]
img = Image.open(img_path).convert('L') # 转灰度图
img = img.resize((28, 28)) # 调整大小
img_arr = np.array(img) / 255.0 # 转化为数组并归一化
img_arr = np.expand_dims(img_arr, axis=0) # 增加一个维度
# 预测类别
predictions = model.predict(img_arr)
predicted_class = np.argmax(predictions[0])
predicted_label = class_names[predicted_class]
# 打印结果
print('This weather image is:', predicted_label)
```
以上代码加载训练好的模型参数,并读取命令行参数中指定的图片文件。使用 PIL 库将图片转化为灰度图,并调整大小为 28x28,然后转化为数组并归一化。最后使用模型进行预测,并输出预测结果。
阅读全文
相关推荐
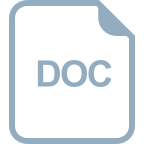
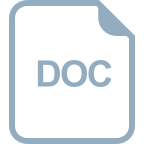
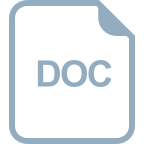
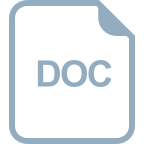
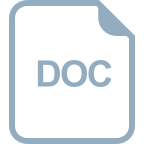
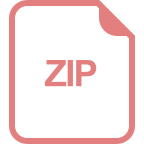
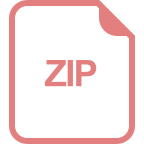