c++实现控制台图书管理系统
时间: 2023-06-29 14:12:18 浏览: 56
好的,下面是一个简单的控制台图书管理系统的C++实现:
```c++
#include<iostream>
#include<fstream>
#include<cstring>
#include<cstdlib>
#include<cstdio>
#include<iomanip>
using namespace std;
const int maxn = 1000;
struct book{
char id[10];
char name[20];
char author[20];
char publisher[30];
char date[20];
int quantity;
double price;
}books[maxn];
int n = 0;
void menu();
void show();
void add();
void search();
void remove();
void modify();
void sort();
void save();
void load();
int main(){
load(); // 加载文件中的数据
menu();
save(); // 保存数据到文件中
return 0;
}
void menu(){
while(1){
system("cls");
cout << "=======================================" << endl;
cout << " 图书管理系统 v1.0" << endl;
cout << "=======================================" << endl;
cout << " 1. 显示图书" << endl;
cout << " 2. 添加图书" << endl;
cout << " 3. 查找图书" << endl;
cout << " 4. 删除图书" << endl;
cout << " 5. 修改图书" << endl;
cout << " 6. 排序图书" << endl;
cout << " 0. 退出系统" << endl;
cout << "=======================================" << endl;
cout << "请选择操作:";
int choice;
cin >> choice;
switch(choice){
case 0:
return;
case 1:
show();
break;
case 2:
add();
break;
case 3:
search();
break;
case 4:
remove();
break;
case 5:
modify();
break;
case 6:
sort();
break;
default:
cout << "输入有误,请重新输入!" << endl;
system("pause");
}
}
}
void show(){
if(n == 0){
cout << "没有图书记录!" << endl;
system("pause");
return;
}
cout << setw(10) << "编号" << setw(20) << "书名" << setw(20) << "作者" << setw(30) << "出版社" << setw(20) << "出版日期" << setw(10) << "数量" << setw(10) << "价格" << endl;
for(int i = 0; i < n; i++){
cout << setw(10) << books[i].id << setw(20) << books[i].name << setw(20) << books[i].author << setw(30) << books[i].publisher << setw(20) << books[i].date << setw(10) << books[i].quantity << setw(10) << books[i].price << endl;
}
system("pause");
}
void add(){
cout << "请输入图书编号:";
cin >> books[n].id;
cout << "请输入图书名称:";
cin >> books[n].name;
cout << "请输入图书作者:";
cin >> books[n].author;
cout << "请输入图书出版社:";
cin >> books[n].publisher;
cout << "请输入图书出版日期:";
cin >> books[n].date;
cout << "请输入图书数量:";
cin >> books[n].quantity;
cout << "请输入图书价格:";
cin >> books[n].price;
n++;
cout << "添加成功!" << endl;
system("pause");
}
void search(){
cout << "请输入要查找的图书名称:";
char name[20];
cin >> name;
for(int i = 0; i < n; i++){
if(strcmp(books[i].name, name) == 0){
cout << setw(10) << "编号" << setw(20) << "书名" << setw(20) << "作者" << setw(30) << "出版社" << setw(20) << "出版日期" << setw(10) << "数量" << setw(10) << "价格" << endl;
cout << setw(10) << books[i].id << setw(20) << books[i].name << setw(20) << books[i].author << setw(30) << books[i].publisher << setw(20) << books[i].date << setw(10) << books[i].quantity << setw(10) << books[i].price << endl;
system("pause");
return;
}
}
cout << "没有找到该图书!" << endl;
system("pause");
}
void remove(){
cout << "请输入要删除的图书名称:";
char name[20];
cin >> name;
for(int i = 0; i < n; i++){
if(strcmp(books[i].name, name) == 0){
for(int j = i; j < n - 1; j++){
books[j] = books[j + 1];
}
n--;
cout << "删除成功!" << endl;
system("pause");
return;
}
}
cout << "没有找到该图书!" << endl;
system("pause");
}
void modify(){
cout << "请输入要修改的图书名称:";
char name[20];
cin >> name;
for(int i = 0; i < n; i++){
if(strcmp(books[i].name, name) == 0){
cout << "请输入图书编号:";
cin >> books[i].id;
cout << "请输入图书名称:";
cin >> books[i].name;
cout << "请输入图书作者:";
cin >> books[i].author;
cout << "请输入图书出版社:";
cin >> books[i].publisher;
cout << "请输入图书出版日期:";
cin >> books[i].date;
cout << "请输入图书数量:";
cin >> books[i].quantity;
cout << "请输入图书价格:";
cin >> books[i].price;
cout << "修改成功!" << endl;
system("pause");
return;
}
}
cout << "没有找到该图书!" << endl;
system("pause");
}
void sort(){
cout << "请选择排序方式:" << endl;
cout << "1.按编号排序" << endl;
cout << "2.按书名排序" << endl;
cout << "3.按作者排序" << endl;
cout << "4.按出版社排序" << endl;
cout << "5.按出版日期排序" << endl;
int choice;
cin >> choice;
switch(choice){
case 1:
for(int i = 0; i < n - 1; i++){
for(int j = 0; j < n - i - 1; j++){
if(strcmp(books[j].id, books[j + 1].id) > 0){
book tmp = books[j];
books[j] = books[j + 1];
books[j + 1] = tmp;
}
}
}
cout << "按编号排序成功!" << endl;
system("pause");
break;
case 2:
for(int i = 0; i < n - 1; i++){
for(int j = 0; j < n - i - 1; j++){
if(strcmp(books[j].name, books[j + 1].name) > 0){
book tmp = books[j];
books[j] = books[j + 1];
books[j + 1] = tmp;
}
}
}
cout << "按书名排序成功!" << endl;
system("pause");
break;
case 3:
for(int i = 0; i < n - 1; i++){
for(int j = 0; j < n - i - 1; j++){
if(strcmp(books[j].author, books[j + 1].author) > 0){
book tmp = books[j];
books[j] = books[j + 1];
books[j + 1] = tmp;
}
}
}
cout << "按作者排序成功!" << endl;
system("pause");
break;
case 4:
for(int i = 0; i < n - 1; i++){
for(int j = 0; j < n - i - 1; j++){
if(strcmp(books[j].publisher, books[j + 1].publisher) > 0){
book tmp = books[j];
books[j] = books[j + 1];
books[j + 1] = tmp;
}
}
}
cout << "按出版社排序成功!" << endl;
system("pause");
break;
case 5:
for(int i = 0; i < n - 1; i++){
for(int j = 0; j < n - i - 1; j++){
if(strcmp(books[j].date, books[j + 1].date) > 0){
book tmp = books[j];
books[j] = books[j + 1];
books[j + 1] = tmp;
}
}
}
cout << "按出版日期排序成功!" << endl;
system("pause");
break;
default:
cout << "输入有误,请重新输入!" << endl;
system("pause");
}
}
void save(){
ofstream fout("books.txt");
for(int i = 0; i < n; i++){
fout << books[i].id << " " << books[i].name << " " << books[i].author << " " << books[i].publisher << " " << books[i].date << " " << books[i].quantity << " " << books[i].price << endl;
}
fout.close();
}
void load(){
ifstream fin("books.txt");
while(fin >> books[n].id >> books[n].name >> books[n].author >> books[n].publisher >> books[n].date >> books[n].quantity >> books[n].price){
n++;
}
fin.close();
}
```
该程序使用结构体 `book` 存储图书信息,包括编号、名称、作者、出版社、出版日期、数量和价格。程序提供了以下功能:
1. 显示图书
2. 添加图书
3. 查找图书
4. 删除图书
5. 修改图书
6. 排序图书
图书信息保存在文件 `books.txt` 中,程序启动时会从文件中读取数据。程序结束时,会将数据保存到文件中。
相关推荐
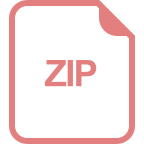
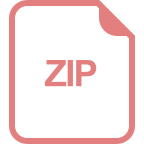














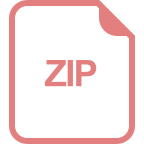