trajectory_cleaning 函数
时间: 2024-01-21 20:04:36 浏览: 27
trajectory_cleaning 函数通常用于处理轨迹数据中的噪声和异常值,使其更加平滑和准确。一般情况下,它会执行以下操作:
1. 移除异常值:将超出给定阈值的点视为异常值,并将它们从轨迹中删除。
2. 平滑轨迹:使用平滑算法(如滑动平均或卡尔曼滤波)来减少噪声和抖动。
3. 修复断点:将断点处的轨迹片段重新连接起来,以消除轨迹的不连续性。
这些操作的目的是提高轨迹数据的准确性和可用性,以便更好地支持各种应用程序,如地图导航、交通监控和物流管理等。
相关问题
trajectory_cleaning 函数Matlab代码
以下是一个可能的 trajectory_cleaning 函数的 Matlab 代码示例:
```matlab
function cleaned_traj = trajectory_cleaning(traj, threshold)
% TRAJECTORY_CLEANING Remove noisy points from a trajectory.
% cleaned_traj = TRAJECTORY_CLEANING(traj, threshold) returns a cleaned
% trajectory by removing points that are too far away from their neighbors.
% The input trajectory is a matrix with each row representing a point in
% 2D space (x, y coordinates). The threshold parameter specifies the
% maximum distance allowed between a point and its neighbor.
% Initialize cleaned trajectory with first point
cleaned_traj = traj(1,:);
% Loop through each point in the trajectory
for i = 2:size(traj, 1)
% Calculate distance between current point and previous point
dist = norm(traj(i,:) - cleaned_traj(end,:));
% If distance is smaller than threshold, add current point to cleaned
% trajectory
if dist <= threshold
cleaned_traj = [cleaned_traj; traj(i,:)];
end
end
end
```
这个函数接受两个参数:原始轨迹 traj 和阈值 threshold。函数返回一个清理过的轨迹 cleaned_traj,其中距离过远的点已经被移除。函数从第二个点开始循环,计算当前点与前一个点的距离。如果距离小于阈值,则将当前点添加到清理后的轨迹中。
trajectory_cleaning 函数代码
下面是一个简单的 trajectory_cleaning 函数的 Python 代码示例:
```python
def trajectory_cleaning(trajectory, min_distance=0.1):
"""
:param trajectory: 二维轨迹数组,格式为 [[x1, y1], [x2, y2], ...]
:param min_distance: 相邻两个点之间的最小距离,小于该距离的点会被合并为一个点
:return: 清理后的轨迹数组
"""
cleaned_trajectory = [trajectory[0]] # 清理后的轨迹数组
last_point = trajectory[0] # 上一个点
for point in trajectory[1:]:
distance = ((point[0] - last_point[0]) ** 2 + (point[1] - last_point[1]) ** 2) ** 0.5 # 计算距离
if distance >= min_distance:
cleaned_trajectory.append(point) # 距离足够远,添加该点
last_point = point # 更新上一个点为当前点
return cleaned_trajectory
```
该函数接受一个二维轨迹数组作为输入,该数组的格式为 `[[x1, y1], [x2, y2], ...]`,表示轨迹上的每个点的坐标。函数还接受一个可选参数 `min_distance`,表示相邻两个点之间的最小距离,小于该距离的点会被合并为一个点。
在函数内部,我们首先将轨迹数组的第一个点添加到清理后的轨迹数组中。然后,我们遍历轨迹数组中的每个点。对于每个点,我们计算它与上一个点之间的距离。如果该距离大于等于 `min_distance`,我们将该点添加到清理后的轨迹数组中,并将上一个点更新为当前点。
最后,我们返回清理后的轨迹数组。
相关推荐
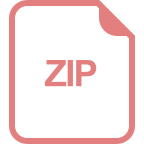
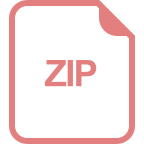












