java:GUI实现通讯录增删改查源码
时间: 2023-08-29 08:08:21 浏览: 96
这是一个简单的Java GUI通讯录增删改查的示例代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
public class ContactListGUI extends JFrame {
private JLabel nameLabel, phoneLabel, emailLabel;
private JTextField nameField, phoneField, emailField;
private JButton addButton, deleteButton, editButton, searchButton;
private JList<Contact> contactList;
private DefaultListModel<Contact> model;
private ArrayList<Contact> contacts;
public ContactListGUI() {
super("Contact List");
nameLabel = new JLabel("Name:");
phoneLabel = new JLabel("Phone:");
emailLabel = new JLabel("Email:");
nameField = new JTextField(20);
phoneField = new JTextField(20);
emailField = new JTextField(20);
addButton = new JButton("Add");
addButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
addContact();
}
});
deleteButton = new JButton("Delete");
deleteButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
deleteContact();
}
});
editButton = new JButton("Edit");
editButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
editContact();
}
});
searchButton = new JButton("Search");
searchButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
searchContacts();
}
});
model = new DefaultListModel<>();
contactList = new JList<>(model);
contactList.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
JPanel inputPanel = new JPanel(new GridLayout(3, 2));
inputPanel.add(nameLabel);
inputPanel.add(nameField);
inputPanel.add(phoneLabel);
inputPanel.add(phoneField);
inputPanel.add(emailLabel);
inputPanel.add(emailField);
JPanel buttonPanel = new JPanel(new GridLayout(4, 1));
buttonPanel.add(addButton);
buttonPanel.add(deleteButton);
buttonPanel.add(editButton);
buttonPanel.add(searchButton);
JPanel listPanel = new JPanel(new BorderLayout());
listPanel.add(new JScrollPane(contactList), BorderLayout.CENTER);
JPanel mainPanel = new JPanel(new BorderLayout());
mainPanel.add(inputPanel, BorderLayout.NORTH);
mainPanel.add(buttonPanel, BorderLayout.EAST);
mainPanel.add(listPanel, BorderLayout.CENTER);
getContentPane().add(mainPanel);
setSize(500, 400);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
contacts = new ArrayList<>();
}
private void addContact() {
String name = nameField.getText();
String phone = phoneField.getText();
String email = emailField.getText();
if (name.isEmpty() || phone.isEmpty() || email.isEmpty()) {
JOptionPane.showMessageDialog(this, "Please enter all fields.");
return;
}
Contact contact = new Contact(name, phone, email);
contacts.add(contact);
model.addElement(contact);
nameField.setText("");
phoneField.setText("");
emailField.setText("");
}
private void deleteContact() {
int index = contactList.getSelectedIndex();
if (index == -1) {
JOptionPane.showMessageDialog(this, "Please select a contact.");
return;
}
Contact contact = model.getElementAt(index);
contacts.remove(contact);
model.removeElementAt(index);
}
private void editContact() {
int index = contactList.getSelectedIndex();
if (index == -1) {
JOptionPane.showMessageDialog(this, "Please select a contact.");
return;
}
Contact contact = model.getElementAt(index);
String name = nameField.getText();
String phone = phoneField.getText();
String email = emailField.getText();
if (!name.isEmpty()) {
contact.setName(name);
}
if (!phone.isEmpty()) {
contact.setPhone(phone);
}
if (!email.isEmpty()) {
contact.setEmail(email);
}
model.setElementAt(contact, index);
nameField.setText("");
phoneField.setText("");
emailField.setText("");
}
private void searchContacts() {
String query = JOptionPane.showInputDialog(this, "Search contacts:");
if (query == null || query.isEmpty()) {
return;
}
model.removeAllElements();
for (Contact contact : contacts) {
if (contact.getName().contains(query) || contact.getPhone().contains(query) || contact.getEmail().contains(query)) {
model.addElement(contact);
}
}
}
public static void main(String[] args) {
ContactListGUI gui = new ContactListGUI();
gui.setVisible(true);
}
}
class Contact {
private String name;
private String phone;
private String email;
public Contact(String name, String phone, String email) {
this.name = name;
this.phone = phone;
this.email = email;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
@Override
public String toString() {
return name + " (" + phone + ", " + email + ")";
}
}
```
这个程序使用了Swing GUI库来创建界面和组件。它使用了一个JList来显示通讯录联系人列表,并提供了添加、删除、编辑和搜索联系人的功能。通讯录联系人数据存储在一个ArrayList中,并使用一个自定义的Contact类来表示联系人。
阅读全文
相关推荐








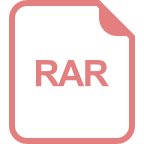







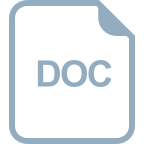

