使用Vue3.0完成一个todolist
时间: 2023-07-20 18:45:15 浏览: 75
好的,下面是一个简单的 Vue3.0 Todolist 示例代码:
```html
<template>
<div>
<h1>Todolist</h1>
<form @submit.prevent="addTodo">
<input type="text" v-model="newTodo" placeholder="Add a new todo">
<button>Add</button>
</form>
<ul>
<li v-for="(todo, index) in todos" :key="index">
<input type="checkbox" v-model="todo.completed" @change="toggleTodoStatus(index)">
<span :class="{completed: todo.completed}">{{ todo.text }}</span>
<button @click="removeTodo(index)">X</button>
</li>
</ul>
</div>
</template>
<script>
import { reactive } from 'vue';
export default {
setup() {
const state = reactive({
newTodo: '',
todos: [],
});
const addTodo = () => {
if (state.newTodo.trim() !== '') {
state.todos.push({ text: state.newTodo, completed: false });
state.newTodo = '';
}
};
const toggleTodoStatus = (index) => {
state.todos[index].completed = !state.todos[index].completed;
};
const removeTodo = (index) => {
state.todos.splice(index, 1);
};
return {
newTodo: state.newTodo,
todos: state.todos,
addTodo,
toggleTodoStatus,
removeTodo,
};
},
};
</script>
<style>
.completed {
text-decoration: line-through;
}
</style>
```
这是一个基本的 Todolist 组件,使用了 Vue3.0 的 Composition API 和响应式数据。在模板中,使用了 v-model 指令来双向绑定输入框和数据,使用 v-for 指令来循环渲染列表项。在 setup 函数中,使用了 reactive 函数来创建响应式数据对象,使用了箭头函数来定义组件的方法。在样式中,使用了伪类选择器 :class 来动态添加类名,实现对已完成的任务的样式修改。
相关推荐
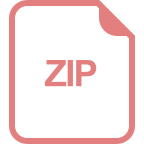














