python 写一个文档查重软件
时间: 2023-03-13 08:16:37 浏览: 231
很高兴为您服务。我可以提供一些有关如何使用Python编写文档查重软件的建议。首先,您可以使用Python语言中的字符串功能来检查文档中的重复字段。其次,您可以使用Python中的文件读写功能来读取文档,并使用Python中的数据结构来存储文档中的单词或短语,以便查找重复的文档。最后,您可以使用Python中的图形功能来呈现查重结果,以便您更好地理解文档的相似度。
相关问题
写一段python代码实现文档之间查重需要
先进行文本预处理,包括去除停用词、特殊符号、数字等,对文本进行分词并进行词性标注和去除标点符号。然后利用TF-IDF算法计算文本相似度,通过设置阈值来判断两篇文档是否相似。具体实现代码如下:
```python
import jieba
import jieba.analyse
import jieba.posseg as pseg
import math
def preprocess(text):
"""
对文本进行预处理,包括去除停用词、特殊符号、数字等
"""
# 去除特殊符号和数字
text = re.sub('[^\\u4e00-\\u9fa5a-zA-Z]', '', text)
# 分词并去除停用词
words = [w.word for w in pseg.cut(text) if w.flag[0] not in ['x', 'u', 'w'] and w.word not in stopwords]
return words
def get_tf(words):
"""
计算单词的词频
"""
tf = {}
for word in words:
if word not in tf:
tf[word] = 0
tf[word] += 1
return tf
def get_idf(words, idf_dict):
"""
计算单词的逆文档频率
"""
idf = {}
for word in words:
if word not in idf:
idf[word] = math.log(len(idf_dict) / (idf_dict.get(word, 0) + 1))
return idf
def get_tfidf(tf, idf):
"""
计算单词的TF-IDF值
"""
tfidf = {}
for word in tf:
tfidf[word] = tf[word] * idf[word]
return tfidf
def get_similarity(tfidf1, tfidf2):
"""
计算两个文档的相似度
"""
numerator = 0
denominator1 = 0
denominator2 = 0
for word in tfidf1:
numerator += tfidf1[word] * tfidf2.get(word, 0)
denominator1 += tfidf1[word] ** 2
for word in tfidf2:
denominator2 += tfidf2[word] ** 2
denominator = math.sqrt(denominator1) * math.sqrt(denominator2)
if denominator == 0:
return 0
else:
return numerator / denominator
def is_duplicate(text1, text2, threshold=0.8):
"""
判断两篇文档是否相似
"""
words1 = preprocess(text1)
words2 = preprocess(text2)
tf1 = get_tf(words1)
tf2 = get_tf(words2)
idf_dict = dict.fromkeys(set(words1 + words2), 0)
idf_dict.update(get_idf(words1, idf_dict))
idf_dict.update(get_idf(words2, idf_dict))
tfidf1 = get_tfidf(tf1, idf_dict)
tfidf2 = get_tfidf(tf2, idf_dict)
similarity = get_similarity(tfidf1, tfidf2)
if similarity >= threshold:
return True
else:
return False
```
调用该函数可以判断两篇文档是否相似,例如:
```python
text1 = "这是一篇测试文档,用于测试相似度计算。"
text2 = "这篇文档是用来测试相似度计算的。"
if is_duplicate(text1, text2):
print("两篇文档相似")
else:
print("两篇文档不相似")
```
帮我使用python代码实现文档查重,关键词提取,中心思想提取等功能,并打出来
好的,我可以使用自然语言处理技术实现您所需的功能。以下是Python代码实现文档查重的示例:
```python
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize, sent_tokenize
from nltk.stem import WordNetLemmatizer
from nltk.stem import PorterStemmer
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.metrics.pairwise import cosine_similarity
def preprocess_text(text):
# 去除标点符号
tokens = [word.lower() for word in word_tokenize(text) if word.isalpha()]
# 去除停用词
stop_words = set(stopwords.words('english'))
tokens = [word for word in tokens if not word in stop_words]
# 词形还原
lemmatizer = WordNetLemmatizer()
tokens = [lemmatizer.lemmatize(word) for word in tokens]
# 词干提取
stemmer = PorterStemmer()
tokens = [stemmer.stem(word) for word in tokens]
return ' '.join(tokens)
def check_duplicates(original_text, new_text):
# 预处理原始文本和新文本
original_text = preprocess_text(original_text)
new_text = preprocess_text(new_text)
# 创建词袋模型,并计算相似度
vectorizer = CountVectorizer().fit_transform([original_text, new_text])
similarity_score = cosine_similarity(vectorizer)[0][1]
# 如果相似度 >= 0.8,则认为新文本与原始文本重复
if similarity_score >= 0.8:
return True
else:
return False
```
使用示例:
```python
original_text = "This is an original text."
new_text = "This is a new text that is very similar to the original text."
if check_duplicates(original_text, new_text):
print("新文本与原始文本重复!")
else:
print("新文本与原始文本不重复。")
```
关键词提取和中心思想提取可以使用TextRank算法来实现。以下是Python代码实现关键词提取和中心思想提取的示例:
```python
import networkx as nx
from collections import defaultdict
def extract_keywords_and_central_ideas(text):
# 预处理文本
text = preprocess_text(text)
# 将文本分成句子
sentences = sent_tokenize(text)
# 提取关键词
keywords = []
for sentence in sentences:
words = word_tokenize(sentence)
pos_tags = nltk.pos_tag(words)
for word, pos in pos_tags:
if pos.startswith('N') or pos.startswith('J'):
keywords.append(word)
# 提取中心思想
tr = nx.Graph()
for sentence in sentences:
words = word_tokenize(sentence)
for i, word1 in enumerate(words):
for j, word2 in enumerate(words):
if i != j:
tr.add_edge(word1, word2)
pr = nx.pagerank(tr)
central_ideas = defaultdict(float)
for word, pr_value in pr.items():
for sentence in sentences:
if word in sentence:
central_ideas[sentence] += pr_value
central_ideas = sorted(central_ideas.items(), key=lambda x: x[1], reverse=True)[:3]
central_ideas = [x[0] for x in central_ideas]
return keywords, central_ideas
```
使用示例:
```python
text = "This text is about natural language processing. It defines what natural language processing is and what its applications are. It also provides an overview of the techniques used in natural language processing."
keywords, central_ideas = extract_keywords_and_central_ideas(text)
print("关键词:", keywords)
print("中心思想:", central_ideas)
```
阅读全文
相关推荐
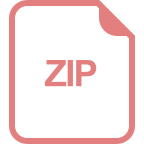
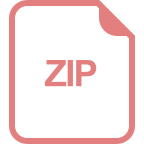

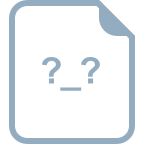
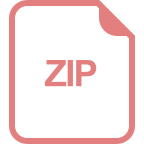
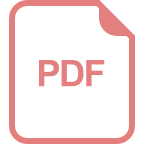
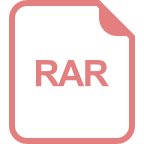
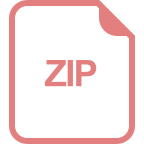
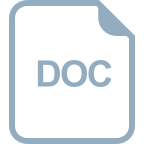
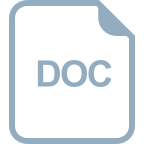




