HttpURLConnection disconnect方法
时间: 2023-08-22 12:05:51 浏览: 55
`disconnect()` 方法是 `HttpURLConnection` 类的一个实例方法,用于断开与远程服务器的连接。当你完成了 `HttpURLConnection` 对象的操作,或者你不再需要该连接时,应该显式地调用 `disconnect()` 方法来释放底层的网络连接和资源。
在调用 `disconnect()` 方法之后,`HttpURLConnection` 对象将不再可用,并且任何尝试对该对象进行操作的方法调用都将抛出 `IllegalStateException` 异常。
注意,在 `HttpURLConnection` 对象上调用 `disconnect()` 方法并不会立即关闭底层的网络连接。相反,该方法会释放连接持有的所有资源,并将该连接放回连接池中以供重用。如果底层的连接已经被关闭,或者连接池已满,那么该方法将不起作用。
以下是 `disconnect()` 方法的语法:
```java
public void disconnect()
```
该方法没有返回值。如果调用 `disconnect()` 方法时出现任何异常,例如 `IOException`,则应该将其捕获并处理。
相关问题
HttpURLConnection get方法调用接口
您可以使用以下代码来使用Java中的HttpURLConnection类进行GET方法调用接口:
```
URL url = new URL("http://example.com/api/data");
HttpURLConnection con = (HttpURLConnection) url.openConnection();
con.setRequestMethod("GET");
int status = con.getResponseCode();
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer content = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
content.append(inputLine);
}
in.close();
con.disconnect();
System.out.println(content.toString());
```
其中,您需要将URL替换为您要访问的接口地址,然后使用`setRequestMethod("GET")`方法指定使用GET方法进行请求。最后,使用`getResponseCode()`方法获取状态码,`getInputStream()`方法获取响应结果。
HttpUrlConnection
HttpUrlConnection 是 Java 标准库中用于发送 HTTP 请求和处理 HTTP 响应的类。它提供了一种简单的方式来与远程服务器进行通信。您可以使用 HttpUrlConnection 类来建立连接、发送请求、读取响应和处理错误。
以下是使用 HttpUrlConnection 发送 GET 请求的示例代码:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpUrlConnectionExample {
public static void main(String[] args) {
try {
URL url = new URL("http://example.com/api");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
int responseCode = connection.getResponseCode();
System.out.println("Response Code: " + responseCode);
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String line;
StringBuilder response = new StringBuilder();
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
System.out.println("Response: " + response.toString());
connection.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
这个示例中,我们创建了一个 URL 对象来指定要发送请求的目标 URL。然后,我们打开一个 HttpURLConnection 连接并设置请求方法为 GET。发送请求后,我们可以获取响应码、读取响应内容,并在最后关闭连接。
您可以根据需要设置请求头、添加请求参数等。同时,HttpUrlConnection 也支持其他的 HTTP 方法,如 POST、PUT、DELETE 等。
相关推荐
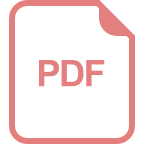
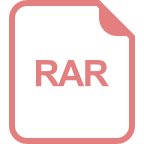












