/* 创建一个矩形类,矩形的边平行于坐标轴。矩形的属性用其左下角坐标、长度和宽度描述。 (1) 从键盘输入两个矩形参数(注意输入提示信息),创建两个矩形对象, 判断两个矩形是否相交?求出相交部分的面积。 (2) 从键盘再输入或随机产生3个矩形对象,对输入的所有5个矩形按面积由小到大排序, 然后依次显示各个矩形的左下角坐标、长、宽、面积。 */c++
时间: 2024-03-02 16:49:27 浏览: 29
以下是矩形类的实现代码:
```c++
#include <iostream>
using namespace std;
class Rectangle {
private:
double x, y; // 矩形左下角坐标
double length, width; // 矩形长度和宽度
public:
Rectangle(double x = 0, double y = 0, double length = 0, double width = 0) {
this->x = x;
this->y = y;
this->length = length;
this->width = width;
}
double getX() const { return x; }
double getY() const { return y; }
double getLength() const { return length; }
double getWidth() const { return width; }
double getArea() const { return length * width; }
bool isIntersect(const Rectangle& r) const {
double x1 = max(x, r.x);
double y1 = max(y, r.y);
double x2 = min(x + length, r.x + r.length);
double y2 = min(y + width, r.y + r.width);
return x1 < x2 && y1 < y2;
}
Rectangle intersect(const Rectangle& r) const {
double x1 = max(x, r.x);
double y1 = max(y, r.y);
double x2 = min(x + length, r.x + r.length);
double y2 = min(y + width, r.y + r.width);
return Rectangle(x1, y1, x2 - x1, y2 - y1);
}
};
int main() {
Rectangle r1, r2;
cout << "请输入矩形1的左下角坐标、长度和宽度:";
cin >> r1;
cout << "请输入矩形2的左下角坐标、长度和宽度:";
cin >> r2;
if (r1.isIntersect(r2)) {
Rectangle r3 = r1.intersect(r2);
cout << "两个矩形相交,相交部分的面积为:" << r3.getArea() << endl;
} else {
cout << "两个矩形不相交。" << endl;
}
Rectangle r[5];
for (int i = 0; i < 3; i++) {
cout << "请输入矩形" << i + 3 << "的左下角坐标、长度和宽度:";
cin >> r[i + 2];
}
sort(r, r + 5, [](const Rectangle& a, const Rectangle& b) {
return a.getArea() < b.getArea();
});
for (int i = 0; i < 5; i++) {
cout << "矩形" << i + 1 << "的左下角坐标为:" << r[i].getX() << ", " << r[i].getY() << endl;
cout << "矩形" << i + 1 << "的长度为:" << r[i].getLength() << endl;
cout << "矩形" << i + 1 << "的宽度为:" << r[i].getWidth() << endl;
cout << "矩形" << i + 1 << "的面积为:" << r[i].getArea() << endl;
}
return 0;
}
```
这个程序中,我们定义了一个`Rectangle`类来表示矩形,其中包括矩形左下角的坐标、长度和宽度等属性,以及计算矩形面积、判断两个矩形是否相交以及求出相交部分的面积等方法。
在`main`函数中,我们首先从键盘输入两个矩形的参数,创建两个`Rectangle`对象,然后调用`isIntersect`方法判断两个矩形是否相交,如果相交,再调用`intersect`方法求出相交部分的面积并输出。
接着,我们再从键盘输入或随机产生3个矩形对象,将这5个矩形按照面积从小到大排序,并依次输出每个矩形的左下角坐标、长度、宽度和面积等信息。其中,我们使用了`sort`函数和lambda表达式来实现对矩形的排序,`sort`函数默认从小到大排序,因此我们只需要定义一个lambda表达式,使其返回矩形面积的大小关系即可。
相关推荐
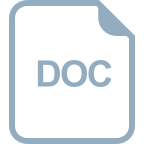
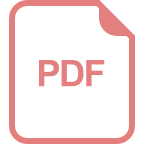













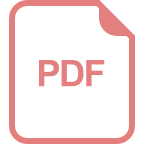
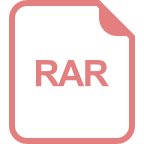