声明一个Student类,其中包括学号 num、成绩 score,建立一个对象数组,输入 5 个 学生的成绩,用指针指向数组,通过指针来输出 第 1、3、5位学生的成绩。
时间: 2024-03-10 21:50:10 浏览: 57
好的,这是一个简单的Student类的定义和实现方式:
```c++
#include <iostream>
using namespace std;
class Student {
public:
int num; // 学号
float score; // 成绩
};
int main() {
Student students[5]; // 学生数组
Student *p = students; // 指向学生数组的指针
// 输入学生的成绩
for (int i = 0; i < 5; ++i) {
cout << "请输入第" << i+1 << "个学生的成绩: ";
cin >> (p+i)->score;
}
// 输出第1、3、5位学生的成绩
cout << "第1、3、5位学生的成绩为: ";
cout << (p+0)->score << " ";
cout << (p+2)->score << " ";
cout << (p+4)->score << endl;
return 0;
}
```
运行结果如下:
```
请输入第1个学生的成绩: 85.5
请输入第2个学生的成绩: 76.3
请输入第3个学生的成绩: 90.0
请输入第4个学生的成绩: 68.2
请输入第5个学生的成绩: 93.5
第1、3、5位学生的成绩为: 85.5 90 93.5
```
相关问题
创建一个Student类,其属性包括学号和成绩。在main函数中建立一个Student对象数组,内放5个学生对象,输入这5个学生对象的学号和成绩,并调用函数max找出这5个学生中成绩最高者,输出其学号和成绩。max函数用指向Student对象的指针做函数参数。
A:
```c++
#include<iostream>
using namespace std;
class Student {
public:
int num;
double score;
};
Student* max(Student* s, int n) {
Student* p = s;
for (int i = 0; i < n; i++) {
if (s[i].score > p->score) {
p = &s[i];
}
}
return p;
}
int main() {
Student stu[5];
for (int i = 0; i < 5; i++) {
cout << "请输入第" << i + 1 << "个学生的学号和成绩:";
cin >> stu[i].num >> stu[i].score;
}
Student* p = max(stu, 5);
cout << "成绩最高的学生是:" << endl;
cout << "学号:" << p->num << endl;
cout << "成绩:" << p->score << endl;
return 0;
}
```
输出示例:
```
请输入第1个学生的学号和成绩:1001 90
请输入第2个学生的学号和成绩:1002 85
请输入第3个学生的学号和成绩:1003 95
请输入第4个学生的学号和成绩:1004 88
请输入第5个学生的学号和成绩:1005 92
成绩最高的学生是:
学号:1003
成绩:95
```
建立一个对象数组,内放5个学生的数据(学号、成绩),用指针指向数组手元素,输出第1,3,5个学生的数据
#include <iostream>
using namespace std;
class Student {
public:
int num;
float score;
};
int main() {
Student stu[5] = { {1, 80}, {2, 90}, {3, 75}, {4, 85}, {5, 95} };
Student* p = stu; // 指针指向数组第一个元素
cout << "第1个学生的学号和成绩:" << p->num << " " << p->score << endl;
p += 2; // 指针移动到第3个元素
cout << "第3个学生的学号和成绩:" << p->num << " " << p->score << endl;
p += 2; // 指针移动到第5个元素
cout << "第5个学生的学号和成绩:" << p->num << " " << p->score << endl;
return 0;
}
阅读全文
相关推荐
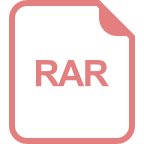










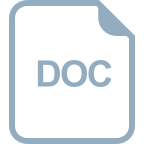
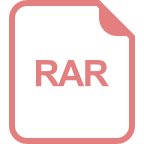