基于粤嵌LinuxGEC6818开发板实现电子相册的c语言程序
时间: 2024-03-22 08:41:03 浏览: 151
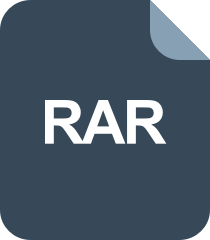
基于GEC6818开发板的电子相册程序
好的,以下是一个基于粤嵌LinuxGEC6818开发板实现电子相册的C语言程序的简单示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <linux/fb.h>
#include <sys/mman.h>
int main()
{
// 打开并映射framebuffer设备文件
int fbfd = open("/dev/fb0", O_RDWR);
if (fbfd == -1) {
printf("Error: cannot open framebuffer device.\n");
exit(1);
}
struct fb_var_screeninfo vinfo;
struct fb_fix_screeninfo finfo;
if (ioctl(fbfd, FBIOGET_FSCREENINFO, &finfo) == -1) {
printf("Error: reading fixed information.\n");
exit(2);
}
if (ioctl(fbfd, FBIOGET_VSCREENINFO, &vinfo) == -1) {
printf("Error: reading variable information.\n");
exit(3);
}
int screensize = vinfo.xres * vinfo.yres * vinfo.bits_per_pixel / 8;
char *fbp = (char *)mmap(0, screensize, PROT_READ | PROT_WRITE, MAP_SHARED, fbfd, 0);
if ((int)fbp == -1) {
printf("Error: failed to mmap framebuffer device to memory.\n");
exit(4);
}
// 读取图片并绘制到framebuffer上
int imgfd = open("test.jpg", O_RDONLY);
if (imgfd == -1) {
printf("Error: cannot open image file.\n");
exit(5);
}
char *imgp = (char *)malloc(screensize);
if (read(imgfd, imgp, screensize) == -1) {
printf("Error: failed to read image file.\n");
exit(6);
}
for (int y = 0; y < vinfo.yres; y++) {
for (int x = 0; x < vinfo.xres; x++) {
int location = (x + vinfo.xoffset) * (vinfo.bits_per_pixel / 8) +
(y + vinfo.yoffset) * finfo.line_length;
*(fbp + location) = *(imgp + location);
*(fbp + location + 1) = *(imgp + location + 1);
*(fbp + location + 2) = *(imgp + location + 2);
*(fbp + location + 3) = *(imgp + location + 3);
}
}
// 关闭文件描述符和解除内存映射
close(imgfd);
munmap(fbp, screensize);
close(fbfd);
return 0;
}
```
这个程序实现了打开并映射framebuffer设备文件,读取图片并绘制到framebuffer上的功能。您可以根据需要对程序进行修改和扩展,实现更完整的电子相册功能。
阅读全文
相关推荐

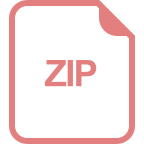
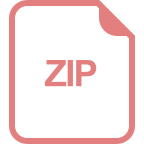
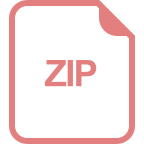
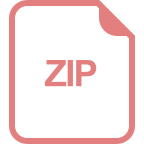
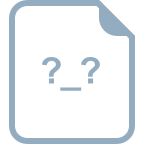
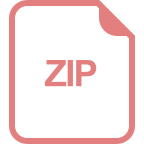
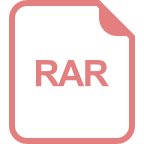
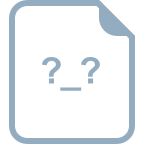
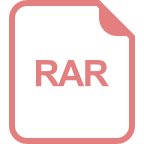
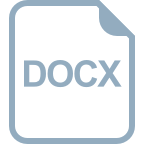
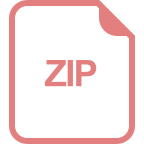